WASM vs Traditional Coding: Which One to Choose?
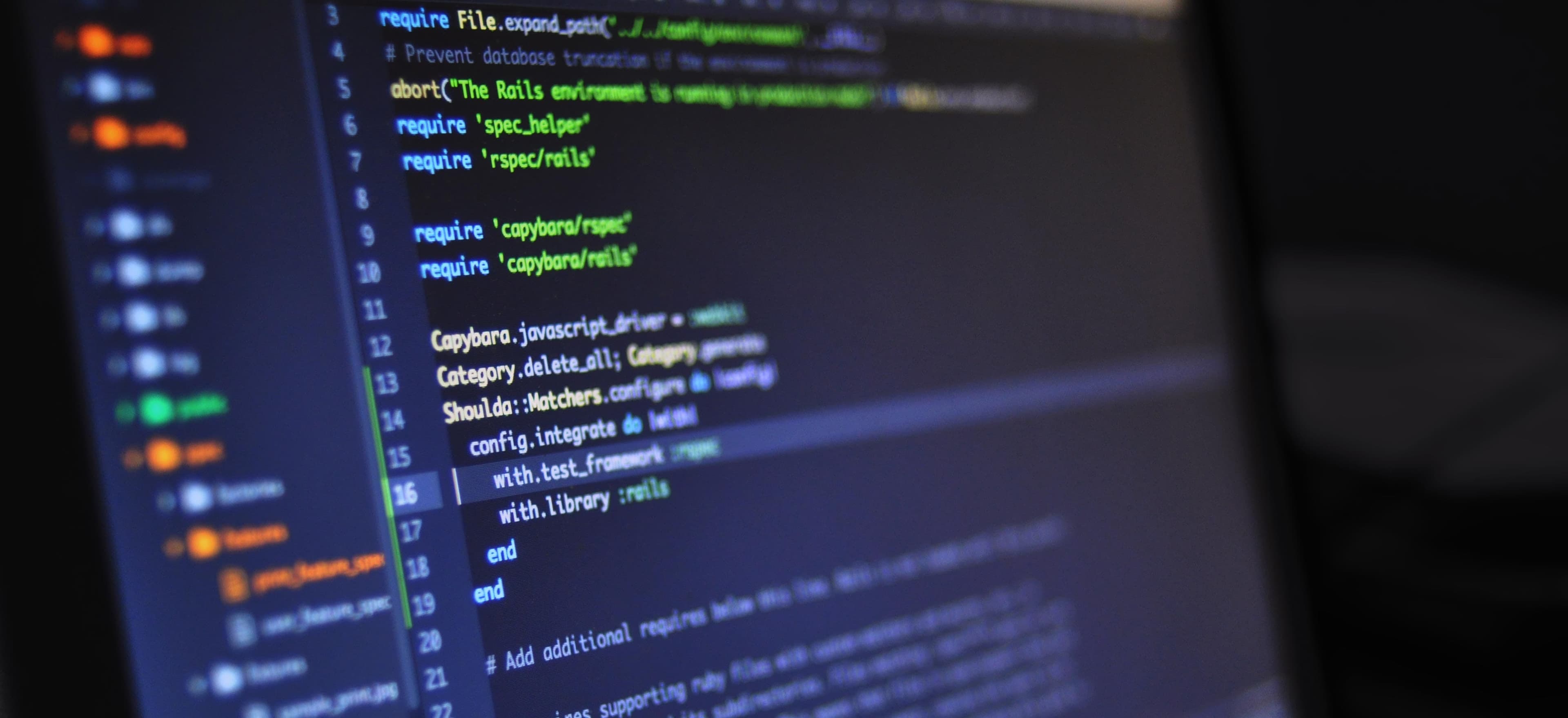
- Published on
WASM vs Traditional Coding: Which One to Choose?
WebAssembly (WASM) has emerged as a game-changing technology in the world of web development, making it possible to run code written in languages like C, C++, and Rust on the web. This opens up exciting possibilities for performance improvements and leveraging existing codebases. But how does it stack up against traditional web coding, typically done in JavaScript? In this blog post, we’ll delve into the pros and cons of WASM and traditional coding, helping you make an informed choice for your next project.
What is WebAssembly?
WebAssembly (WASM) is a low-level binary format designed to be a portable compilation target for high-level programming languages. This means you can write your application in a language like C, compile it to WASM, and run it in the browser alongside JavaScript. WASM is designed for performance and is executed at near-native speeds, making it an excellent choice for resource-intensive applications like games or complex calculations.
Why Choose WebAssembly?
-
Performance: WASM runs at near-native speed due to its low-level nature. It enhances performance for CPU-intensive applications, offering significantly faster execution times compared to JavaScript.
-
Language Flexibility: With WASM, developers are not limited to JavaScript. You can use languages such as Rust, C, and C++, benefiting from their performance optimizations and libraries.
