Common JPA Query Mistakes and How to Avoid Them
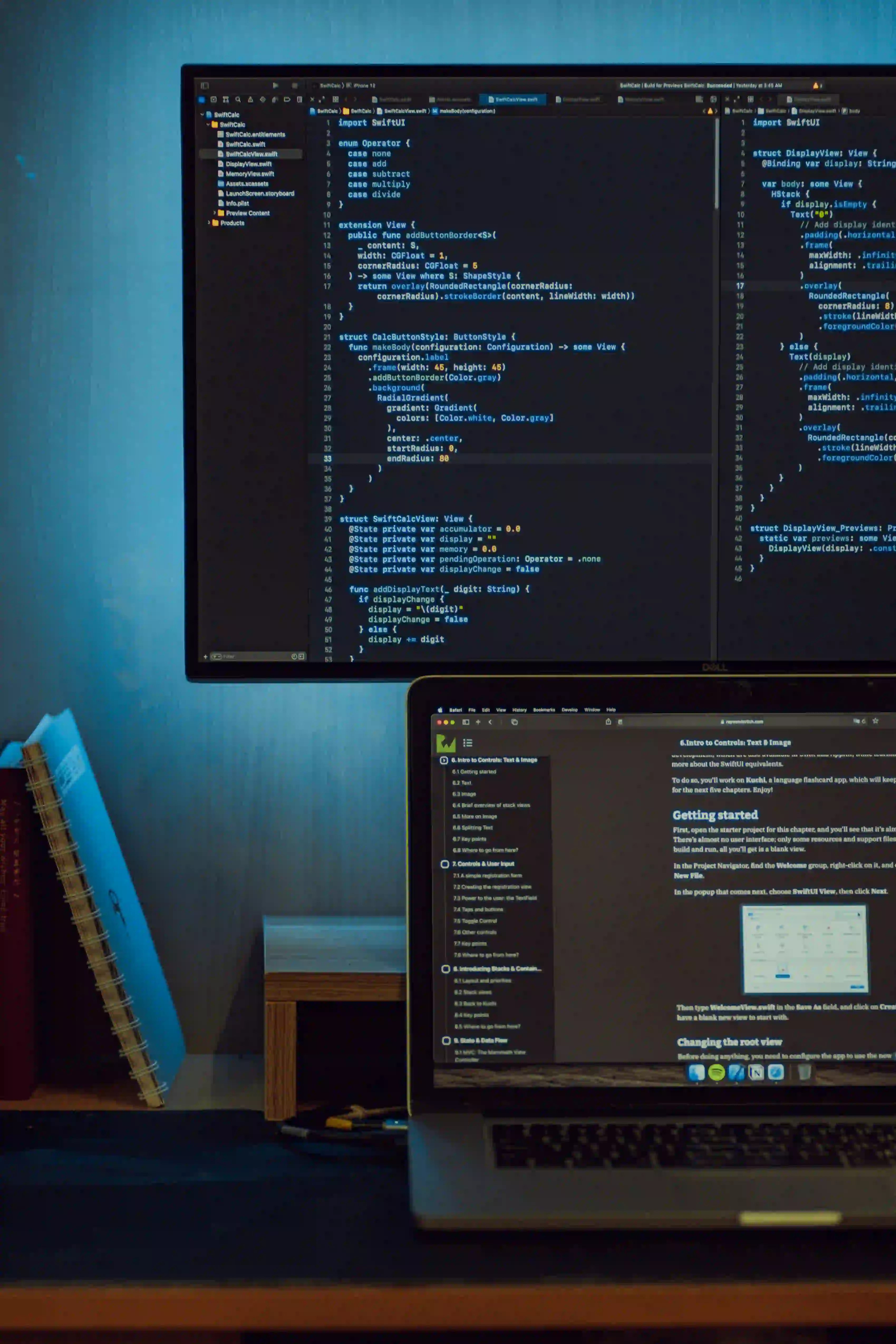
Common JPA Query Mistakes and How to Avoid Them
Java Persistence API (JPA) has greatly simplified database interactions for Java applications. However, like any powerful tool, it can lead to pitfalls if not used properly. In this blog post, we will explore the common mistakes developers make when using JPA queries, along with how to avoid them.
Understanding JPA
Before we dive into mistakes, let's briefly understand what JPA is. JPA is a Java specification for accessing, persisting, and managing data between Java objects and a relational database. It serves as a bridge between object-oriented domain models and the relational database.
Many frameworks, such as Hibernate and EclipseLink, implement JPA to provide enhanced functionality.
The Importance of Optimizing JPA Queries
Optimizing JPA queries is essential for maintaining performance, especially for applications with growing datasets. Efficient query design can significantly reduce database load and improve application responsiveness.
