Eliminating Noise: Streamline Your Stack Trace Analysis
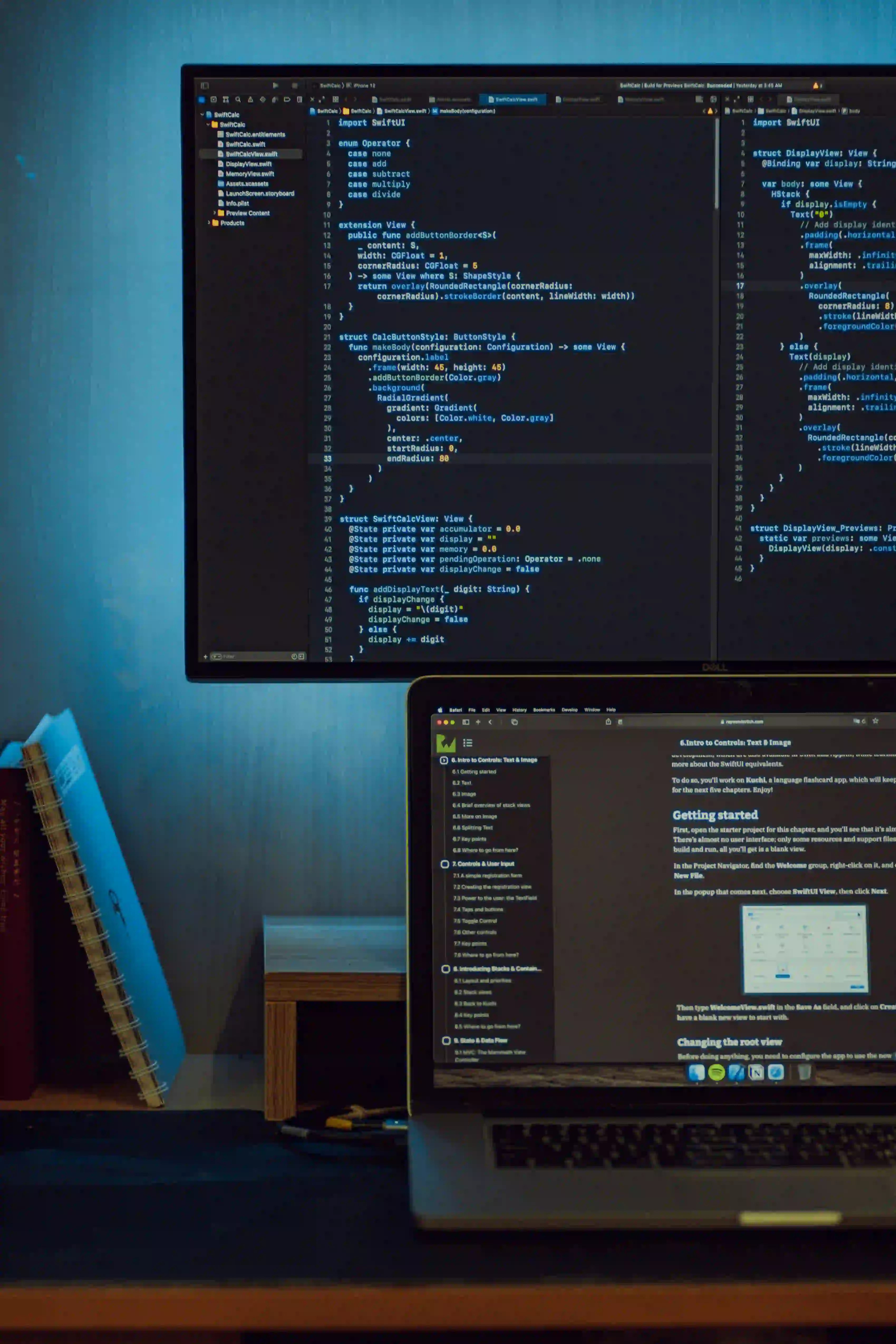
Eliminating Noise: Streamline Your Stack Trace Analysis in Java
Stack traces in Java can often be intimidating. They can look like a long, twisted road of complexity filled with confusing errors and irrelevant information. However, mastering stack trace analysis can be the key to debugging your applications efficiently. In this blog post, we will explore techniques to streamline your stack trace analysis process, ultimately making your debugging experience more effective.
What is a Stack Trace?
A stack trace is a report of the active stack frames at a certain point in time during the execution of a program. It can be generated whenever an exception is thrown, providing developers with a roadmap of method calls leading to the point of failure. Understanding stack traces is essential for diagnosing issues within your Java applications.
Example of a Stack Trace
Here's a simplified example of a stack trace generated when a NullPointerException
is thrown:
Exception in thread "main" java.lang.NullPointerException
at com.example.MyClass.myMethod(MyClass.java:10)
at com.example.Main.main(Main.java:5)
In this example, the trace shows that the error originated in MyClass
on line 10, which was called by Main
on line 5.
