Common Spring MVC Resource Handling Issues and Solutions
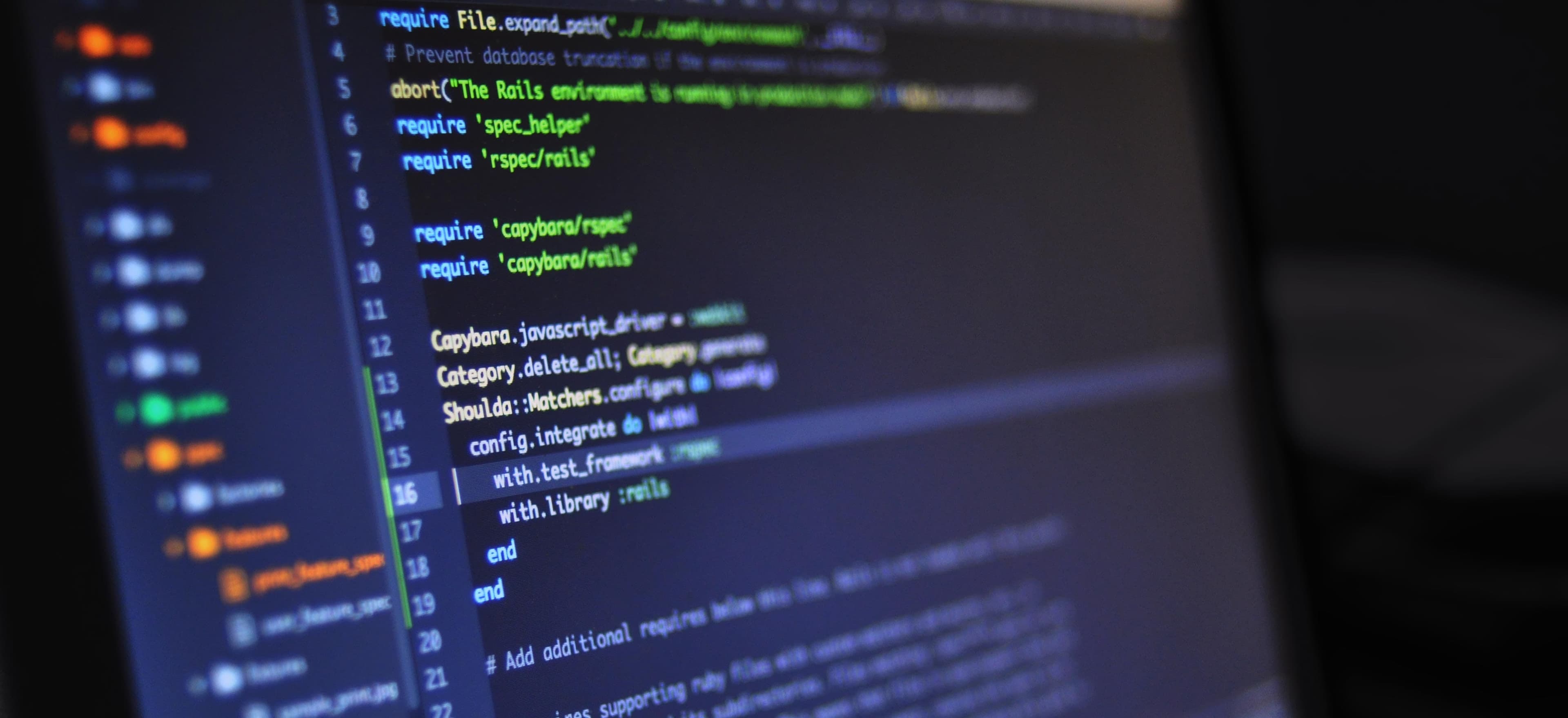
- Published on
Common Spring MVC Resource Handling Issues and Solutions
Spring MVC is a powerful framework that simplifies the building of web applications in Java. While it offers extensive capabilities for managing resources—like static files, views, and REST endpoints—developers occasionally encounter issues. In this blog post, we will explore common Spring MVC resource handling problems along with their solutions.
