Mastering DispatcherServlet: 5 Common Pitfalls to Avoid
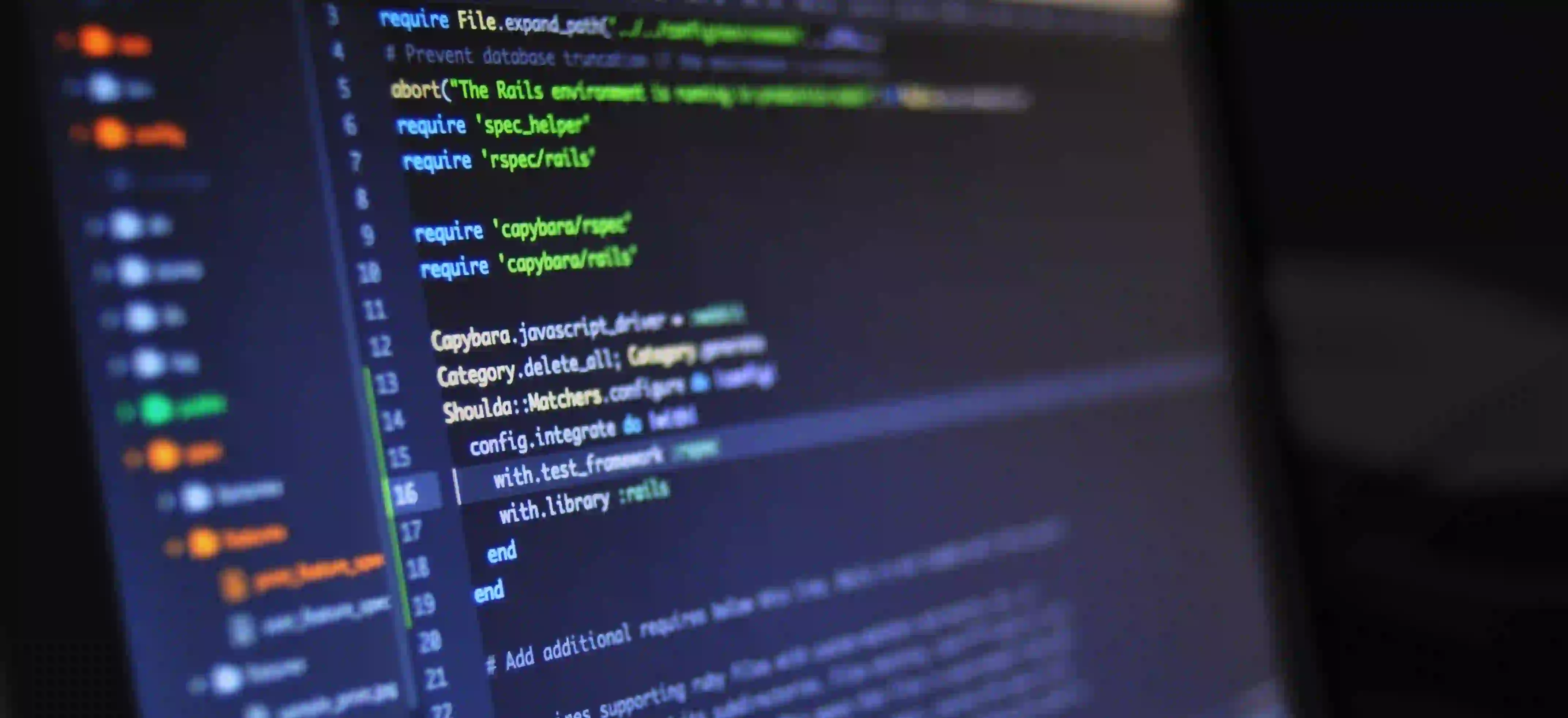
Mastering DispatcherServlet: 5 Common Pitfalls to Avoid
When developing web applications with the Spring Framework, the DispatcherServlet is a pivotal component that acts as the front controller, handling all incoming requests and directing them to the appropriate handlers. While mastering DispatcherServlet can tremendously enhance your application, a few common pitfalls can lead to frustrating bugs and ineffective performance. In this blog post, we will explore five pitfalls to avoid while using DispatcherServlet and provide you with practical solutions and code examples to navigate these challenges successfully.
Table of Contents
- Understanding DispatcherServlet
- Pitfall #1: Misconfigured Request Mappings
- Pitfall #2: Ignoring Exception Handling
- Pitfall #3: Overcomplicating Handler Methods
- Pitfall #4: Inefficient Resource Handling
- Pitfall #5: Not Leveraging Interceptors
- Conclusion
Understanding DispatcherServlet
Before jumping into the pitfalls, let’s briefly discuss what DispatcherServlet is and its role in a Spring MVC application.
