Troubleshooting Service Discovery Issues in Java DC/OS Apps
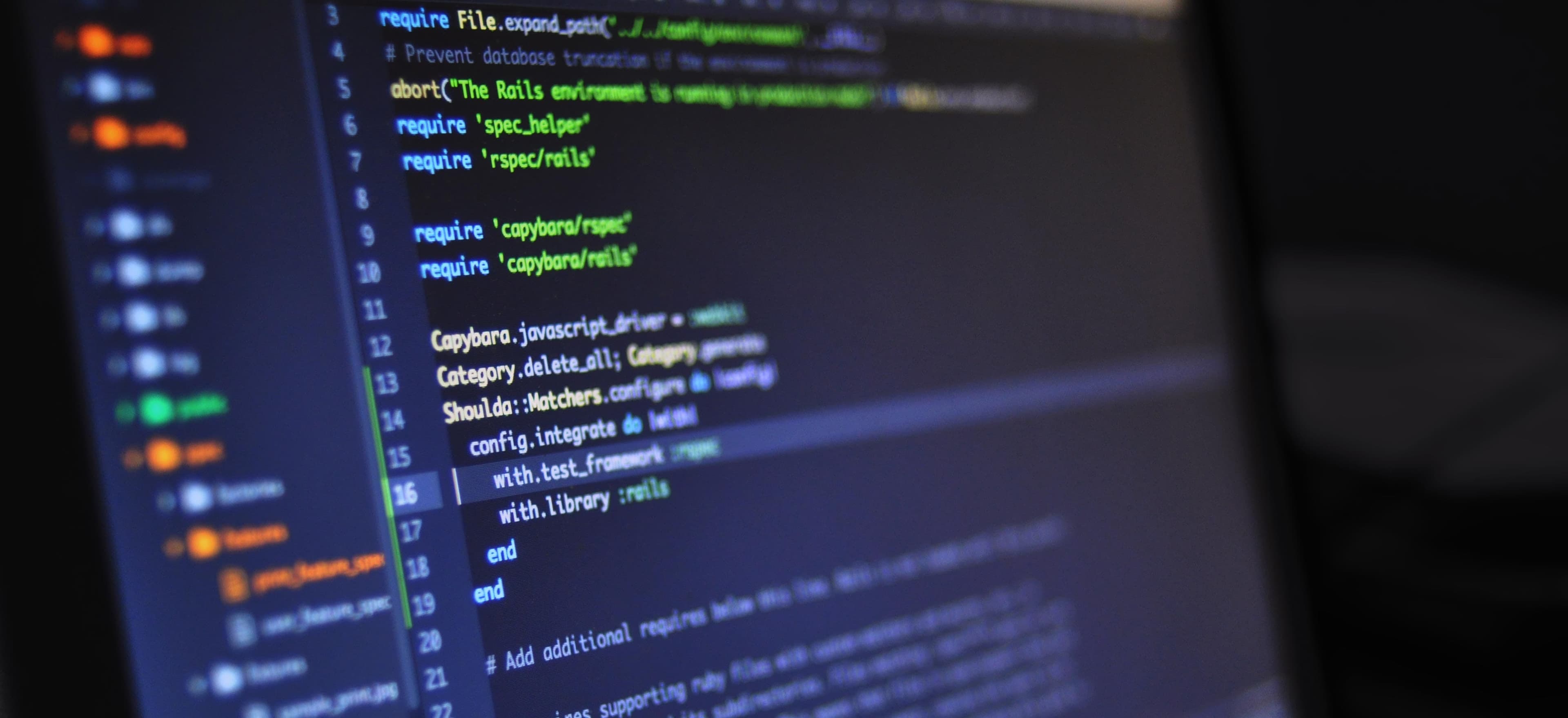
- Published on
Troubleshooting Service Discovery Issues in Java DC/OS Apps
Service discovery is a crucial part of modern distributed systems, particularly when deploying applications with container orchestration platforms like DC/OS (Datacenter Operating System). When services are not able to locate each other, it can lead to performance bottlenecks and even application failure. In this blog post, we will explore common service discovery issues in Java DC/OS applications and provide troubleshooting techniques.
Table of Contents
- What is Service Discovery?
- Common Service Discovery Methods in Java
- Issues You May Encounter
- Troubleshooting Techniques
- Best Practices for Service Discovery in Java DC/OS Apps
- Conclusion
What is Service Discovery?
