Mastering IntStream: Convert to Array in Java 8 with Ease
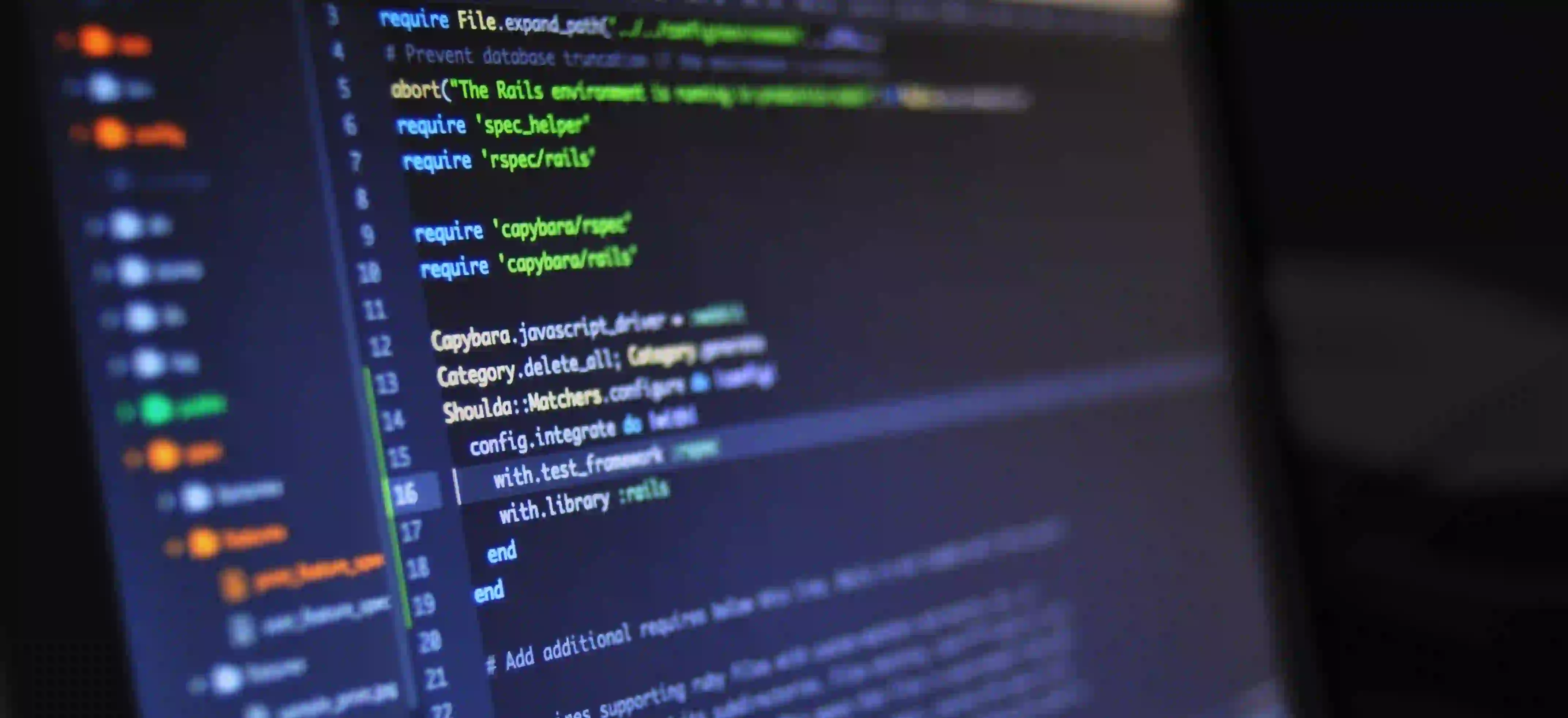
Mastering IntStream: Convert to Array in Java 8 with Ease
Java 8 introduced a plethora of features aimed at simplifying data manipulation and enhancing developer productivity. One of the standout features is the IntStream
class, which is part of the java.util.stream package. Among its many capabilities, converting an IntStream
to an array is a fundamental operation that every Java developer should master. In this blog post, we will delve deep into the workings of IntStream
, illustrating how to efficiently convert it to an array. Along the way, I'll provide illustrative code snippets, explanations of why each part is important, and useful tips.
What is IntStream?
IntStream
is a sequence of primitive int-valued elements supporting sequential and parallel aggregate operations. By using streams, Java developers can harness the power of functional programming, making their code more readable and concise.
Why Use IntStream?
- Performance: Operations on
IntStream
are generally faster because they operate on raw integers instead of Integer objects. - Conciseness: Functional-style programming leads to less boilerplate code.
