Common Trunk-Based Development Pitfalls to Avoid
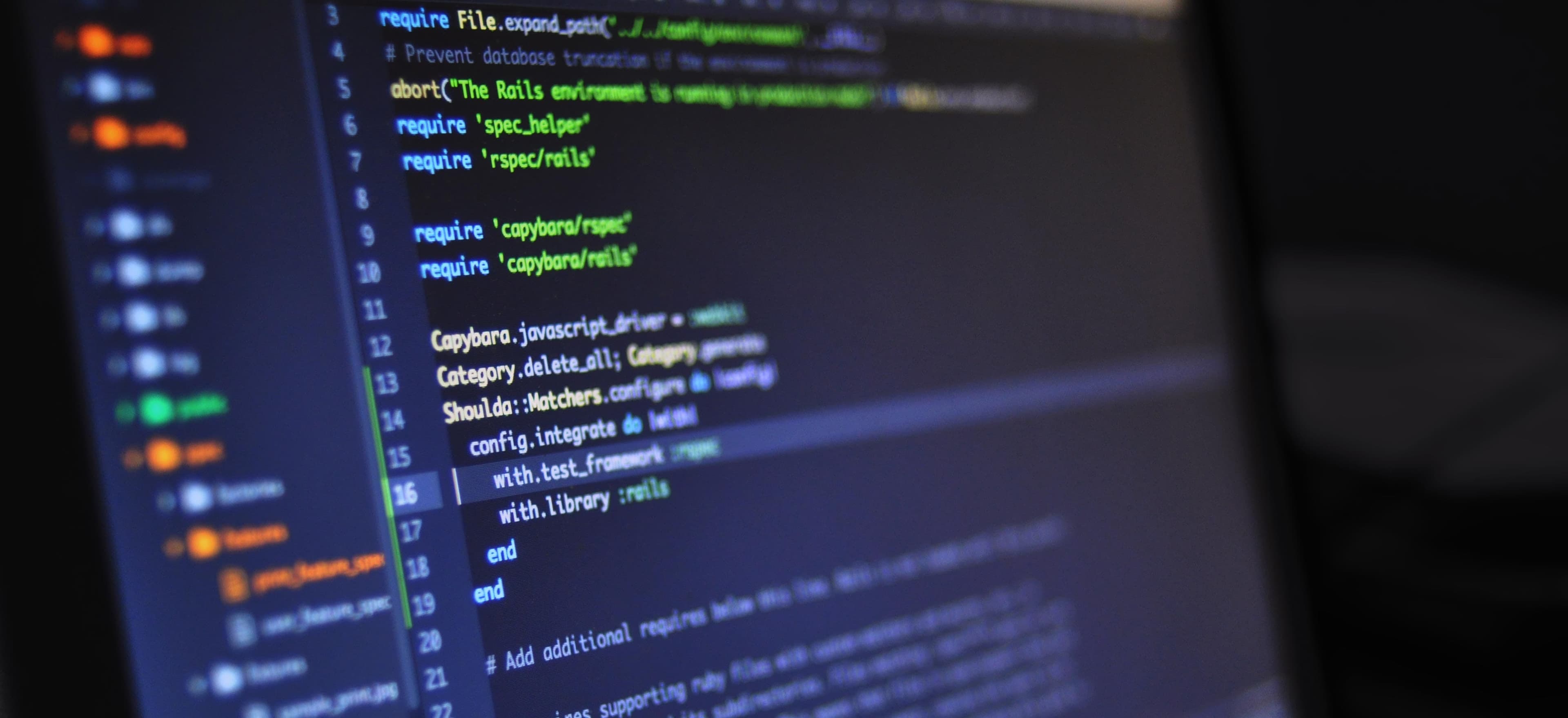
- Published on
Common Trunk-Based Development Pitfalls to Avoid
Trunk-Based Development (TBD) has gained immense traction in the modern software engineering landscape due to its ability to streamline workflows, reduce complexity, and enhance collaboration among teams. However, like any methodology, TBD is not devoid of pitfalls. This blog post discusses common mistakes developers encounter when practicing TBD and offers solutions to avoid them.
What is Trunk-Based Development?
Before delving into the pitfalls, let's clarify what Trunk-Based Development entails. In TBD, all developers work off a single branch (often called 'trunk' or 'main'), merging their changes into this main branch. This approach encourages frequent integration and minimizes the risks associated with long-lived branches.
Benefits of Trunk-Based Development
- Increased Collaboration: Since everyone is working off the same branch, developers are continuously aware of each other’s changes.
- Reduced Merge Conflicts: By integrating changes frequently, conflicts become minimal.
