Are HATEOAS Constraints Hurting Your RESTful API Design?
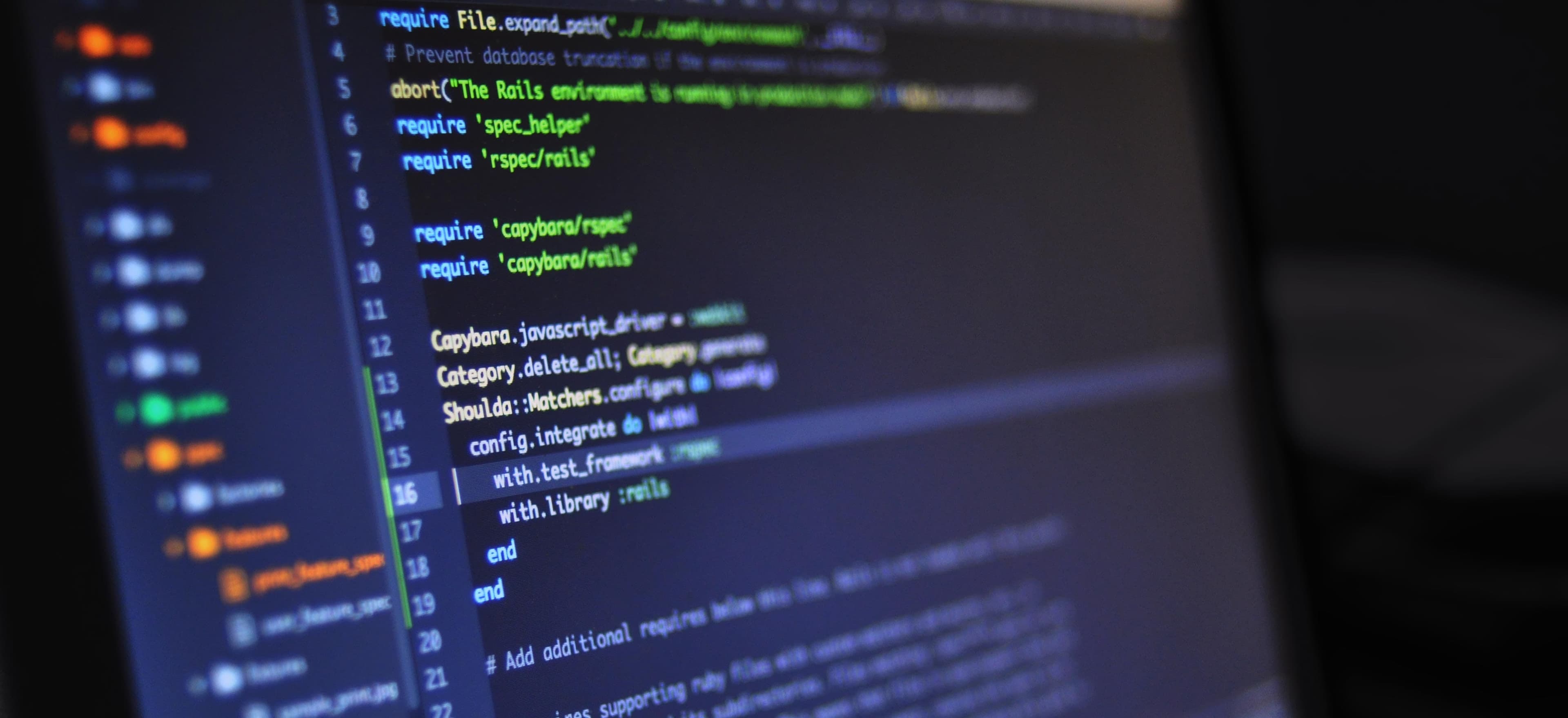
- Published on
Are HATEOAS Constraints Hurting Your RESTful API Design?
In the world of web services, RESTful APIs have become a formidable standard, embraced for their simplicity and robustness. Among the various concepts associated with REST, HATEOAS (Hypermedia as the Engine of Application State) stands out. It specifies that a client interacts with a network application entirely through hypermedia links. This concept aims to enhance the discoverability of API resources and their associated operations. However, its implementation does come with constraints that may hurt your API design.
