Understanding the Common Pitfalls of Java Executor Service
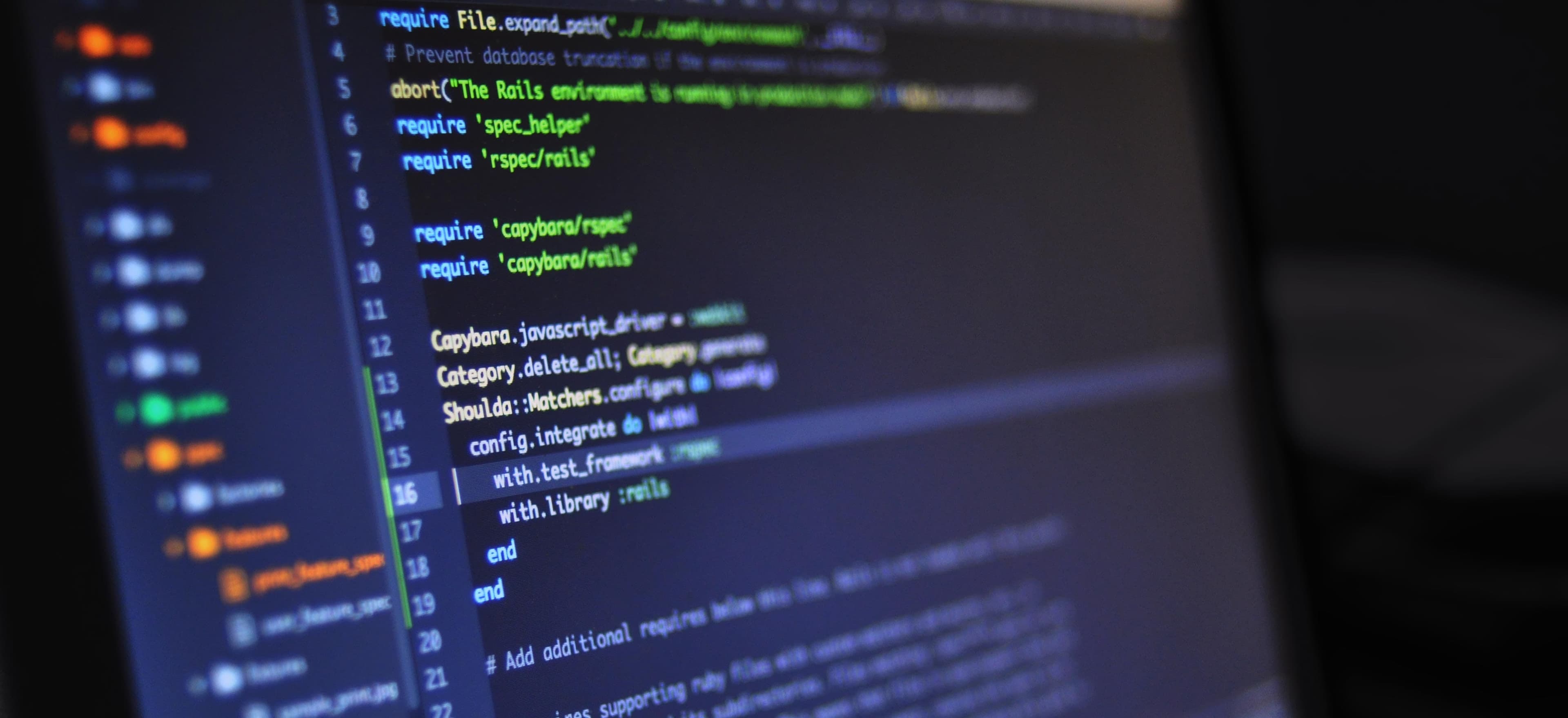
- Published on
Understanding the Common Pitfalls of Java Executor Service
Java's ExecutorService
is a powerful framework for managing thread execution, which abstracts the complexities of thread management and allows developers to focus on the task at hand. However, as with any powerful tool, improper usage can lead to significant pitfalls. This article delves into these common pitfalls and offers best practices to ensure you leverage the full potential of ExecutorService
.