Why Is Stack Trace Analysis Important?
Analyzing stack traces effectively saves time and effort. It helps you:
- Identify the root cause of exceptions.
- Navigate through your codebase quickly.
- Improve debugging skills through experience.
- Reduce downtime by enabling faster issue resolution.
By eliminating noise from your stack traces, you can focus on what really matters.
Techniques for Streamlining Stack Trace Analysis
1. Focus on Relevant Information
In a typical stack trace, you might encounter numerous entries. However, not all of them are valuable for diagnosing an issue. Here’s how to filter effectively:
-
Locate the Exception Type: The first line usually contains the exception type. Get acquainted with common exceptions such as
NullPointerException
,ArrayIndexOutOfBoundsException
, andIOException
. -
Examine the First Entry: The first occurrence in the trace often indicates where the problem originated. This is your initial clue.
-
Ignore Framework Noise: If your stack trace includes library or framework code that you did not write, you can often ignore these entries unless you're using a specific feature or method from that framework.
2. Use Custom Exception Messages
Custom exception messages can make a significant difference. Instead of relying solely on standard Java exceptions, consider creating your exceptions. Here’s an example:
public class InvalidUserInputException extends Exception {
public InvalidUserInputException(String message) {
super(message);
}
}
Why Custom Exceptions?
By crafting your custom exceptions, you can provide meaningful context in your exception messages. When caught, they help clarify the exact nature of the issue. For instance:
try {
validateUserInput(input);
} catch (InvalidUserInputException e) {
System.out.println("User input is invalid: " + e.getMessage());
}
This approach enhances the clarity of your stack traces since it supplant standard error messages with custom context.
3. Integrate Logging Parameters
Logging is crucial in Java applications, especially for error tracking. Adding relevant parameters to your logs can help correlate logs with stack traces. Consider using a logging framework like SLF4J for a more flexible logging mechanism. Here’s a brief example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void findUserById(Long userId) {
try {
User user = userRepository.findById(userId).orElseThrow(() -> new InvalidUserInputException("User not found with ID: " + userId));
logger.info("User found: {}", user.getUsername());
} catch (InvalidUserInputException e) {
logger.error("Error occurred: {}", e.getMessage(), e);
}
}
}
Why Logging?
Effective logging can bridge the gap between what happens in your application and the events leading up to it. This makes it easier to decipher stack traces when debugging.
4. Use Visual Debugging Tools
Sometimes, code can be convoluted. Visual debugging tools such as the ones built into IDEs like IntelliJ IDEA or Eclipse can greatly assist in stack trace analysis. These tools visually represent the call stack, allowing you to explore methods and variable states with ease.
- Quick Navigation: Click on the method name to jump directly to the location of the code.
- Hover Over Variables: Check variable states at various points in the stack.
Utilizing visual debuggers streamlines the analysis process, providing an intuitive, user-friendly method for understanding stack traces.
5. Implement Exception Handlers
Setting up global exception handlers can aid in consistent stack trace management. Here’s an example of how to achieve this using a Java Servlet Filter:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
public class GlobalExceptionHandlerFilter implements Filter {
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
try {
chain.doFilter(request, response);
} catch (Exception e) {
logException(e);
HttpServletResponse httpResponse = (HttpServletResponse)response;
httpResponse.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "An error occurred");
}
}
private void logException(Exception e) {
// Logging the exception
Logger logger = LoggerFactory.getLogger(GlobalExceptionHandlerFilter.class);
logger.error("Exception occurred: {}", e.getMessage(), e);
}
}
Why Global Handlers?
This pattern centralizes exception management in your application, simplifying your stack trace outputs. It reduces repetitive boilerplate code as you catch the exceptions consistently in one place.
6. Measure and Analyze Trends
Over time, you might notice patterns in stack traces. Analyzing trends in exception occurrences can help you proactively address recurring problems. Here are some indicators to look for:
- Number of occurrences of a specific exception.
- Common root causes of failures.
- Specific modules or components frequently while being problematic.
Utilizing a tool like Sentry or Logstash can aid in tracking and analyzing these trends automatically.
Key Takeaways
Streamlining your stack trace analysis can significantly enhance your debugging capabilities. By filtering through only essential information, implementing custom exception classes, leveraging logging, utilizing visual debugging tools, employing global exception handlers, and analyzing trends, you can transform your debugging methodology into a far more efficient process.
In the complex world of Java development, creating an organized stack trace analysis approach is not just a beneficial practice—it is a necessity. By focusing on what truly matters, you can minimize unnecessary noise and drastically reduce the time spent debugging.
For further reading, you might find these links valuable:
- Understanding Java Stack Traces
- Best Practices for Logging in Java
Happy coding, and may your stack traces always be clear!