DispatcherServlet acts as a front controller in a Spring Web application. It intercepts all HTTP requests and dispatches them to the appropriate controllers. The key functionalities of the DispatcherServlet include:
- Routing requests to correct controllers
- Handling view resolution
- Managing request attributes and parameters
- Integrating with other Spring components
For more in-depth understanding, check out the official Spring MVC Documentation.
Pitfall #1: Misconfigured Request Mappings
The Problem
A common mistake developers make is misconfiguring request mappings. This often leads to HTTP 404 errors, where the desired endpoint is unreachable.
The Solution
Make sure to specify your @RequestMapping
annotations properly, ensuring they match the incoming requests' URLs. For example:
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
@PostMapping("/data")
public ResponseEntity<String> postData(@RequestBody MyData data) {
// process data
return ResponseEntity.ok("Data processed!");
}
}
Why This Matters: Each method must be correctly mapped to the expected endpoint to ensure that requests are routed properly. Testing your endpoints with tools like Postman or cURL can help catch these mistakes early.
Pitfall #2: Ignoring Exception Handling
The Problem
Failing to handle exceptions effectively can result in poor user experiences. If an error occurs, users may see generic errors or server stack traces, which can be confusing and unhelpful.
The Solution
Implement centralized exception handling by using the @ControllerAdvice
annotation, which allows you to manage exceptions across your entire application.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(CustomException.class)
public ResponseEntity<String> handleCustomException(CustomException ex) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body(ex.getMessage());
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGeneralException(Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An unexpected error occurred.");
}
}
Why This Matters: Centralizing your exception handling improves the maintainability of your code and ensures a consistent response structure for errors, enhancing user experience.
Pitfall #3: Overcomplicating Handler Methods
The Problem
Another pitfall is creating overly complex handler methods, which can lead to difficulties in maintenance and testing. Handlers should be concise and focused.
The Solution
Break down complex logic into service classes or utility methods. Here’s an example of how to simplify handlers:
@RestController
@RequestMapping("/api")
public class OrderController {
private final OrderService orderService;
public OrderController(OrderService orderService) {
this.orderService = orderService;
}
@PostMapping("/orders")
public ResponseEntity<Order> createOrder(@RequestBody OrderRequest orderRequest) {
Order order = orderService.createOrder(orderRequest);
return ResponseEntity.status(HttpStatus.CREATED).body(order);
}
}
@Service
public class OrderService {
public Order createOrder(OrderRequest orderRequest) {
// Business logic for creating order
// Validation, saving to database, etc.
return new Order(); // return created order
}
}
Why This Matters: By delegating complex logic away from the controller, you keep your code modular, easier to read, and more testable.
Pitfall #4: Inefficient Resource Handling
The Problem
Neglecting resource handling can lead to performance issues. Using blocking I/O in a web application can slow down response time, especially under heavy load.
The Solution
Consider using non-blocking I/O with Spring WebFlux when handling resources. Here’s a simple example of how you can return data in a non-blocking manner:
@RestController
@RequestMapping("/api/products")
public class ProductController {
private final ProductService productService;
public ProductController(ProductService productService) {
this.productService = productService;
}
@GetMapping("/{id}")
public Mono<Product> getProduct(@PathVariable String id) {
return productService.findById(id);
}
}
Why This Matters: Non-blocking I/O can help your application efficiently handle more concurrent requests, leading to a better user experience and higher throughput.
Pitfall #5: Not Leveraging Interceptors
The Problem
Interceptors are an underrated feature in the Spring MVC ecosystem. Failing to use them can result in code duplication, particularly for pre-processing requests and post-processing responses.
The Solution
Utilize HandlerInterceptors to encapsulate common processing logic in one place. Here is how you can implement an interceptor:
public class LoggingInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
System.out.println("Request URL: " + request.getRequestURI());
return true;
}
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response,
Object handler, ModelAndView modelAndView) {
System.out.println("Response Status: " + response.getStatus());
}
}
Registering the Interceptor:
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(new LoggingInterceptor());
}
}
Why This Matters: Using interceptors enhances code reusability and separates concerns, leading to a cleaner application architecture.
Wrapping Up
Mastering DispatcherServlet can significantly enhance your Spring applications, but it’s crucial to be aware of potential pitfalls. By avoiding misconfigurations, implementing robust exception handling, simplifying handler methods, efficiently managing resources, and leveraging interceptors, you can create applications that are easier to maintain, perform better, and provide an enhanced user experience.
We hope you found this guide helpful. For further reading, explore the Spring MVC documentation, which provides detailed insights into these concepts. Happy coding!
If you have any additional insights or experiences working with DispatcherServlet, feel free to leave a comment below!