What is ExecutorService?
The ExecutorService
interface, part of the java.util.concurrent
package, is designed to execute Runnable
and Callable
tasks asynchronously. With ExecutorService
, you can easily manage the lifecycle of threads, submit tasks for execution, and retrieve results without dealing with the low-level intricacies of thread management.
Here's a basic way to create an ExecutorService
:
ExecutorService executor = Executors.newFixedThreadPool(5);
In this example, we create a thread pool with five threads, allowing us to run multiple tasks concurrently.
Common Pitfalls of ExecutorService
- Not Shutting Down the Executor
Failing to properly shut down an ExecutorService
can lead to resource leaks and memory issues. If you do not call shutdown()
or shutdownNow()
, the application may not terminate as expected, often keeping threads alive while waiting for tasks that might never complete.
Best Practice:
Always ensure you shut down the executor after use:
executor.shutdown(); // Initiates an orderly shutdown
In cases where you need to interrupt currently executing tasks, consider using:
executor.shutdownNow(); // Attempts to stop all actively executing tasks
- Using an Unbounded Executor
Creating an ExecutorService
without a limit on the number of threads can lead to OutOfMemoryError and performance degradation. For example, if you're not using managed thread pools, each task could spawn a new thread, escalating resource consumption exponentially.
Example of Problematic Code:
ExecutorService executor = Executors.newCachedThreadPool();
Here, the newCachedThreadPool()
allows unrestricted threads, which could lead to excessive resource use.
Recommended Approach:
Utilize a fixed thread pool or a bounded queue to manage your threads effectively:
ExecutorService executor = Executors.newFixedThreadPool(10);
- Ignoring Future Handling
When you submit tasks to an ExecutorService
, it returns a Future
object. Ignoring the handling of this Future
can prevent you from catching exceptions that occur during task execution.
Example:
Future<Integer> future = executor.submit(() -> {
// some task
return 1 / 0; // This will throw an ArithmeticException
});
Handling Future:
Always check for result retrieval and handle exceptions properly:
try {
Integer result = future.get(); // This blocks until the task completes
} catch (ExecutionException e) {
// Handle the exception thrown during task execution
e.getCause().printStackTrace();
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore interrupted status
}
- Not Handling InterruptedException
When a task is executing, it needs to be able to handle interruptions gracefully. If the thread executing the task is interrupted, it should stop what it is doing and exit.
Example of Poor Handling:
public void myTask() {
while (true) {
// Perform task
}
}
Recommended Code:
public void myTask() {
while (!Thread.currentThread().isInterrupted()) {
// Perform task
}
}
This allows your task to exit cleanly whenever interrupted, releasing resources appropriately.
- Blocking Calls in ExecutorTasks
Placing blocking calls in Runnable
or Callable
tasks can lead to depleted threads, starving your thread pool and causing tasks to wait indefinitely.
Example:
executor.submit(() -> {
// Blocking call
Thread.sleep(5000); // This blocks the thread for 5 seconds
});
Solution:
If you have blocking operations, consider using the Future
's timeouts or options like CountDownLatch
or Semaphore
for better control over task execution.
- ExecutorService Resource Leak on Exceptions
If an exception occurs inside a task, and it is not handled properly, it can terminate the thread prematurely, leading to an unaccounted thread. The pool will keep trying to create new threads, further depleting the resources.
Example:
executor.submit(() -> {
throw new RuntimeException("Some error");
});
Managing Exceptions:
Wrap the task in a try-catch block:
executor.submit(() -> {
try {
// Task logic here
} catch (Exception e) {
// Handle/log the exception
}
});
Summary
In closing, while Java's ExecutorService
provides a robust framework for concurrent programming, it comes with its share of pitfalls. By understanding and addressing these concerns, such as ensuring proper shutdown, managing thread limits, handling exceptions, and gracefully managing interruptions, you can enhance the reliability and performance of your applications.
For more insights on concurrency in Java, you can refer to the Java concurrency tutorial or explore more advanced patterns, such as Fork/Join and the CompletableFuture API.
Happy coding, and may you avoid the pitfalls of Java's threading world!