Troubleshooting Spring Bean Encapsulation Issues
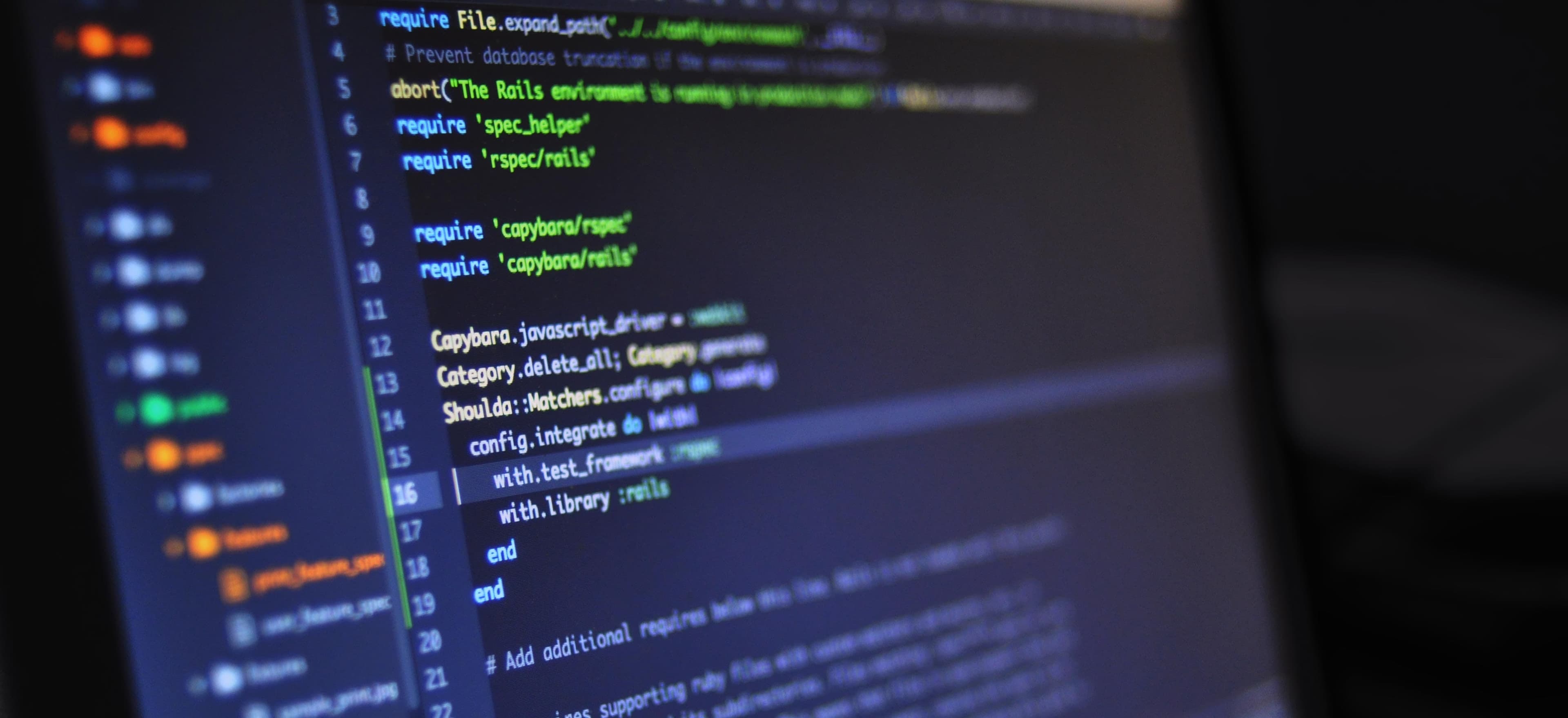
- Published on
Troubleshooting Spring Bean Encapsulation Issues
In the world of Java development, Spring Framework stands out as a powerful tool for building enterprise applications. One of its greatest features is dependency injection, which facilitates the separation of concerns and enhances testability. However, developers sometimes encounter encapsulation issues with Spring Beans. In this blog post, we will explore common encapsulation challenges, how to troubleshoot them effectively, and best practices to avoid these pitfalls in the future.