-
Easier Porting: If you have a codebase written in another language, transitioning it to the web using WASM can be smooth thanks to the compiled output.
-
Security: WASM executes in a memory-safe and sandboxed environment, reducing risks related to native vulnerabilities.
What is Traditional Coding?
Traditional coding typically involves languages like JavaScript, HTML, and CSS. JavaScript is the main scripting language for web development, enabling interactive web applications. It has a vast ecosystem and is integrated into all major web browsers.
Why Choose Traditional Coding?
-
Wide Adoption and Community Support: JavaScript has become ubiquitous on the web, boasting a robust community and extensive resources. This makes it easy to find help or libraries to speed up development.
-
Direct DOM Manipulation: JavaScript interacts seamlessly with the Document Object Model (DOM), allowing for dynamic updates to the web page without needing a full reload.
-
Simplicity and Learning Curve: For beginners, JavaScript is generally easier to learn than compiling code with WASM. A significant number of tutorials, documentation, and courses are available.
-
Rich Ecosystem: The JavaScript ecosystem is rich with frameworks and libraries such as React, Angular, and Vue, making it easier to build complex applications quickly.
The Head-to-Head Comparison: Key Factors
Performance
WASM:
- WASM code is typically faster than JavaScript code, especially for computationally intensive tasks.
- The binary format allows for faster decoding in browsers, leading to quicker load times.
Traditional Coding:
- JavaScript engines have improved significantly, but it might still lag behind WASM in performance for heavy tasks.
Development Speed and Complexity
WASM:
- Initial setup can be more complex due to the need for compiling and configuring.
- Debugging can be less straightforward compared to writing and debugging JavaScript.
Traditional Coding:
- Quick prototyping and rapid development are more achievable.
- Immediate feedback is available through the browser, making it easier to edit on-the-fly.
Integration with Existing Tools
WASM:
- Integrating with existing web technologies might require extra effort, particularly for developers unfamiliar with WASM.
- However, it plays nicely with JavaScript, allowing for the best of both worlds.
Traditional Coding:
- Robust integration with HTML and CSS makes JavaScript straightforward for most web-related tasks.
- Works excellently with various frameworks and tools.
Use Cases Where Each Shines
When to Use WASM
-
Game Development: If you are building a web-based game requiring performance handling, WASM can tremendously enhance the gaming experience.
-
Advanced Data Analytics: For applications that require heavy computations, such as simulations or mathematical computations, consider leveraging WASM.
-
Porting Existing Libraries: If you want to bring existing C/C++ libraries to the web with minimal changes, WASM is the way to go.
When to Use Traditional Coding
-
Web Applications: If you are building a standard web application focused on interactivity rather than pure performance, JavaScript suffices.
-
Prototyping: When speed-to-market is critical, traditional coding allows for rapid iterations and updates.
-
User Interfaces: Given JavaScript’s superior integration with the DOM, traditional coding shines in building dynamic user interfaces.
A Case Study: WASM in Action
Let’s take a look at a simple case where we leverage WASM in a project. Suppose we have a C function that performs a Fibonacci calculation. Instead of implementing it in JavaScript, we compile it to WASM.
C Code (fibonacci.c)
#include <stdint.h>
uint32_t fibonacci(uint32_t n) {
if (n <= 1) {
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2);
}
Compilation to WASM
To compile this function to WebAssembly, we can use Emscripten:
emcc fibonacci.c -o fibonacci.js -s EXPORTED_FUNCTIONS='["_fibonacci"]' -s WASM=1
This creates fibonacci.js
and fibonacci.wasm
. The EXPORTED_FUNCTIONS
flag specifies which functions are available to call from JavaScript.
Calling WASM from JavaScript
Now let's bridge our WASM function with JavaScript:
const Module = require('./fibonacci.js');
Module.onRuntimeInitialized = async _ => {
const fib = Module._fibonacci;
console.log(fib(10)); // Outputs 55
};
The Why Behind This Code
By compiling the Fibonacci function written in C to WASM, we achieved two key metrics: performance and language specialization. Instead of writing an inefficient recursive algorithm in JavaScript, we leverage a language built for such tasks.
Final Thoughts
Choosing between WASM and traditional coding boils down to the specific needs of your project. WebAssembly offers undeniable advantages in performance and can be a critical component for computation-heavy applications. Traditional JavaScript coding remains the king for rapid web development, interactivity, and ease of use.
As the web continues to evolve, merging these approaches creates exciting possibilities. By harnessing the strengths of both WASM and traditional coding, developers can create high-performing, interactive web applications to meet the ever-growing expectations of users.
For more information on WASM and how to get started with it, check out MDN Web Docs on WebAssembly and Emscripten Documentation.
Whether your next project leans towards WASM or traditional coding, understanding both provides you with a powerful toolkit. Happy coding!
Checkout our other articles