Mastering JW Options: Common Pitfalls in Java CLI Development
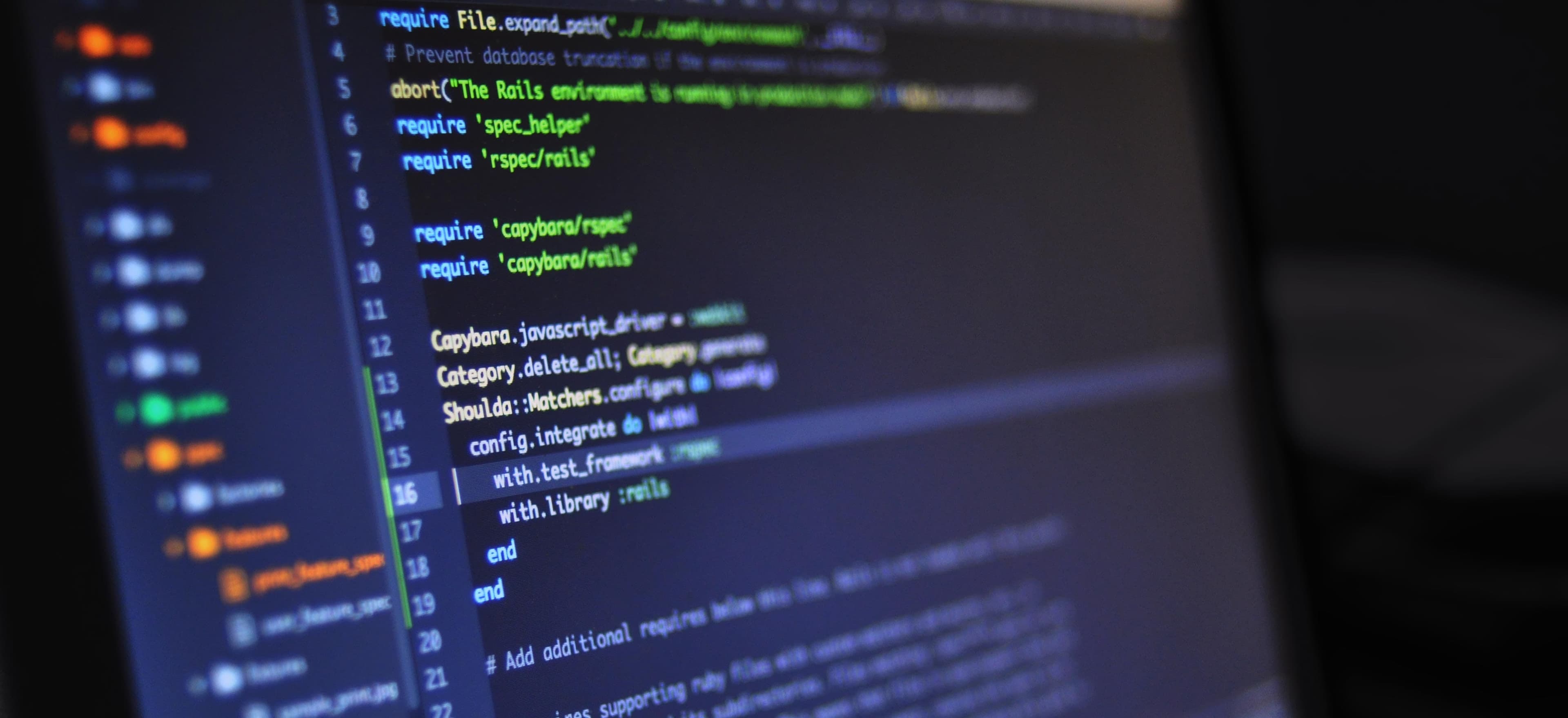
- Published on
Mastering JW Options: Common Pitfalls in Java CLI Development
Creating command-line interface (CLI) applications in Java can be both rewarding and challenging. Java is a robust programming language with strong community support, making it an excellent option for developers aiming to build CLI tools. However, implementing command-line options through libraries like JW Options can become tricky if you're not aware of common pitfalls. This blog post will explore these pitfalls and provide insights for mastering JW Options in your CLI development.
