Overcoming Java EE 6 Deployment Challenges on the Cloud
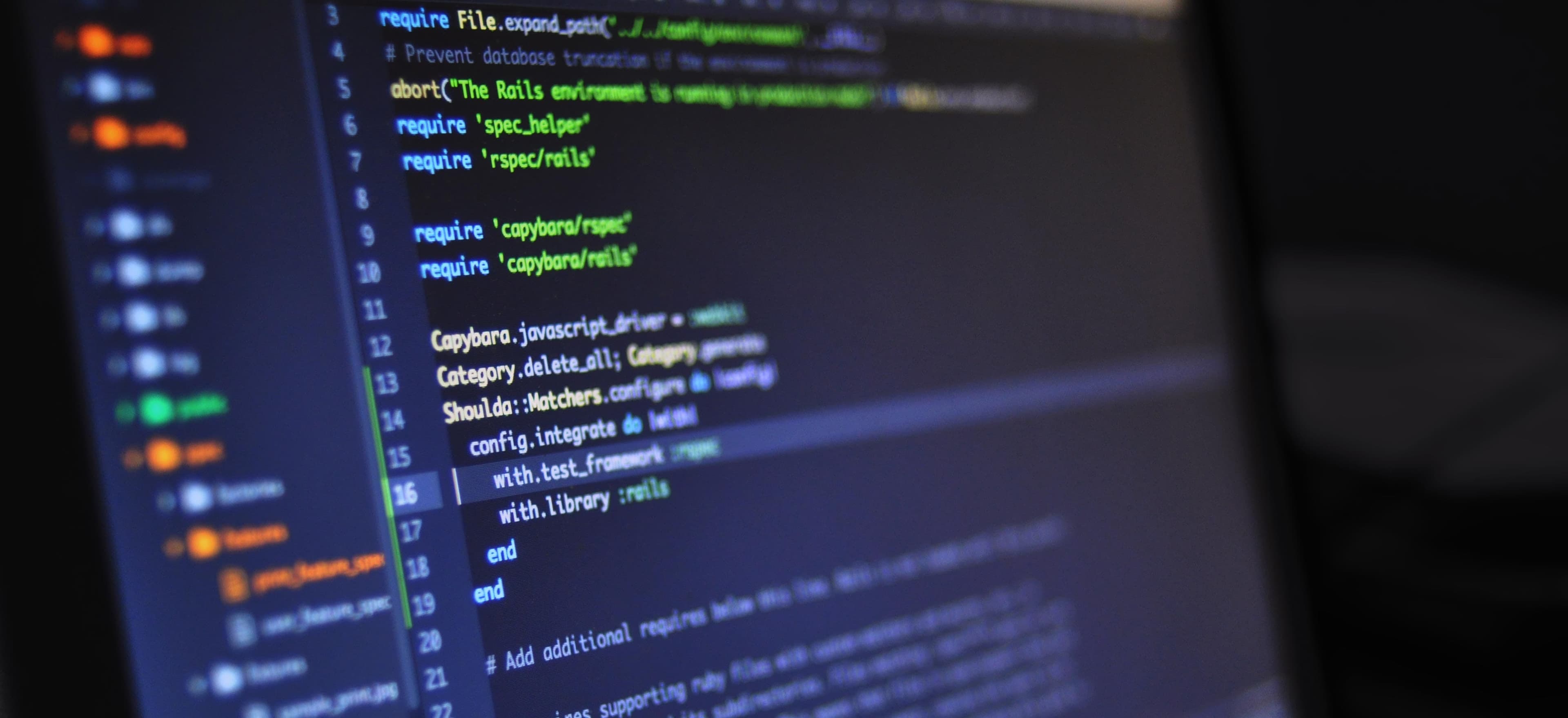
- Published on
Overcoming Java EE 6 Deployment Challenges on the Cloud
Java EE (Enterprise Edition) has been a central player in enterprise-level applications development for years. Even though Java EE 6 was released over a decade ago, many enterprise systems still leverage its capabilities. One challenge that developers often face is deploying Java EE 6 applications to the cloud. This blog post addresses common deployment challenges and strategies to overcome them.
Understanding Java EE 6 Architecture
Before diving into deployment, it’s crucial to understand the architectural components of Java EE 6. The key components of Java EE 6 include:
- EJB (Enterprise JavaBeans): Manages business logic, enabling scalability and transaction management.
- JPA (Java Persistence API): Handles data persistence, making it easier to manage database operations.