- Faster Feedback: Automated testing can be run immediately against the latest changes, providing developers rapid feedback.
Common Pitfalls of Trunk-Based Development
Let's shine a spotlight on common pitfalls associated with Trunk-Based Development, as well as how to steer clear of them.
1. Neglecting Automation
Problem: One of the most significant pitfalls is the absence of automated testing and Continuous Integration (CI). When teams do not implement automated tests, they risk deploying unverified changes.
Solution: Invest time in setting up a robust CI pipeline. Automate testing so that every commit triggers builds and tests. This ensures that the code in the trunk is always in a deployable state.
// Sample CI configuration snippet using Maven
<project>
...
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>2.22.2</version>
<configuration>
<includes>
<include>**/*Test.java</include>
</includes>
</configuration>
</plugin>
</plugins>
</build>
</project>
Why? This snippet configures the Maven Surefire plugin to run all test cases during the build process, ensuring that any introduction of breaking changes is caught early.
2. Introducing Long-Lived Feature Branches
Problem: Developers may be tempted to create long-lived branches to work on features that take time to complete. This can lead to integration hell, where merging becomes complex and frustrating.
Solution: Aim for short-lived feature toggles. Keep features small and continuously integrate them into the trunk. Use feature flags to control the rollout of incomplete features without destabilizing the trunk.
// Example of a feature toggle
if (FeatureToggle.isNewFeatureEnabled()) {
executeNewFeature();
} else {
executeOldFeature();
}
Why? Using feature toggles allows developers to integrate their work frequently. It reduces the risk of complex merges and keeps the codebase current.
3. Poor Communication
Problem: Trunk-Based Development relies heavily on team collaboration. A lack of communication can lead to misunderstandings, duplicated work, or overwriting each other's changes.
Solution: Encourage regular stand-ups and team syncs to discuss changes and development priorities. Utilize communication tools like Slack or Microsoft Teams effectively.
4. Insufficient Code Reviews
Problem: Regardless of the integration strategy, code reviews are essential for maintaining code quality. In TBD, however, the urgency to merge can lead to oversight.
Solution: Establish a rigorous code review process. Tools such as GitHub or Bitbucket can facilitate this by allowing team members to comment on and approve code changes before they are merged.
// Example pull request title
Fix: Address null pointer exception in UserLoginService
Why? By using clear and conventional pull request titles, developers signal what changes have been made and why, aiding in the review process.
5. Ignoring Version Control Best Practices
Problem: Developers may overlook basic Git commands and best practices, leading to a chaotic codebase. Simple mistakes can cause major setbacks.
Solution: Train teams in Git best practices. Encourage the use of descriptive commit messages and require pull requests before merging.
# Good commit message format
git commit -m "Fix: correct user input validation in RegistrationService"
Why? Clear commit messages help track changes and facilitate easier understanding of project history for team members.
6. Underestimating the Importance of Refactoring
Problem: As codebases evolve, the necessity for refactoring grows. Some teams may rush to add features without addressing technical debt, affecting overall code health.
Solution: Schedule regular refactoring sessions or allocate time in sprints specifically for improving existing code. This practice keeps the codebase clean and maintainable.
7. Skipping Documentation
Problem: Team members might assume everyone is on the same page and skip documentation, which can lead to confusion, especially for new developers.
Solution: Encourage teams to document their processes, especially when changes are made to critical components. Confluence or Notion can be useful platforms for sharing documentation.
8. Lack of Continuous Deployment (CD)
Problem: Just because development is continuous doesn’t mean deployment has to be. Introducing a manual deployment process can counteract the agility offered by TBD.
Solution: Establish a Continuous Deployment pipeline to ensure that every change that passes testing is automatically deployed to production. This ensures that feedback loops remain tight and teams can respond quickly to issues.
# Example deployment configuration for AWS Elastic Beanstalk
Resources:
MyApplication:
Type: AWS::ElasticBeanstalk::Application
Properties:
ApplicationName: !Ref AppName
Description: Application for managing user data.
Why? Automating deployment minimizes the lead time to get new features into the hands of users, fostering a culture of rapid iteration and improvement.
In Conclusion, Here is What Matters
Trunk-Based Development provides numerous benefits for teams willing to embrace it, but it comes with its own set of challenges. Keeping the above pitfalls in mind and proactively addressing them can facilitate a smoother, more efficient development process.
For more information about effective strategies in software development, you may want to explore these best practices of Agile Development and Continuous Integration strategies.
By recognizing common pitfalls and adopting solutions, development teams can maximize the advantages of TBD while minimizing its risks. Happy coding!