Top JMS Interview Questions You Need to Ace in 2023
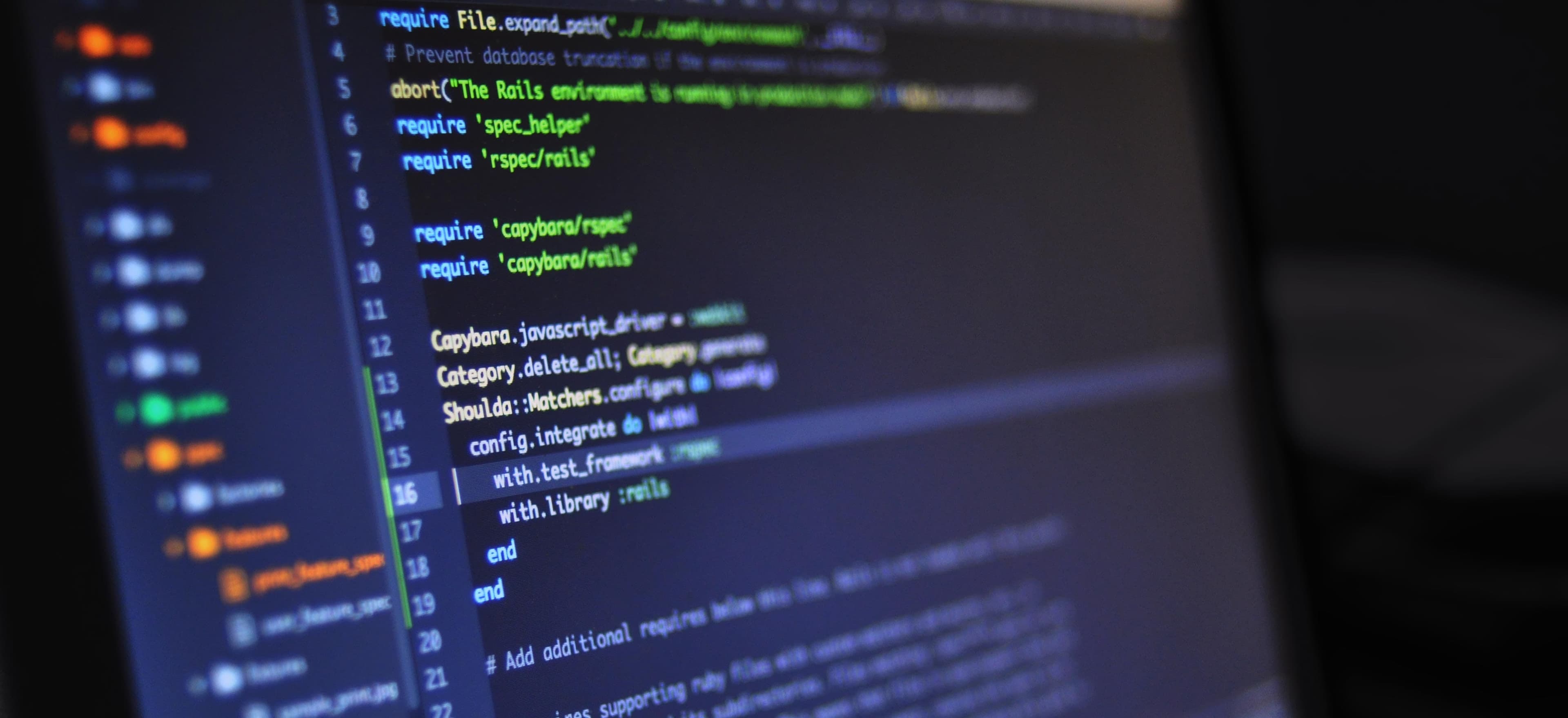
- Published on
Top JMS Interview Questions You Need to Ace in 2023
Java Message Service (JMS) is an essential API in Java for sending messages between two or more clients. As enterprise applications grow, the need for robust messaging systems increases. If you're preparing for a Java-related position in 2023, especially one involving enterprise-level applications, a solid understanding of JMS is crucial.
In this blog post, we'll cover the top JMS interview questions you need to ace. We’ll delve into the concepts, provide code snippets, and elucidate the practical implications of each topic. Let’s dive in!
What is JMS?
Java Message Service (JMS) is a messaging standard that allows application components to create, send, receive, and read messages. It is part of the Java EE (Enterprise Edition) platform and facilitates communication between different components of a distributed application.
Key JMS Concepts
-
Messaging Models:
- Point-to-Point (PTP): In this model, a message is sent from one producer to one consumer through a queue.
- Publish/Subscribe: In this model, messages are sent from one producer to multiple consumers through topics.
-
JMS Providers: These are messaging systems like ActiveMQ, RabbitMQ, and IBM MQ that implement the JMS API.
Why Learn JMS?
Understanding JMS is crucial for any Java developer working with distributed systems. It ensures that applications can communicate reliable and asynchronously, decoupling the sender and receiver.
