Mastering Retry Logic: Avoiding Infinite Loops in Spring Retry
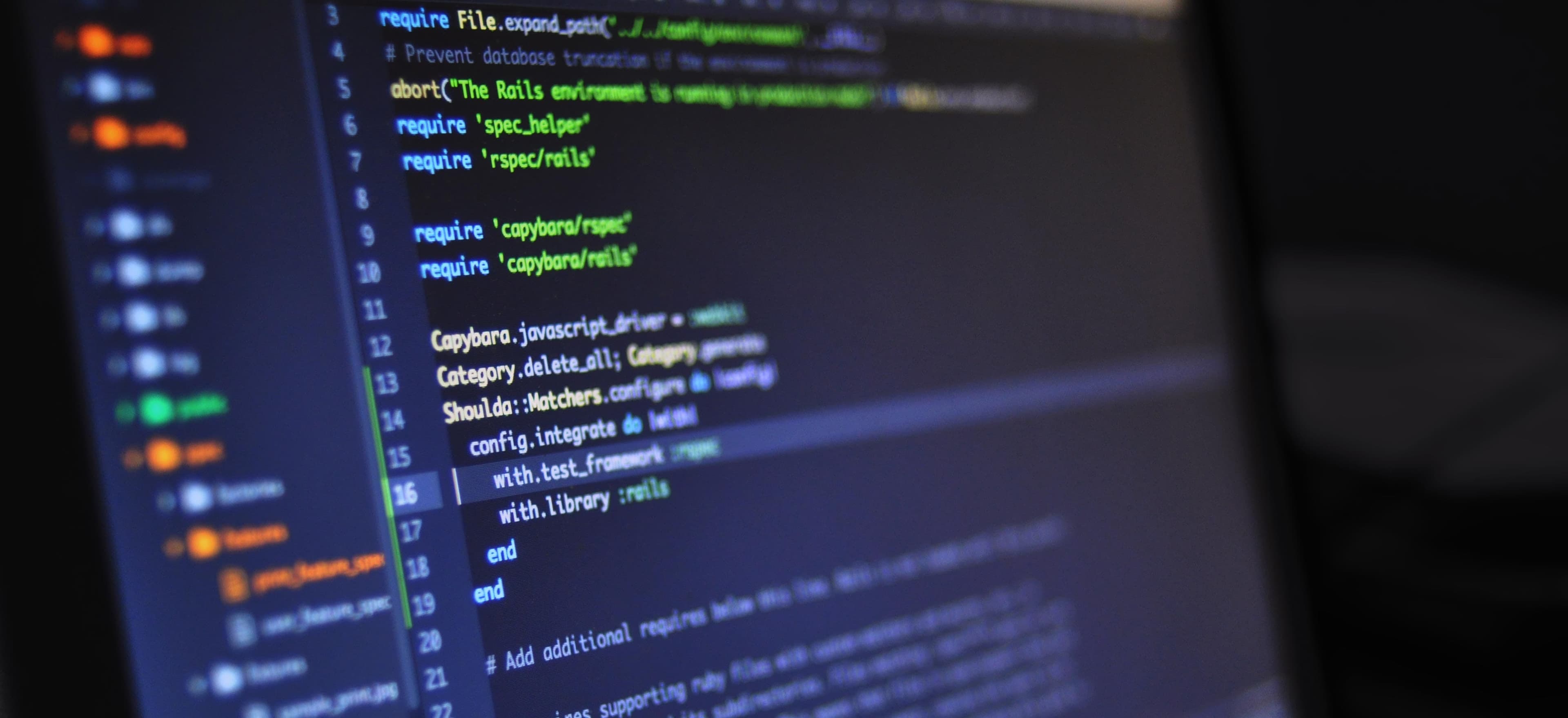
- Published on
Mastering Retry Logic: Avoiding Infinite Loops in Spring Retry
In modern software applications, resilience is crucial. Whether you’re dealing with unpredictable network calls or transient data storage issues, implementing proper retry logic can help your application maintain functionality during adverse conditions. One of the tools available for managing retry logic in Java is the Spring Retry mechanism. However, improper use of this feature may lead to infinite loops, which can cause application failure instead of recovery. In this blog post, we will dive into how to effectively implement retry logic using Spring Retry while avoiding infinite loops.
What is Spring Retry?