- Lazy Evaluation: Streams are evaluated on demand, which can lead to performance optimizations.
Creating an IntStream
Before we can convert an IntStream
to an array, we first need to create one. Here are some ways to create an IntStream
:
-
From an Array:
☕snippet.javaint[] numbers = {1, 2, 3, 4, 5}; IntStream intStreamFromArray = Arrays.stream(numbers);
-
From Range:
☕snippet.javaIntStream intStreamFromRange = IntStream.range(1, 6); // 1 to 5
-
From Random Numbers:
☕snippet.javaIntStream randomIntStream = new Random().ints(5, 1, 101); // 5 random numbers between 1 and 100
These examples demonstrate the simplicity and flexibility of creating an IntStream
. Now that we have our IntStream
, let's move on to converting it to an array.
Converting IntStream to Array
To convert an IntStream
to an array of integers, we can use the toArray()
method provided by the IntStream
class. This method is straightforward and efficient.
Example of Conversion
Here’s a concise example demonstrating the conversion of an IntStream
to an array:
import java.util.stream.IntStream;
public class IntStreamExample {
public static void main(String[] args) {
IntStream intStream = IntStream.range(1, 6); // Creating IntStream from range 1 to 5
int[] resultArray = intStream.toArray(); // Converting IntStream to array
// Display the array content
System.out.println(Arrays.toString(resultArray)); // Output: [1, 2, 3, 4, 5]
}
}
Why toArray()?
- Simplicity: The
toArray()
method abstracts away the complexities that come with traditional array creation, saving time and effort. - Efficiency: Internally, it streamlines the conversion process, leveraging optimizations for primitive types.
Filter and Convert IntStream to Array
One of the powerful features of IntStream
is the ability to filter elements before conversion. This ensures that only relevant data is stored in the resulting array.
Example: Filtering Even Numbers
Let's say we want to extract only the even numbers from a range of integers and convert those into an array:
import java.util.Arrays;
import java.util.stream.IntStream;
public class FilteredIntStream {
public static void main(String[] args) {
IntStream intStream = IntStream.range(1, 11); // Range from 1 to 10
int[] evenNumbersArray = intStream
.filter(num -> num % 2 == 0) // Filtering even numbers
.toArray(); // Converting to array
// Display the even numbers array
System.out.println(Arrays.toString(evenNumbersArray)); // Output: [2, 4, 6, 8, 10]
}
}
Why Filter Before Conversion?
Filtering before conversion allows for targeted data extraction. By employing such filters:
- Reduced Memory Usage: Only relevant data is stored, minimizing memory footprint.
- Clean Data: You end up with a dataset that is already curated as per your requirements.
Using IntStream with Custom Logic
IntStream
is not just about simple operations; you can introduce custom logic to enrich your data processing routines.
Example: Custom Operation with Reduce
Suppose we want to compute the sum of squares of a range of integers and want to store the final result in an array:
import java.util.Arrays;
import java.util.stream.IntStream;
public class CustomIntStream {
public static void main(String[] args) {
int[] squares = IntStream.range(1, 6) // Range from 1 to 5
.map(x -> x * x) // Squaring each element
.toArray(); // Convert to array
// Display the squares array
System.out.println(Arrays.toString(squares)); // Output: [1, 4, 9, 16, 25]
}
}
Why Use Custom Logic?
Adding custom operations lets you mold streaming data to fit your needs. In this example:
- Flexibility: You define how each element is transformed.
- Comprehensibility: The expression reads clearly, making the intent immediately obvious.
Real-world Scenarios for IntStream Transformation
Understanding how to use IntStream
effectively is crucial when dealing with real-world scenarios:
- Financial Applications: Analyzing series of financial transactions, applying filters for profitable vs. non-profitable transactions.
- Statistical Analysis: Gathering data points for linear regression or other statistical computations.
These scenarios leverage the power of IntStream
to handle large datasets efficiently and purposefully.
Lessons Learned
In conclusion, mastering the conversion of IntStream
to an array is a pivotal skill in Java 8 programming. Understanding how to create an IntStream
, seamlessly convert it to an array, filter elements, and apply custom operations opens avenues for efficient and expressive code.
By embracing IntStream
and the whole streams API, Java developers can greatly enhance productivity, readability, and performance of their applications.
For further reading on Java 8 Streams, check out the official Java documentation to delve deeper into the nuances of this powerful feature.
Get started with IntStream
in your projects today, and you'll find your Java experience transformed!
Feel free to leave questions or share your experiences with IntStream
in the comments below! Happy coding!