Common JMS Interview Questions
1. What are the key components of JMS?
Answer: The primary components in a JMS architecture include:
- JMS Provider: The messaging system that implements the JMS API.
- JMS Clients: The applications that produce and consume messages.
- Messages: The data exchanged between clients, which can be of various types (TextMessage, ObjectMessage, etc.).
- Destinations: Queues or topics where messages are sent.
2. What is the difference between a Queue and a Topic?
Answer:
-
Queue: In a point-to-point model, a message sent to a queue is consumed by one and only one consumer. This is useful for tasks like load balancing.
-
Topic: In a publish-subscribe model, a message sent to a topic can be consumed by multiple subscribers. This is suitable for scenarios where multiple clients need to receive the same message.
3. Can you explain the difference between synchronous and asynchronous communication?
Answer:
-
Synchronous communication: The sender waits for the receiver to process the message before continuing. This can create bottlenecks.
-
Asynchronous communication: The sender sends the message and continues processing without waiting for a response. This is more efficient and scalable.
4. Write a simple JMS producer and consumer in Java.
Here’s a basic example of a JMS producer that sends a message to a queue:
import javax.jms.*;
import javax.naming.InitialContext;
import javax.naming.NamingException;
public class JMSProducer {
public static void main(String[] args) {
Connection connection = null;
try {
InitialContext ctx = new InitialContext();
ConnectionFactory factory = (ConnectionFactory) ctx.lookup("jms/MyConnectionFactory");
connection = factory.createConnection();
connection.start();
Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = session.createQueue("MyQueue");
MessageProducer producer = session.createProducer(queue);
TextMessage message = session.createTextMessage("Hello JMS!");
producer.send(message);
System.out.println("Message sent: " + message.getText());
} catch (JMSException | NamingException e) {
e.printStackTrace();
} finally {
if (connection != null) {
try {
connection.close();
} catch (JMSException e) {
e.printStackTrace();
}
}
}
}
}
Commentary:
- We start the connection with the JMS provider and establish a session.
- The
MessageProducer
is created for the specified queue. - A
TextMessage
is constructed and sent. Using auto acknowledgment simplifies the message handling.
5. Explain message acknowledgment modes.
Answer: JMS provides several acknowledgment modes to ensure messages are processed:
-
AUTO_ACKNOWLEDGE: The session automatically acknowledges the receipt of messages. This is simple but can be risky if a message fails during processing.
-
CLIENT_ACKNOWLEDGE: The client acknowledges a message's receipt explicitly. It allows more control but requires careful handling.
-
DUPS_OK_ACKNOWLEDGE: The implementation allows duplicate messages but guarantees delivery.
-
SESSION_TRANSACTED: All messages sent/received in the session are committed or rolled back as a unit. This is useful for ensuring atomicity.
6. How can you ensure message durability in JMS?
Answer: To ensure message durability:
-
Use persistent messages: When sending a message, set its delivery mode to DeliveryMode.PERSISTENT. This guarantees that messages are not lost even if the messaging provider crashes.
-
Use a reliable JMS provider: Providers like Apache ActiveMQ and IBM MQ are designed for high durability.
Example code for sending a persistent message:
producer.send(message, DeliveryMode.PERSISTENT, Message.DEFAULT_PRIORITY, Message.DEFAULT_TIME_TO_LIVE);
7. What are JMS message types?
Answer: JMS has several types of messages:
- TextMessage: A message that contains a string.
- ObjectMessage: A message that contains a serializable Java object.
- MapMessage: A message that contains a set of name-value pairs.
- BytesMessage: A message that contains an array of bytes.
- StreamMessage: A message that allows sending a stream of primitive types.
8. What is Message Selector in JMS?
Answer: A message selector is a SQL-like syntax used to filter messages. This allows consumers to receive only specific messages from a topic or queue based on certain criteria.
Example:
String selector = "color = 'red'";
MessageConsumer consumer = session.createConsumer(queue, selector);
This way, only messages with the property color
set to red
will be consumed.
9. What is the role of JNDI in JMS?
Answer: Java Naming and Directory Interface (JNDI) is used for looking up JMS resources like connection factories and destinations. This decouples the application from the specifics of the JMS provider.
10. How do you handle exceptions in JMS?
Answer: Since JMS is asynchronous, it’s essential to manage exceptions gracefully. Use try-catch blocks around JMS operations and consider implementing the following strategies:
- Connection Handling: Re-establish connections in case of loss.
- Retries: Implement a retry mechanism for transient failures.
- Dead Letter Queue (DLQ): Unsuccessful messages can be routed to a DLQ for further examination.
Wrapping Up
In 2023, mastering JMS is indispensable for a successful career in Java enterprise development. From understanding the basic concepts to handling exceptions, each aspect of JMS plays a pivotal role in creating reliable and responsive applications.
We encourage you to explore further on Oracle's JMS Documentation for a deeper understanding and practical implementations. With these essentials in mind, you are now equipped with the foundational knowledge to navigate JMS interviews confidently. Good luck!
Checkout our other articles