Common OAuth Pitfalls in Spring Security: How to Avoid Them
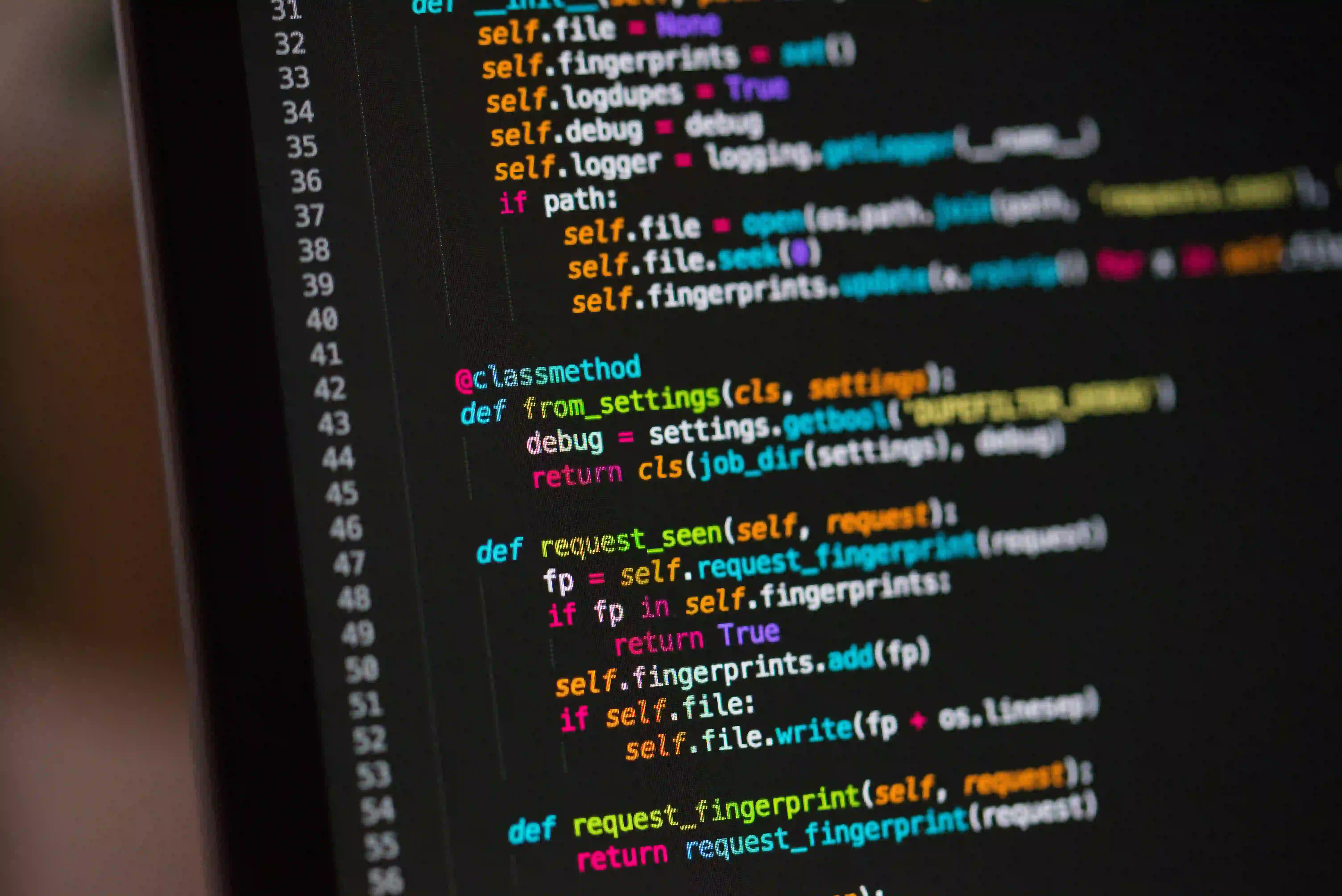
Common OAuth Pitfalls in Spring Security: How to Avoid Them
When building modern applications, authorization is a key factor that cannot be overlooked. OAuth 2.0 has emerged as a leading authorization framework, particularly for APIs and web applications. With Spring Security simplifying the integration of OAuth in Java applications, developers often find themselves wrestling with various pitfalls. In this post, we will explore common OAuth errors encountered in Spring Security and provide solutions to avoid them.
