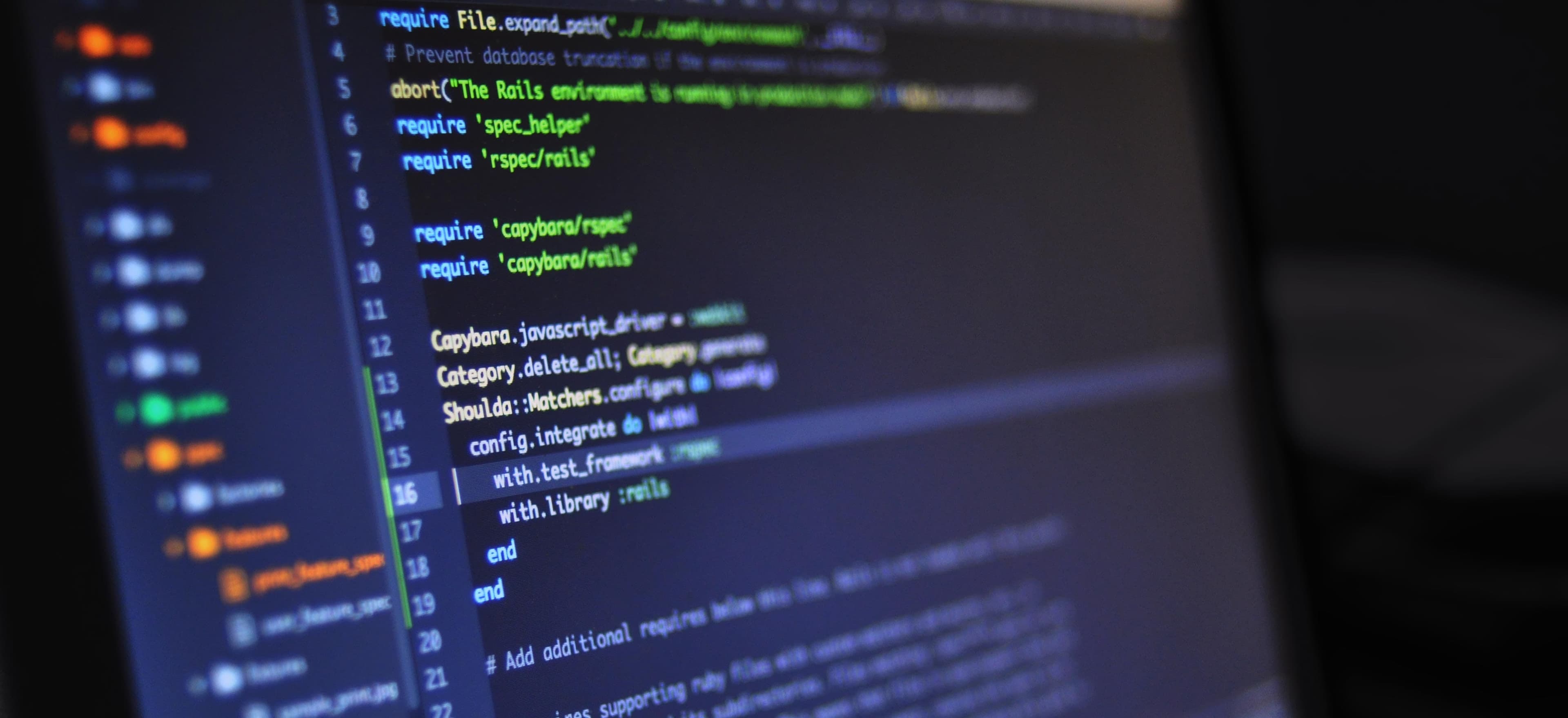
- Published on
Mastering Auto-Scrolling in JavaFX ListView
JavaFX is a powerful library designed for building rich internet applications. One of its many components is the ListView
, which provides a way to display a vertical list of elements. One common feature that developers often find necessary is auto-scrolling within a ListView
. This guide will explore how to implement auto-scrolling in a JavaFX application using ListView
.
What is Auto-Scrolling?
Auto-scrolling refers to the ability of a user interface component to scroll automatically as new data or content is added or when certain events occur. In the context of a ListView
, auto-scrolling ensures that the most recently added item is always visible to the user, creating a smooth and intuitive experience.
