Common Pitfalls in Implementing Lender-Borrower Patterns
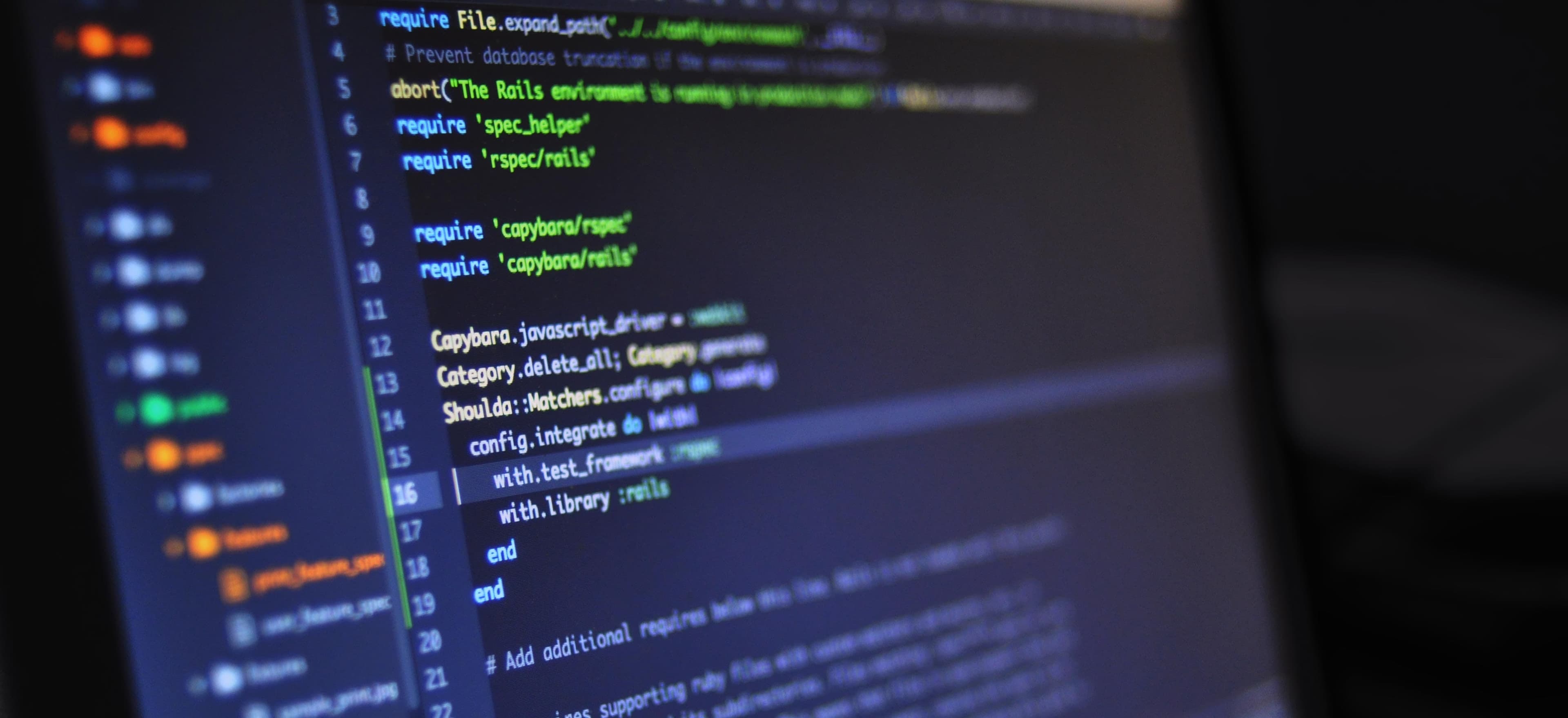
- Published on
Common Pitfalls in Implementing Lender-Borrower Patterns in Java
When engaging in software design, especially in situations involving loans or borrowings, the Lender-Borrower pattern surfaces frequently. As developers, understanding this pattern allows us to handle complex relationships more smoothly. However, common pitfalls can arise during its implementation. In this blog post, we discuss the key strategies to manage these pitfalls effectively and present code snippets to illustrate our points.