Service discovery refers to the process of automatically detecting devices and services on a network. In microservices architecture, where multiple services need to communicate with one another, effective service discovery is essential. DC/OS provides various tools and libraries that facilitate service discovery for Java applications, often handling the heavy lifting and allowing developers to focus on their code.
Common Service Discovery Methods in Java
In a Java application, service discovery can be achieved using several methods, including:
-
DNS-Based Discovery: Using DNS records to automatically resolve service names to their respective IP addresses.
-
Client-Side Load Balancing: Tools like Ribbon or Spring Cloud allow clients to directly discover services and perform load balancing.
-
Service Registries: Systems like Consul, Zookeeper, or Eureka serve as a registry where services can register and be discovered.
Example: Using Spring Cloud Eureka
If you're using Spring Cloud and Eureka for service discovery, registering a service could look like this:
@SpringBootApplication
@EnableEurekaClient
public class MyEurekaClientApplication {
public static void main(String[] args) {
SpringApplication.run(MyEurekaClientApplication.class, args);
}
}
In this example, the @EnableEurekaClient
annotation allows this application to register itself with the Eureka server, making it discoverable to other services.
Why Use Eureka?
Using a service registry simplifies service discovery, allowing multiple instances of a service to register and manage their lifecycle automatically.
Issues You May Encounter
Despite the robust mechanisms provided for service discovery, several issues can arise:
-
Service Not Registering: Sometimes a service fails to register due to misconfigurations, network issues, or initialization order.
-
Service Not Discoverable: Services may be registered but not discoverable due to network partitions or security groups.
-
Stale Instances: Occasionally, service registries may contain stale or dead instances, leading to unnecessary failures.
-
Multiple Instances Conflict: Identical service instances may conflict with each other, causing inconsistent discovery results.
Troubleshooting Techniques
1. Check Service Registration
First, verify whether the service is correctly registering with the registry. Access the DC/OS web interface or query the registry's API to check the list of currently registered services.
curl http://<Eureka_Server_IP>:<Eureka_Server_Port>/eureka/apps
Ensure your service appears in the response. If it’s not registered, revisit your service’s configuration.
2. Network Configuration
Network issues can often lead to discovery problems. Ensure that all services can communicate with the service registry and each other. Use the following methods to diagnose network issues:
-
Ping Services: Use the ping command to check connectivity.
-
Port Availability: Use
netstat
to ensure that the necessary ports are open and listening.
3. Logs and Monitoring
Examine application and service registry logs for any warning or error messages. These logs can provide insight into potential issues with the registration process or communication failures.
tail -f /path/to/your/log/file.log
Adding appropriate logging in your application will also provide context on service discovery events. For instance:
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void registerService() {
try {
// registration logic
logger.info("Registering service with Eureka");
} catch (Exception e) {
logger.error("Failed to register service", e);
}
}
4. Configuration Validation
Ensure that your application’s configuration (e.g., application.yml
or application.properties
) contains the correct registry URL, service name, and, if necessary, authentication credentials. An example configuration for Eureka would look like this:
eureka:
client:
service-url:
defaultZone: http://<Eureka_Server_IP>:<Eureka_Server_Port>/eureka/
5. Stale Instances Management
If stale instances are a problem, ensure that your registry is configured to remove unhealthy or stale instances automatically. In a Eureka-based system, you can configure the instance lease expiration settings.
6. Client-Side Discovery Configurations
If using a client-side load balancer, ensure that it’s correctly implemented and that your client-side configuration matches the service name specified in the registry.
Best Practices for Service Discovery in Java DC/OS Apps
-
Health Checks: Implement health checks on both the application and service registry to ensure that only healthy services are available for discovery.
-
Consistent Configuration: Centralize your configuration management to avoid discrepancies across different environments.
-
Monitoring and Alerting: Utilize monitoring tools to track the health and performance of the service discovery process.
-
Versioning: Use versioning in service names to manage deployment without service conflicts.
In Conclusion, Here is What Matters
Service discovery plays a fundamental role in the efficiency of Java applications deployed on platforms like DC/OS. By understanding common service discovery issues and implementing troubleshooting techniques as we've discussed, you can maintain a robust communication layer for your distributed applications.
By following best practices, you can enhance your service discovery architecture, ensuring high availability and performance for your microservices. Remember, an ounce of prevention is worth a pound of cure. Regularly monitor and validate your service discovery mechanism to mitigate issues before they occur.
For further reading on service discovery concepts and patterns, consider exploring Spring Cloud or Microservices: Principles, Practices, and Patterns. Happy coding!
Checkout our other articles