Understanding OAuth 2.0
Before diving into the pitfalls, it is crucial to understand OAuth 2.0. This protocol enables applications to obtain limited access to user accounts on an HTTP service. It does this by delegating user authentication to a service that hosts the user account and authorizing third-party applications without sharing passwords.
Key Components of OAuth 2.0:
- Resource Owner: The user who grants access to their resources.
- Client: The application wishing to access the resource owner's data.
- Authorization Server: The server that authenticates the resource owner and issues access tokens.
- Resource Server: The server that holds the users' protected resources.
Common Pitfalls in Spring Security OAuth
1. Failing to Secure Sensitive Endpoints
One of the foremost mistakes developers make is neglecting to secure sensitive endpoints. Often, developers assume that because they implemented OAuth, their endpoints are safe. This is far from the truth.
Solution: Implement proper role-based access control.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/admin/**").hasRole("ADMIN")
.antMatchers("/api/user/**").authenticated()
.anyRequest().permitAll()
.and()
.oauth2Login();
}
}
This configuration ensures that only users with the "ADMIN" role can access /api/admin/**
endpoints, reinforcing security.
2. Hardcoding Client Credentials
A prevalent issue developers face is hardcoding client ID and secret directly into the application. This not only poses a security risk but also hinders maintainability.
Solution: Use environment variables or application properties files.
For instance, define your credentials in application.yml
:
security:
oauth2:
client:
registration:
my-client:
client-id: ${CLIENT_ID}
client-secret: ${CLIENT_SECRET}
# Set environment variables
export CLIENT_ID=my-client-id
export CLIENT_SECRET=my-client-secret
This approach enhances security and makes it easier to manage configurations across different environments.
3. Ignoring Token Expiration and Refresh Mechanisms
Another significant oversight is disregarding token management. Access tokens typically expire, and failing to refresh them can lead to unauthorized access errors.
Solution: Implement token refresh logic.
Spring Security provides mechanisms to handle token expiration. Use refresh tokens and the following setup to manage access:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.oauth2ResourceServer()
.jwt()
.and()
.authorizeRequests()
.anyRequest().authenticated();
}
}
Ensure your client requests a refresh token when initially obtaining access tokens. This mechanism allows seamless user experiences without requiring users to re-authenticate frequently.
4. Mismanaging Scopes
Scopes determine the level of access granted to users and resources. Misconfiguring scopes can either expose too much data or result in applications being overly restrictive.
Solution: Clearly define and document your scopes.
security:
oauth2:
client:
registration:
my-client:
scope:
- read
- write
By explicitly stating the scopes your application requires, you can avoid both overexposure and unnecessary blocks of legitimate requests.
5. Lack of Logging and Monitoring
Failing to implement logging can leave your application vulnerable and difficult to debug. Without adequate monitoring, malicious attempts at access can go unnoticed.
Solution: Implement comprehensive logging.
@Component
public class CustomOAuth2AuthenticationSuccessHandler
implements AuthenticationSuccessHandler {
@Override
public void onAuthenticationSuccess(HttpServletRequest request,
HttpServletResponse response,
Authentication authentication)
throws IOException, ServletException {
// Log the authentication success
logger.info("User {} authenticated successfully",
authentication.getName());
response.sendRedirect("/home");
}
}
This logging mechanism allows you to trace successful authentications easily. Pair this with monitoring tools like Spring Actuator and ELK Stack (Elasticsearch, Logstash, Kibana) for effective insights into user behavior and security audits.
6. Not Validating Redirect URIs
Finally, failing to validate redirect URIs can allow for malicious redirects, leading to security vulnerabilities, such as Open Redirect attacks.
Solution: Define valid redirect URIs in your OAuth configuration.
# application.yml
security:
oauth2:
client:
registration:
my-client:
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
redirect-uri-template: "{baseUrl}/login/oauth2/code/{registrationId}"
By setting valid redirect URIs, you can mitigate the risk of unauthorized redirection during the OAuth flow.
Key Takeaways
Integrating OAuth 2.0 in Spring Security provides a robust framework for secure user authentication. However, developers must remain vigilant to avoid common pitfalls. By adhering to best practices—such as not hardcoding credentials, carefully managing token lifecycles, and implementing proper logging and monitoring—you can significantly enhance the security of your applications.
If you wish to dive deeper into OAuth 2.0 and Spring Security, the official Spring Security documentation provides detailed guidelines and examples.
Happy coding, and may your applications remain secure!