Common Mistakes in Selenium WebDriver Automation Testing
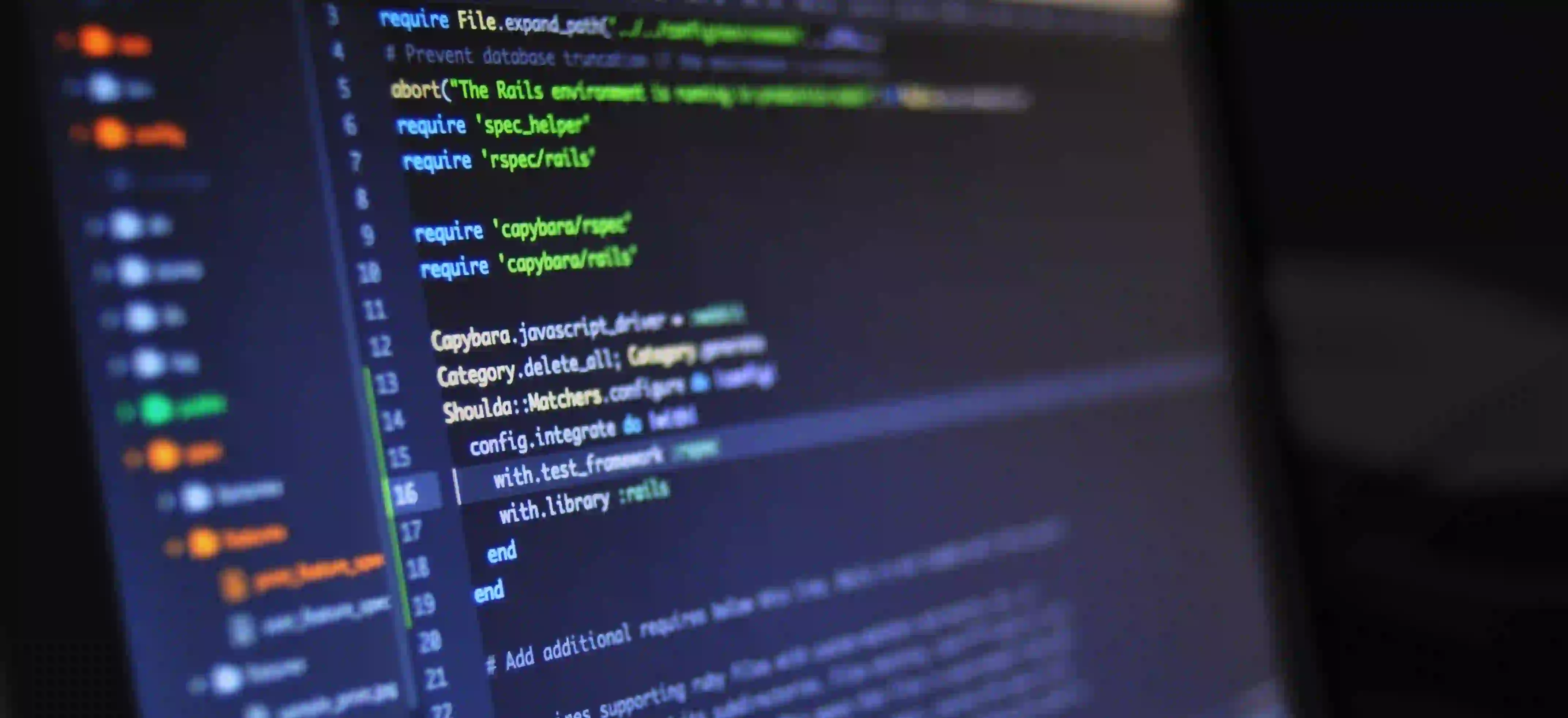
Common Mistakes in Selenium WebDriver Automation Testing
Automation testing is an essential part of software development and quality assurance, particularly when it comes to web applications. Selenium WebDriver, one of the most popular frameworks for this type of testing, allows testers to simulate user interactions in browsers effectively. However, even seasoned testers make mistakes that can lead to ineffective tests, wasted resources, and even project delays.
In this blog post, we will explore some common pitfalls when using Selenium WebDriver for automation testing. By understanding these mistakes, you can improve your automation scripts and ultimately deliver higher quality software.
1. Not Choosing the Right Locator Strategy
The Importance of Locators
Locators are fundamental in Selenium as they help identify and interact with web elements. A common mistake many testers make is not choosing the right locator strategy. For example, relying solely on XPath can slow down your tests.