Understanding JW Options
JW Options is a small but powerful Java library that simplifies option handling in command-line applications. It enables you to define options and arguments with minimal configuration. The library not only helps in parsing commands but also validates input, meaning you can focus more on the functionality of your application rather than the underlying structure.
You can find the JW Options library here.
Why Use JW Options?
- Simplicity: Easy to set up and use.
- Type Safety: Handles various data types seamlessly.
- Validation: Helps in ensuring that the inputs received are valid.
Common Pitfalls and Solutions
Now, let’s dive into some frequent mistakes developers make when using JW Options and how to avoid them.
1. Incorrect Option Definition
One of the most common errors is improperly defining options. Each option must have a unique identifier and should properly specify whether it's a flag or requires an argument.
Here is an example snippet that demonstrates proper option definition:
import org.jwoptions.Options;
import org.jwoptions.Option;
public class CommandLineApp {
public static void main(String[] args) {
Options options = new Options();
Option help = options.add("help").asBoolean().withDescription("Show help information.");
Option filePath = options.add("file").asString().withDescription("Path to the input file.");
options.parse(args);
if (help.isPresent()) {
System.out.println("Usage: java CommandLineApp --file <path>");
return;
}
String file = filePath.value(); // Accessing the argument value
if (file == null) {
System.out.println("Error: File path is required!");
} else {
// Process the file
}
}
}
Why it matters: Failing to set the option correctly might lead to runtime exceptions, crashing your application or causing unexpected behavior.
2. Ignoring Input Validation
Another common pitfall is neglecting to validate user input. While JW Options provides basic validation, it’s essential to implement your own checks to ensure the input meets your application’s requirements.
Here's an improved snippet with validation:
if (filePath.isPresent()) {
String file = filePath.value();
if (!file.endsWith(".txt")) {
System.out.println("Error: Currently, only .txt files are supported.");
return;
}
// Proceed to process the .txt file
}
Why it matters: Input validation helps to avoid runtime errors and improves user experience by providing informative feedback.
3. Overcomplicating Argument Parsing
Another frequent mistake is overcomplicating the parse logic. Simplicity is key. When parsing arguments, one should focus on readability and maintainability.
Take a look at this streamlined approach:
options.parse(args);
if (options.unrecognizedOptions().isEmpty()) {
// No errors in the options, proceed
} else {
System.out.println("Unrecognized options: " + options.unrecognizedOptions());
}
Why it matters: Keeping the code simple makes it easier for both you and others to understand, contributing to better maintenance.
4. Not Considering Default Values
When using options, it's also crucial to consider the default values. Failing to do so might lead to null pointer exceptions or undefined behaviors in your application.
Here’s how to set default values effortlessly:
Option outputFile = options.add("output").asString().defaultValue("defaultOutput.txt")
.withDescription("Output file path (default: defaultOutput.txt)");
Why it matters: By providing defaults, you improve the usability of your CLI tool. It allows your application to work intuitively without always requiring explicit command arguments.
5. Failing to Handle Exceptions
Finally, one of the fundamental aspects often overlooked is exception handling. Any time you deal with user input, there's a risk of encountering unexpected situations. Wrapping your code in try-catch blocks can help you handle these gracefully.
Here is a basic way to implement exception handling:
try {
options.parse(args);
// Further processing...
} catch (IllegalArgumentException e) {
System.out.println("Error parsing arguments: " + e.getMessage());
}
Why it matters: Handling exceptions gracefully ensures greater reliability and allows your application to communicate errors effectively, without crashing unexpectedly.
Bringing It All Together
Mastering JW Options and recognizing these common pitfalls can significantly improve your Java CLI applications. The key takeaways include defining options correctly, validating user input, keeping parsing logic simple, using default values, and handling exceptions.
Additional Resources
By understanding these principles, you can build robust, user-friendly command-line applications that stand the test of time. Happy coding!