Common JPA Query Mistakes
1. Not Using Criteria API for Dynamic Queries
Using JPQL (Java Persistence Query Language) is often favored for its readability. However, when creating dynamic queries, developers often resort to string concatenation, leading to severe issues like SQL injection vulnerabilities and code maintainability problems.
Example of JPQL with issues:
String queryString = "SELECT e FROM Employee e WHERE e.department = '" + departmentName + "'";
TypedQuery<Employee> query = entityManager.createQuery(queryString, Employee.class);
Better Approach: Use Criteria API
By utilizing the Criteria API, you gain type safety and prevent SQL injection issues inherently.
CriteriaBuilder criteriaBuilder = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> criteriaQuery = criteriaBuilder.createQuery(Employee.class);
Root<Employee> employee = criteriaQuery.from(Employee.class);
criteriaQuery.select(employee)
.where(criteriaBuilder.equal(employee.get("department"), departmentName));
TypedQuery<Employee> query = entityManager.createQuery(criteriaQuery);
Why this Matters
The Criteria API ensures that your queries are safe and scalable. When your requirements change, modifying criteria is easier and avoids potential errors.
2. Ignoring Fetch Strategies
JPA provides two fetching strategies: EAGER and LAZY. Failing to adjust these settings based on use cases can lead to performance issues such as the N+1 query problem.
Example of Eager Fetching Mistake
// User has an EAGER relationship with Orders
User user = entityManager.find(User.class, userId);
List<Order> orders = user.getOrders(); // Potential N+1 query issue
Avoiding This Mistake
Use LAZY loading where appropriate, especially in one-to-many relationships. Adjust fetching strategies based on the specific context of your application.
@OneToMany(fetch = FetchType.LAZY)
private List<Order> orders;
Why Choosing the Right Fetch Strategy Matters
Choosing the correct strategy can dramatically influence the number of database calls made, thus optimizing performance. Understanding the data access patterns of your application helps in making educated fetch strategy choices.
3. Overusing DISTINCT
While DISTINCT
can seem like a quick fix for duplicate records, overusing it can lead to performance degradation. Since DISTINCT
forces an additional sort and check for duplicates, unnecessary use can slow down your queries.
Example
TypedQuery<User> query = entityManager.createQuery("SELECT DISTINCT u FROM User u", User.class);
Avoiding This Mistake
Always analyze whether distinct results are necessary. If you have relationships that inherently contain duplicates, consider refining your selection criteria or using GROUP BY.
Why It Matters
Performance issues can result from unnecessary data processing. Understanding your data’s uniqueness helps to refine queries effectively.
4. Not Using Pagination
Another common mistake is failing to paginate query results. Many developers overlook pagination in expectations of small datasets, only to run into performance issues when the dataset grows.
Example of a Query Without Pagination
TypedQuery<Employee> query = entityManager.createQuery("SELECT e FROM Employee e", Employee.class);
List<Employee> employees = query.getResultList(); // Not paginated
Implementing Pagination
Use the setFirstResult()
and setMaxResults()
methods for pagination.
TypedQuery<Employee> query = entityManager.createQuery("SELECT e FROM Employee e", Employee.class);
query.setFirstResult(pageNumber * pageSize);
query.setMaxResults(pageSize);
List<Employee> employees = query.getResultList();
Why Pagination Matters
It reduces memory consumption and translates to faster query execution. Always consider user experience—fetching only the required data fosters better performance.
5. Neglecting Transactions
JPA interacts with relational databases, which means all changes must be executed within a transaction. Neglecting to manage transactions can result in data inconsistency or even runtime exceptions.
Example of Transaction Management
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
try {
// Your code to persist entities
em.getTransaction().commit();
} catch (Exception e) {
if (em.getTransaction().isActive()) {
em.getTransaction().rollback();
}
// Handle exception
} finally {
em.close();
}
Why Transaction Management is Important
Managing transactions is crucial for data integrity, performance, and error handling. Always ensure that you wrap data manipulation operations in transactions to maintain consistency.
Additional Resources
To further your understanding of JPA and avoid these mistakes, consider checking out the following resources:
- Official JPA Documentation
- Hibernate Documentation
Closing the Chapter
By understanding and avoiding these common JPA query mistakes, developers can build more efficient, maintainable, and secure applications. Remember to leverage the capabilities of JPA, like the Criteria API and proper transaction management, to streamline your data access layer. Do not underestimate the importance of choosing appropriate fetching strategies and managing your data’s uniqueness.
As you continue your journey with JPA, ensure to evaluate your queries regularly, adapting and optimizing them based on the evolving needs of your application. Happy coding!