Example Code Snippet
// Inefficient use of XPath
WebElement element = driver.findElement(By.xpath("//div[@id='example']//span[text()='Sample Text']"));
The Why
Using complex XPath locators can lead to fragile tests. If the structure of the page changes, tests break easily. Instead, prefer simpler and more stable locators like ID or CSS Selectors whenever possible:
// Using ID for better performance and stability
WebElement element = driver.findElement(By.id("exampleId"));
2. Ignoring Synchronization Issues
The Problem with Speed
Automation scripts often run faster than a web page can load. Testers frequently overlook synchronization issues, leading to "No Such Element" exceptions or incorrect element interactions.
Example Code Snippet
// Incorrectly assuming an element is ready
driver.findElement(By.id("submitButton")).click();
The Why
Waiting for the element to be interactable improves script reliability. Implementing explicit waits can solve this problem:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("submitButton")));
element.click();
3. Overusing Implicit Waits
Implicit versus Explicit Waits
Implicit waits automatically wait for a certain amount of time before throwing an exception if the element is not present. Many testers misuse them, causing unexpected delays in test execution.
Example Code Snippet
// Global implicit wait
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
The Why
Overusing implicit waits can lead to longer test times as they apply to all elements. It's more efficient to use explicit waits for specific elements that need longer loading times. This keeps the tests swift and enhances readability.
4. Hardcoding Values
Flexibility Matters
Many automation testers hardcode values within scripts, making them less flexible and harder to maintain. This can lead to frequent modifications in multiple places when a change occurs.
Example Code Snippet
// Hardcoded values
driver.findElement(By.name("username")).sendKeys("testUser");
driver.findElement(By.name("password")).sendKeys("testPassword");
The Why
Instead, parameterize the values or use external data sources:
String username = TestData.getUsername(); // Assume TestData is a class that fetches data
String password = TestData.getPassword();
driver.findElement(By.name("username")).sendKeys(username);
driver.findElement(By.name("password")).sendKeys(password);
This approach enhances flexibility and maintainability, allowing changes in one place.
5. Lack of Proper Exception Handling
Anticipate and React
Selenium tests can encounter unexpected scenarios. Not handling exceptions properly can lead to false results or hanging tests that waste valuable time.
Example Code Snippet
// No exception handling
WebElement element = driver.findElement(By.id("unknownId"));
element.click();
The Why
Wrap your actions inside try-catch blocks to gracefully handle such scenarios:
try {
WebElement element = driver.findElement(By.id("unknownId"));
element.click();
} catch (NoSuchElementException e) {
System.out.println("Element not found: " + e.getMessage());
}
This way, your script continues running even when an element isn’t found, allowing for better logging and understanding of failures.
6. Not Employing Page Object Model (POM)
Improve Structure and Readability
The Page Object Model is a design pattern that enhances code maintainability and reusability. Some testers ignore this pattern and create linear scripts instead.
The Why
Without POM, your codebase becomes a tangled web of script lines, reducing readability and making updates difficult. Here’s how POM improves your setup:
Page Class Example
public class LoginPage {
WebDriver driver;
By usernameField = By.name("username");
By passwordField = By.name("password");
By loginButton = By.id("submit");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void login(String username, String password) {
driver.findElement(usernameField).sendKeys(username);
driver.findElement(passwordField).sendKeys(password);
driver.findElement(loginButton).click();
}
}
Usage in Test Class
LoginPage loginPage = new LoginPage(driver);
loginPage.login("testUser", "testPassword");
By separating concerns, you facilitate easier code updates and testing.
7. Lack of Test Reporting
Communicating Results
Failing to implement test reporting can leave teams in the dark regarding what tests passed or failed. This lack can cause delays in identifying issues.
The Why
Using reporting frameworks like TestNG or ExtentReports provides visibility into test execution and performance results.
Example Code Snippet for TestNG
import org.testng.Assert;
import org.testng.annotations.Test;
public class LoginTest {
@Test
public void testLogin() {
LoginPage loginPage = new LoginPage(driver);
loginPage.login("testUser", "testPassword");
Assert.assertTrue(driver.getTitle().contains("Dashboard"));
}
}
This not only checks conditions but also generates reports for better teamwork and faster resolutions.
Lessons Learned
Automation testing can drive faster development cycles and improve software quality, but only if executed correctly. By recognizing and addressing these common mistakes with Selenium WebDriver, you can create more reliable, efficient, and maintainable automation scripts.
As you strengthen your knowledge, consider exploring additional resources, such as the official Selenium documentation and quality assurance best practices. Remember, the road to effective automation testing is continuous learning and adaptation.
Your automation journey starts with awareness. Avoiding these common pitfalls allows you to leverage the full capabilities of Selenium WebDriver and build a robust testing process that stands the test of time.
Additional Resources
Happy Testing!