Understanding the Lender-Borrower Pattern
The Lender-Borrower pattern relates to a situation where one entity (the lender) provides resources to another entity (the borrower), who then returns those resources after use. It is crucial in financial applications, library systems, and any scenario where resource management is critical.
Common Pitfalls
1. Poor Resource Management
The most critical aspect of the Lender-Borrower pattern is resource management. A common mistake is failing to release resources promptly upon completion. This can lead to memory leaks and overall application sluggishness.
Example:
class Resource {
public void use() {
System.out.println("Resource is being used...");
}
public void release() {
System.out.println("Resource has been released.");
}
}
// Borrower class
class Borrower {
private Resource resource;
public Borrower(Resource resource) {
this.resource = resource;
}
public void borrow() {
resource.use();
// Forgetting to release can cause issues
}
}
Why is this an issue? In the example above, the resource is not released after usage, leading to potential memory leaks. To avoid this, ensure you define a clear return policy for borrowed resources.
Best Practice
Always release resources in a finally
block or use Java’s try-with-resources statement:
try (Resource resource = new Resource()) {
resource.use();
} // Automatically calls release method
2. Lack of Synchronization
When multiple borrowers attempt to borrow the same resource, race conditions can occur without proper synchronization. This may cause inconsistent states and unexpected behavior.
Example:
class SynchronizedResource {
public synchronized void use() {
System.out.println("Resource is exclusively in use...");
}
}
Why synchronize? In this situation, using the synchronized keyword ensures that one thread will access the resource at a time. Synchronization maintains data integrity but can impact performance if used excessively.
3. Non-Standard Error Handling
Many implementations overlook robust error handling. When exceptions occur, any remaining references will need to be reset or released gracefully. Neglecting this can compromise application stability.
Example:
class Borrower {
public void borrowResource() {
try {
Resource resource = new Resource();
resource.use();
} catch (Exception e) {
System.out.println("An error occurred: " + e.getMessage());
// Missing resource cleanup
}
}
}
Best Practice
Always ensure to include cleanup in the catch block or finally block:
Resource resource = null;
try {
resource = new Resource();
resource.use();
} catch (Exception e) {
System.out.println("An error occurred: " + e.getMessage());
} finally {
if (resource != null) {
resource.release(); // Ensures resource is cleaned up
}
}
4. Bottlenecks Due to Overhead
Creating too many instances of resources can lead to bottlenecks, as they may consume memory and lead to increased latency. In designs using shared resources, consider pooling mechanisms.
Example:
class ResourcePool {
private final List<Resource> availableResources = new ArrayList<>();
// Initialization logic...
}
Why Use a Pool? A resource pool allows multiple borrowings without needing to create new instances each time, significantly enhancing performance and resource utilization.
Alternative Implementations
Consider using a third-party library such as DBCP or HikariCP, which provide robust connection pooling for database access while allowing you to focus on other application logic.
5. Not Following Design Patterns
Not adhering to established patterns like Dependency Injection (DI) or the Singleton pattern can exacerbate issues. Instantiating resources directly in the borrower class can lead to tight coupling and hinder testing.
Example:
class Borrower {
private Resource resource;
// Constructor injection for better testability
public Borrower(Resource resource) {
this.resource = resource;
}
}
Summary of Best Practices
- Release Resources: Always ensure that resources are appropriately released after use.
- Synchronize Access: Manage parallel access to shared resources to prevent inconsistency.
- Implement Error Handling: Use try-catch-finally blocks effectively to ensure proper resource management during errors.
- Utilize Resource Pools: Use pooling for efficient resource handling and minimize bottlenecks.
- Follow Design Patterns: Understand and implement design principles to ensure lower coupling and better testability.
Bringing It All Together
Implementing the Lender-Borrower pattern in Java efficiently requires an understanding of the common pitfalls and strategies to mitigate them. From ensuring proper resource management to utilizing design patterns effectively, these steps play a critical role in enhancing the stability and performance of applications involving loans or borrowings.
Further Reading
- Oracle Java Documentation
- Understanding Java Synchronization
Integrate these insights into your projects to optimize not only your code's performance but also your development process. Happy coding!
Checkout our other articles