- Servlets and JSP (JavaServer Pages): Facilitate the creation of web applications.
- CDI (Contexts and Dependency Injection): Improves decoupling and makes dependency management more intuitive.
These components collectively enhance the development of robust, maintainable, and scalable applications.
Common Deployment Challenges
1. Configuration Issues
When deploying Java EE applications to the cloud, misconfiguration can lead to runtime failures. Ensuring that the application server configurations align with cloud environment settings can be daunting.
2. Database Connectivity
Many businesses utilize CNC (Cloud-Native Databases). The challenge here is establishing reliable connectivity, especially due to networking and security settings in the cloud.
3. State Management
In a cloud environment, maintaining the state of applications can pose difficulties, particularly in handling sessions over multiple instances of application servers.
4. Legacy Dependencies
Java EE 6 applications often rely on libraries and frameworks that may not be optimized for cloud environments. Transitioning these dependencies can require significant code rewrites.
Deployment Strategies
Here are some effective strategies for overcoming the deployment challenges mentioned above:
1. Utilize a Modern Application Server
Selecting a modern application server like WildFly or Payara can ease many deployment challenges. These servers have excellent support for the Java EE ecosystem and cloud deployment.
Here's an example of how you might configure WildFly using a simple standalone.xml
file:
<subsystem xmlns="urn:jboss:domain:datasources:2.0">
<datasource jndi-name="java:jboss/datasources/MyDS" pool-name="MyDS" enabled="true" use-java-context="true">
<connection-url>jdbc:mysql://hostname:3306/dbname</connection-url>
<driver>mysql</driver>
<security>
<user-name>username</user-name>
<password>password</password>
</security>
</datasource>
</subsystem>
Why This Matters: This configuration directly sets up your data source with the necessary parameters to connect to your cloud-native database effectively. Misconfiguration here could lead to connection failures.
2. Implement Dependency Injection
Using dependency injection via CDI can streamline the application's setup. In a cloud environment, the goal is to minimize the coupling among components.
For example, consider this simple implementation:
import javax.enterprise.context.RequestScoped;
import javax.inject.Inject;
@RequestScoped
public class MyService {
@Inject
private DatabaseConnector dbConnector;
public void performAction() {
dbConnector.connect();
// perform action with the database
}
}
Why This Matters: This approach allows better management of services, promoting a decoupled architecture that is easier to maintain and adapt to cloud environments.
3. Use Cloud-Based Database Solutions
Utilizing cloud-native databases like Amazon RDS, Google Cloud SQL, or Azure SQL Database can enhance performance and reliability. Ensure that your application is compatible with the chosen database technology.
4. Embrace Microservices
Transitioning from a monolithic architecture to microservices is beneficial for cloud deployment. Microservices allow you to deploy independent components, improving scalability and resilience.
Consider this refactored service:
@ApplicationScoped
@Path("/account")
public class AccountService {
@Inject
private AccountRepository repository;
@GET
@Path("/{id}")
public Response getAccount(@PathParam("id") String id) {
Account account = repository.findById(id);
return account != null ? Response.ok(account).build() : Response.status(Response.Status.NOT_FOUND).build();
}
}
Why This Matters: This RESTful service allows for independent deployment and scaling of accounts. It illustrates a clean separation of concerns, which is ideal in cloud environments.
5. Monitor and Implement Logging
To ensure application health, robust monitoring and logging systems must be in place. Utilize tools like ELK Stack (Elasticsearch, Logstash, and Kibana) or cloud-native logging solutions.
Here's an example of basic logging in a Java EE application:
import java.util.logging.Logger;
import javax.ejb.Stateless;
@Stateless
public class MyBusinessLogic {
private static final Logger logger = Logger.getLogger(MyBusinessLogic.class.getName());
public void executeBusinessLogic() {
logger.info("Executing business logic...");
// actual logic
}
}
Why This Matters: Implementing logging helps capture issues proactively, making your cloud application more resilient and performant.
Final Thoughts
Deploying Java EE 6 applications in the cloud does present unique challenges, but with the right strategies, they can be effectively addressed. From employing modern application servers to adapting a microservices architecture, each step takes your application closer to a successful cloud deployment.
For more information on Java EE and its deployment on cloud platforms, check out Oracle's Java EE documentation and the IBM Cloud Post on Java EE deployment strategies.
By following these insights and examples, you can overcome Java EE 6 deployment challenges and leverage the power of cloud computing. Happy coding!
Checkout our other articles