Prerequisites
Before diving into auto-scrolling functionality, ensure you have:
- Basic knowledge of Java and JavaFX
- Set up an environment ready for JavaFX development
- Java SDK installed (version 8 or higher)
You can download the latest JavaFX library from Gluon.
Setting Up Your Project
To start off, create a simple JavaFX project structure. Use your favorite IDE or command line tools to set up the environment. Below is a sample structure:
MyJavaFXProject/
│
├── src/
│ └── main/
│ └── java/
│ └── com/
│ └── example/
│ └── AutoScrollListView.java
└── lib/
Creating The Main Application
Let's create a basic JavaFX application with a ListView
. Here is a simple implementation:
package com.example;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ListView;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class AutoScrollListView extends Application {
private ObservableList<String> items;
@Override
public void start(Stage primaryStage) {
items = FXCollections.observableArrayList();
ListView<String> listView = new ListView<>(items);
Button addButton = new Button("Add Item");
addButton.setOnAction(event -> addItem(listView));
VBox vbox = new VBox(addButton, listView);
Scene scene = new Scene(vbox, 300, 250);
primaryStage.setScene(scene);
primaryStage.setTitle("Auto-Scrolling ListView Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
private void addItem(ListView<String> listView) {
items.add("Item " + (items.size() + 1));
// TODO: Implement auto-scroll
}
}
In this snippet, we have the beginnings of our application. A button allows users to add items to the ListView
. However, we will need to implement the auto-scroll functionality when items are added.
Implementing Auto-Scroll
To enable auto-scrolling, we can use the ListView
's scrollTo
method. This method scrolls the viewport to the specified item, ensuring that it is visible. Here is how you can implement it:
private void addItem(ListView<String> listView) {
String newItem = "Item " + (items.size() + 1);
items.add(newItem);
listView.scrollTo(items.size() - 1); // Scrolls to the last item
}
This code will effectively cause the ListView
to scroll to the last item whenever it is added. It uses the size()
method of the items
list to refer to the last index, ensuring that visibility is maintained.
Why Use scrollTo?
Using the scrollTo
method is essential for enhancing user experience. When dealing with long lists, users appreciate not having to manually scroll to the end to see recent additions. This small feature can significantly improve usability, particularly in chat interfaces or log viewers.
For more complex scrolling needs, where you might want to set scrolling behavior based on conditions, you may also want to explore the JavaFX ScrollBar class.
Enhancements
Smooth Scrolling Based on Data Addition
In situations where items are added rapidly, you could enhance the auto-scroll feature by introducing smooth scrolling rather than jumping straight to the last item. This can be done using a Timeline
or animation.
For instance, consider the below method to slowly scroll into position:
private void smoothScrollToBottom(ListView<String> listView) {
int lastIndex = items.size() - 1;
listView.scrollTo(lastIndex); // Jump to last
// Optionally, implement a loop to scroll continuously if necessary.
}
Example of Smooth Animation
Here is a mock-up of how you could implement a smoother scrolling effect:
private void addItem(ListView<String> listView) {
String newItem = "Item " + (items.size() + 1);
items.add(newItem);
// Start a smooth scrolling animation
Timeline timeline = new Timeline();
timeline.getKeyFrames().add(new KeyFrame(Duration.millis(100), e -> {
listView.scrollTo(items.size() - 1);
}));
timeline.setCycleCount(5); // Change the number as needed for more duration
timeline.play();
}
By employing a timeline, you engender an experience that feels more dynamic and less abrupt. Every little enhancement contributes to user satisfaction, eventually translating to greater application success.
Lessons Learned
Mastering auto-scrolling in JavaFX ListView
is a vital skill, especially for applications that deal with dynamic data. By implementing the methods described, you ensure that users can engage with your application effectively, making the most out of the user interface.
Next Steps
To delve deeper into JavaFX and its capabilities, check out the JavaFX Documentation. Additionally, experimenting with more complex components, and features of JavaFX can help refine your skills further.
Whether you are developing a chat application, an event logger, or any other data-driven interface, auto-scrolling in ListView
can significantly elevate user experience. Integrating such simple yet effective features will set your applications apart.
Happy coding!