Understanding Resource Handling in Spring MVC
Before delving into specific issues, let’s take a moment to understand how Spring MVC handles resources. In general, resources such as CSS, JavaScript, and image files are delivered to clients in a web application. Spring MVC facilitates this through configuration settings and annotations.
Static Resource Configuration
To serve static resources, Spring MVC provides a convenient way of mapping them to specific handlers. This typical configuration is part of the WebMvcConfigurer
interface. Here's an example:
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/static/**")
.addResourceLocations("classpath:/static/");
}
}
Why It Matters
This code snippet configures the Spring application to serve resources located in src/main/resources/static
directory under the /static/
path. This approach ensures that when your browser requests http://your-domain/static/example.jpg
, Spring looks for example.jpg
in the specified location.
Common Issues and Their Solutions
Issue 1: 404 Not Found Errors for Static Resources
Problem: Users often report receiving a 404 error when trying to access static files.
Solution: Make sure your resource handlers are correctly set up. If you're serving files from the classpath, ensure they're placed in the right directory.
For example, if you're trying to access static files at /static/
but the resources are not located under src/main/resources/static
, you'll encounter a 404 error.
Debugging Steps:
-
Confirm the directory structure:
src └── main └── resources └── static ├── css │ └── styles.css ├── js │ └── script.js └── images └── logo.png
-
Ensure your
addResourceLocations
method points to the correct path.
Example:
If resources are stored in src/main/resources/public
, change your configuration like this:
registry.addResourceHandler("/static/**")
.addResourceLocations("classpath:/public/");
Issue 2: Cache Issues with Static Resources
Problem: Changes to static resources do not seem to reflect upon refreshing the browser.
Solution: Leverage cache-busting techniques. A common approach is to include a version number or build timestamp in the resource URLs. Alternatively, set the appropriate headers.
Example:
The following snippet sets a cache-control header:
import org.springframework.http.CacheControl;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import java.util.concurrent.TimeUnit;
@Controller
public class HomeController {
@GetMapping("/resource")
@ResponseBody
public String getResource() {
return "Cache-Control: public, max-age=3600";
}
}
This controller method returns cache headers that instruct the browser to cache for an hour.
Issue 3: CORS Issues with Frontend Resources
Problem: When making AJAX requests to send or fetch data, developers face Cross-Origin Resource Sharing (CORS) issues.
Solution: Enable CORS in the Spring application. This can be achieved with a simple configuration.
Example:
Add the @CrossOrigin annotation at the method or class level:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@CrossOrigin(origins = "http://your-frontend-domain.com")
public class ApiController {
@GetMapping("/api/data")
public String getData() {
return "This is data from the server.";
}
}
This allows your Spring backend to serve data to the specified frontend domain.
Issue 4: Version Management of Static Resources
Problem: Multiple versions of a resource might clash, which can be problematic when deploying updated files.
Solution: Use a versioning system for your static resources or use a build tool like Maven or Gradle to handle versioning.
Example:
For Maven, you can specify resource filtering in your pom.xml
file:
<build>
<resources>
<resource>
<directory>src/main/resources/static</directory>
<includes>
<include>**/*.css</include>
</includes>
<filtering>true</filtering>
</resource>
</resources>
</build>
Best Practices for Resource Handling in Spring MVC
- Use Resource Versioning: Always version your resources to avoid caching issues.
- Leverage Build Tools: Use tools like Maven or Gradle to simplify dependency management and version control of static resources.
- Set Proper Headers: Configure Cache-Control headers to optimize resource delivery.
- Organize Files Logically: Maintain a clear structure for where your static resources are stored and used.
- Test Extensively: Use tools like Postman to test your endpoints and ensure resources are correctly served.
For more advanced configurations related to Spring MVC and resources, you may want to check the Spring MVC Documentation and community discussions. These resources provide additional insights and community-contributed solutions to common issues.
Key Takeaways
We have covered some common resource handling issues in Spring MVC along with practical solutions. By adhering to best practices, you can create a seamless experience for users of your web application. Remember to check your configurations regularly and keep your resource management strategies in place to avoid interruptions.
Feel free to leave your comments or share your experiences below if you've encountered other peculiar issues. Your insights are valuable to fellow developers navigating the challenges of Spring MVC!
Checkout our other articles