Spring Retry is a component of the Spring Framework that provides retry capabilities for operations using declarative annotations or programmatic API. This is particularly useful when working with remote services that may experience occasional failures.
You can read more about Spring Retry here.
Why Use Retry Logic?
Retry logic serves several important purposes:
- Resilience: It allows your application to recover from transient failures without shutting down.
- Improved User Experience: End users are less likely to encounter errors, as the application retries operations automatically.
- Backoff Strategies: Control over how long to wait before retrying, allowing external services time to recover.
Setting Up Spring Retry
To get started, you will need to add the Spring Retry dependency to your project. For Maven, include the following in your pom.xml
:
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
<version>1.3.1</version>
</dependency>
For Gradle, add:
implementation 'org.springframework.retry:spring-retry:1.3.1'
Make sure to also configure your Spring application to enable retry support:
import org.springframework.context.annotation.Configuration;
import org.springframework.retry.annotation.EnableRetry;
@Configuration
@EnableRetry
public class AppConfig {
}
This annotation will enable Spring Retry within your application context.
Basic Retry Logic Implementation
Once you have set up your dependencies, you can use annotations to easily implement retry logic:
import org.springframework.retry.annotation.Backoff;
import org.springframework.retry.annotation.Retryable;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Retryable(
value = {RemoteServiceException.class},
maxAttempts = 3,
backoff = @Backoff(delay = 1000))
public String callRemoteService() {
// Call a remote service that may fail
System.out.println("Calling remote service");
if (Math.random() < 0.7) {
throw new RemoteServiceException("Service Unavailable");
}
return "Success";
}
}
Why Use Annotations?
Using annotations allows for clean, declarative code. Our @Retryable
annotation specifies:
value
: the type of exceptions to retry on.maxAttempts
: the maximum number of times to retry.backoff
: the delay between retries.
This implementation will try to execute callRemoteService()
three times, waiting one second between attempts if a RemoteServiceException
occurs.
Be Cautious: Avoiding Infinite Loops
While easy to implement, retry logic can lead to infinite loops if not carefully designed. Here are some common pitfalls to avoid:
- Unspecific Exception Handling: If you retry on a general
Exception
, you risk entering an infinite loop if the cause is not transient. - Too High
maxAttempts
Value: Setting this value too high can prolong the issue and exhaust resources. - Unreliable Termination Conditions: Make sure you have appropriate conditions under which retries should stop.
Example: Safeguarding Against Infinite Loops
Let’s explore how we can safeguard against infinite loops with specific exception handling and appropriate termination conditions:
import org.springframework.retry.annotation.Backoff;
import org.springframework.retry.annotation.Retryable;
import org.springframework.retry.annotation.Recover;
import org.springframework.stereotype.Service;
@Service
public class MyAdvancedService {
@Retryable(
value = {TransientApiException.class},
maxAttempts = 3,
backoff = @Backoff(delay = 1000))
public String callTransientService() {
// Adding an intentional failure with probability
if (Math.random() < 0.8) {
throw new TransientApiException("Temporary issue, retrying...");
}
return "Call succeeded!";
}
@Recover
public String recover(TransientApiException e) {
// Handles the case when retries are exhausted
System.out.println("Max attempts reached. Handling failure.");
return "Fallback response due to: " + e.getMessage();
}
}
Explanation of the Code
- Custom Exception: The use of
TransientApiException
allows fine-grained control over what exceptions trigger a retry. - Recover Method: The
@Recover
annotation specifies a fallback method. If all retries fail, the logic in this method will execute, thus preventing the loop.
With this setup, if the error in callTransientService
persists after three attempts, the application won’t keep retrying indefinitely. Instead, it will execute the recovery logic.
A Final Look: Best Practices for Retry Logic
Implementing retry logic using Spring Retry can greatly enhance the resilience of your applications. However, it’s essential to:
- Use Specific Exceptions: General exception handling can lead to undesired behavior.
- Set Reasonable Limits: Be cautious with
maxAttempts
and consider the implications of resource consumption. - Implement Recovery Logic: Always provide a recovery method that executes after retry attempts are exhausted.
By adhering to these best practices, you can provide a robust experience for your users and avoid pitfalls that can lead to infinite loops in your applications.
For more detailed insights, you might want to check the Spring documentation on Retry.
Now that you're armed with knowledge about Spring Retry, go ahead and integrate it into your applications for enhanced resilience! Happy coding!
Checkout our other articles