Understanding HATEOAS
HATEOAS is a constraint of the REST application architecture. According to Roy Fielding, the creator of REST, HATEOAS allows clients to obtain the necessary information purely through hypermedia. In simpler terms, it ensures that the client doesn’t need to hardcode URLs or request paths; instead, they navigate through links provided dynamically in API responses.
Example of HATEOAS
To illustrate, consider an API response for a user resource:
{
"id": 1,
"name": "John Doe",
"links": [
{
"rel": "self",
"href": "http://api.example.com/users/1"
},
{
"rel": "posts",
"href": "http://api.example.com/users/1/posts"
},
{
"rel": "friends",
"href": "http://api.example.com/users/1/friends"
}
]
}
In the example above, the links
array provides the client with the necessary URLs to access related resources, thus following the HATEOAS principle.
Benefits of HATEOAS
Before diving into the constraints, let's explore some advantages of implementing HATEOAS:
1. Discoverable Interfaces
Clients can effortlessly discover resources and operations. When APIs evolve, changes in the links can be made without impacting clients that utilize the API.
2. Reduced Client Coupling
Clients are less coupled to a hardcoded URL structure. This leads to easier maintenance and adaptability to changing API structures.
3. Improved Navigation
Similar to web applications, clients can ‘navigate’ through the API using links, providing a more intuitive experience.
Constraints of HATEOAS
While HATEOAS has its strengths, it also introduces challenges that can hinder the efficiency and clarity of API design.
1. Increased Complexity
Implementing HATEOAS adds a layer of complexity to both the API and the client.
- API Development: Each response must be meticulously designed to include appropriate links. This requires additional planning and coding efforts.
- Client Development: Clients may struggle with parsing and following these links instead of straightforward URL paths.
2. Overhead in Client Performance
Clients must traverse links to understand resource states. This approach can lead to more HTTP requests being made:
public void fetchUserDetails() {
// Obtain user from API
User user = api.getUser();
// Fetch related resources using provided links
List<Post> userPosts = api.getPosts(user.getLink("posts").getHref());
List<User> userFriends = api.getFriends(user.getLink("friends").getHref());
}
Here, if the client is consumed with navigating multiple links, it may lead to performance degradation especially if not managed well.
3. Indirection and Usability Issues
The indirect nature of HATEOAS can lead to confusion for developers unfamiliar with navigating hypermedia. Consider a developer who has to follow complex link relations without a clear understanding of the API's structure. This can result in:
- Steep Learning Curve: New developers may find it difficult to integrate smoothly.
- Increased Development Time: More time spent understanding navigation rather than focusing on core functionalities.
4. Limitations in Tooling
There’s still a gap in tooling that fully supports HATEOAS in many programming environments. While some frameworks provide HATEOAS support, it may not always align seamlessly with your existing architecture or stacks.
Balancing HATEOAS with Simplicity
In conclusion, while HATEOAS certainly offers several benefits, the constraints it introduces may outweigh those advantages in certain scenarios. Here are some tips on integrating HATEOAS while keeping your design clear and efficient:
Assess Application Needs
Before fully adopting HATEOAS, consider how discoverable the API needs to be. If there are minimal changes anticipated in the API paths, the cost of implementing HATEOAS may not be justified.
Target Simplicity
Focus on providing essential links that genuinely drive discoverability. Avoid overwhelming clients with unnecessary data in each API response.
Consider Alternative Patterns
If you find HATEOAS may complicate things, consider using clear and documented endpoint structures. This allows for a balance between REST principles and developer usability.
Use Documentation Tools
Leverage documentation tools like Swagger or API Blueprint. These tools can serve as a guideline for users interacting with your API, offsetting some of the learnability issues posed by HATEOAS.
Final Considerations
HATEOAS has the potential to significantly enhance RESTful API interactions by making them more discoverable and flexible. However, this comes at the cost of increased complexity, potential performance drawbacks, and usability issues. Balancing the merits of HATEOAS with the principles of simplicity and clarity is key to designing a RESTful API that stands the test of time.
Ultimately, the decision to embrace HATEOAS or not should be based on the specific needs of your application and your user base. With careful consideration, you can create an API design that not only adheres to REST principles but also enhances the user experience.
Feel free to explore more interesting insights regarding RESTful APIs through these useful resources and API design guidelines.