Understanding Spring Beans
Before delving into troubleshooting, let’s clarify what Spring Beans are. In simpler terms, Spring Beans are objects managed by the Spring IoC (Inversion of Control) container. They are instantiated, assembled, and otherwise managed by the Spring framework. The encapsulation principle, which refers to restricting access to the inner workings of a class, is crucial to ensuring that beans operate as intended without unintended side-effects.
Key Characteristics of Spring Beans:
- Lifecycle Management: Spring manages the entire lifecycle of the beans.
- Configuration and Wiring: Utilizing XML, annotations, or Java configuration.
- Scope: Beans can have various scopes like singleton, prototype, request, session, etc.
Common Encapsulation Issues
1. Access Modifiers
A frequent source of encapsulation issues arises from mistakenly exposing class properties. In Java, class attributes should be private to ensure controlled access. When a Spring Bean property is set to public or package-private, it can be directly manipulated outside the class, leading to inconsistent states.
Example Code:
public class UserService {
// This exposes the bean property, which is not recommended
public String userName;
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
}
Why This is a Problem:
Exposing properties directly like this goes against the encapsulation principle. Other classes could change userName
without using the designated setter, leading to unpredictable behavior within UserService
.
Solution:
Always keep the properties private and provide proper getters and setters for access.
public class UserService {
private String userName; // private access modifier
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
// Add validation if needed
this.userName = userName;
}
}
2. Dependency Exposure
When using Spring’s dependency injection, a common mistake is publicly exposing dependencies that should remain internal. This not only breaks encapsulation but also makes unit testing more challenging.
Example Code:
public class NotificationService {
public EmailService emailService; // Public exposure of dependency
public void sendEmail(String message) {
emailService.send(message);
}
}
Why This is a Problem:
By exposing emailService
, the integrity of NotificationService
is compromised. Other components can modify or replace emailService
, leading to side effects.
Solution:
Utilize constructor or setter injection, keeping dependencies private.
public class NotificationService {
private final EmailService emailService; // private and final to enforce immutability
public NotificationService(EmailService emailService) {
this.emailService = emailService;
}
public void sendEmail(String message) {
emailService.send(message);
}
}
Diagnosing Encapsulation Issues
1. Debugging Beans
When problems arise, it is crucial to inspect your beans. You can enable debug logging in your application.properties
file:
logging.level.org.springframework=DEBUG
This allows you to see the lifecycle events of your beans, which can help diagnose any issues with their states during initialization.
2. Bean Scenarios
Understand the scenarios in which your beans are created and utilized. For example, using singleton scopes for beans that manage state can cause unexpected behaviors. It’s vital to pick the right scope for your beans.
Example of Scope Misunderstanding:
If you define a stateful bean as a singleton but expect it to behave like a prototype, you’ll face issues.
@Component
@Scope("singleton")
public class SessionData {
private int userId; // stateful variable
public void setUserId(int userId) {
this.userId = userId;
}
}
In this case, all users will share this SessionData
bean. Instead, define it as a prototype for each user session:
@Component
@Scope("prototype")
public class SessionData {
private int userId;
public void setUserId(int userId) {
this.userId = userId;
}
}
Best Practices for Encapsulation
-
Use access modifiers wisely: Always prefer private attributes with public methods for controlled access.
-
Favor Constructor Injection: This method promotes immutability and ensures that your bean is always in a valid state.
-
Avoid exposing the implementation details: Think of your beans as a black box; expose only what is necessary.
-
Unit Test Your Beans: Write test cases for your beans to ensure they are working as intended without unintended side effects. Use frameworks like JUnit and Mockito for testing Spring beans effectively. Here’s a guide on unit testing Spring Boot applications.
-
Document your code: Clear documentation can help other developers understand how to interact with your beans without breaking encapsulation.
To Wrap Things Up
In Java development, especially when using the Spring Framework, encapsulation should never be an afterthought. Proper access modifiers, dependency management, and a thoughtful approach to bean scopes are essential for maintaining the integrity of your application. Just remember, when encapsulation issues arise, take a step back, debug effectively, and adhere to best practices to ensure robust, maintainable code.
By following the steps outlined in this blog post, you will be well on your way to troubleshooting Spring Bean encapsulation issues effectively. For further reading on Spring Beans, check out the official Spring documentation.
Whether you are a novice or an experienced developer, understanding and applying these principles will dramatically improve the ease with which you can maintain and extend your Java applications. The power of Spring is in its flexibility, so embrace it and let your applications shine!
Checkout our other articles