Overcoming Java's Limitations in Machine Learning Libraries
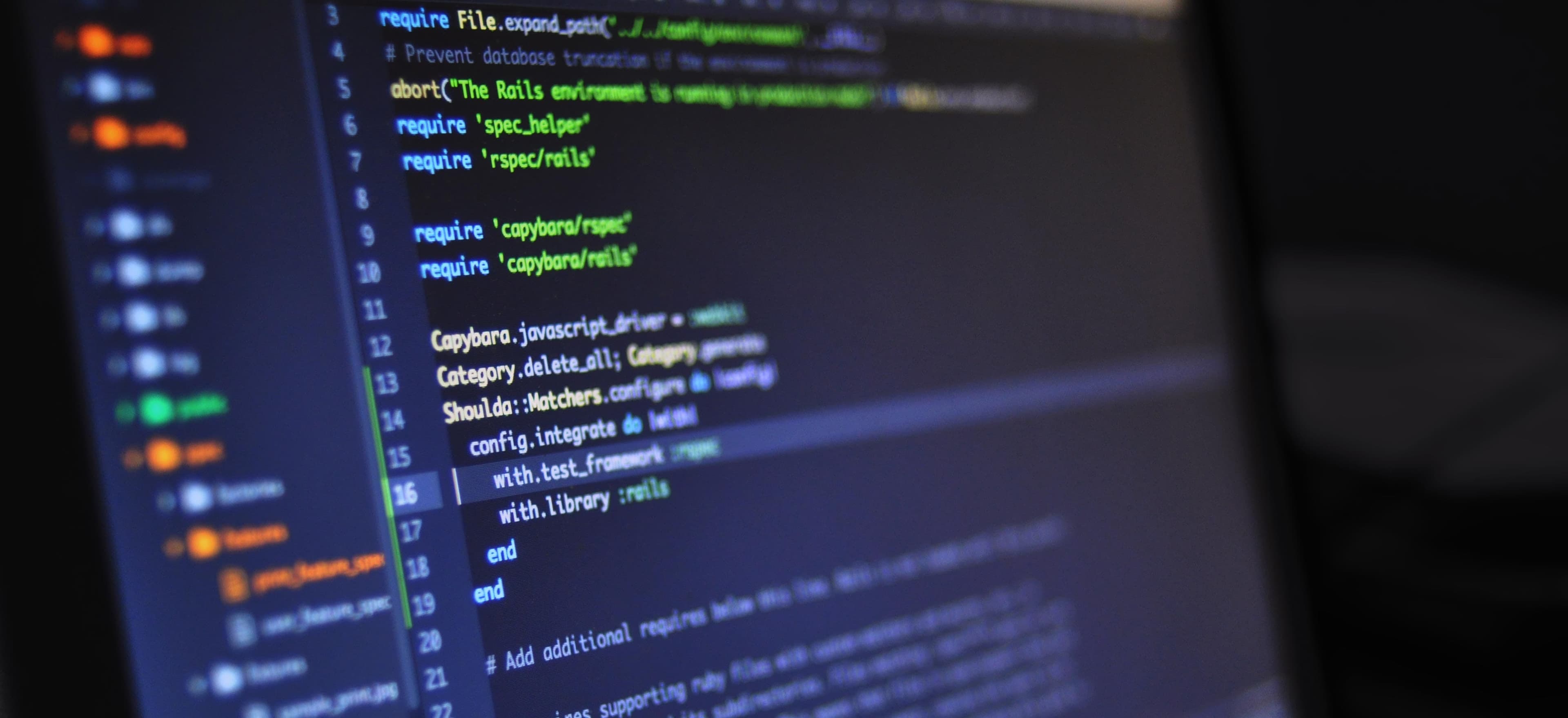
- Published on
Overcoming Java's Limitations in Machine Learning Libraries
Java has long been a powerhouse in the realm of software development. However, when it comes to machine learning, the landscape is markedly different. While languages like Python dominate due to their extensive libraries and community support, Java still holds its ground in enterprise solutions. In this blog post, we will explore how to overcome Java's limitations in machine learning, empowering developers to leverage its robust capabilities while navigating its constraints.
Understanding Java’s Limitations in Machine Learning
Before diving into solutions, it’s crucial to understand the limitations we are attempting to overcome. Here are some of the prominent challenges:
-
Limited Libraries: While there are machine learning libraries available in Java, they are not as extensive or user-friendly as those available for Python. Libraries like Weka, Deeplearning4j, and MOA exist but may not cover the latest advances found in Python libraries like TensorFlow or PyTorch.
-
Syntax Complexity: Java’s verbose syntax can lead to longer code, which may hinder and confuse rapid prototyping, a critical component of machine learning.
-
Performance Issues: Although Java is performance-oriented, dynamic languages often outperform it in tasks that require quick adjustments and iterations in models due to their flexible structure.
-
Community Support: Community-driven development is vital for innovation in machine learning. The Python ecosystem benefits from broader community support and frequent contributions to its libraries.
With these challenges identified, let's explore how you can work around them.
1. Leveraging Java-based Machine Learning Libraries
One of the more straightforward approaches to overcoming Java's limitations is using Java-based machine learning libraries. Deeplearning4j, for instance, brings powerful tools directly into Java applications.
Deeplearning4j: A Java Perspective
Deeplearning4j (DL4J) is a widely-adopted deep learning framework for the JVM. It integrates with Hadoop and Spark for big data processing, making it ideal for enterprise environments.
Code Example: Building a Neural Network
Here’s an example of how to build a simple neural network using Deeplearning4j.
import org.deeplearning4j.nn.conf.MultiLayerConfiguration;
import org.deeplearning4j.nn.conf.NeuralNetConfiguration;
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork;
import org.nd4j.linalg.activations.Activation;
import org.nd4j.linalg.learning.config.Adam;
import org.nd4j.linalg.dataset.api.iterator.DataSetIterator;
public class SimpleNeuralNetwork {
public static void main(String[] args) {
MultiLayerConfiguration configuration = new NeuralNetConfiguration.Builder()
.updater(new Adam(0.01)) // Optimizer
.list() // Add layers sequentially
.layer(0, new DenseLayer.Builder()
.nIn(784) // Input size (e.g., for MNIST)
.nOut(100) // Number of neurons in this layer
.activation(Activation.RELU) // Activation function
.build())
.layer(1, new OutputLayer.Builder()
.nIn(100)
.nOut(10) // Output size (e.g., digits 0-9)
.activation(Activation.SOFTMAX) // Softmax for classification
.build())
.build();
MultiLayerNetwork model = new MultiLayerNetwork(configuration);
model.init();
// Placeholder for data loading code.
DataSetIterator iterator = ...; // Load your training dataset
model.fit(iterator); // Train the model
}
}
Commentary
In this code snippet, we define a simple neural network configuration:
- Updaters like the Adam optimizer allow for efficient training.
- DenseLayer is used to build hidden layers, where
nIn
defines input size andnOut
defines how many neurons will be in that layer. - Activation functions like ReLU and Softmax help introduce non-linearity and aid in multi-class classification, respectively.
Utilizing libraries like DL4J allows you to tap into advanced neural network features while working in Java's robust ecosystem.
2. Incorporating Other Languages with Java
Another effective method is to leverage other languages' strengths while still operating within a Java environment. This approach can be particularly useful when you need functionality not present in Java.
Using Jython for Python Integration
Jython is an implementation of Python that runs on the Java platform. With Jython, you can utilize Python libraries seamlessly.
Code Example: Utilizing numpy with Jython
import org.python.util.PythonInterpreter;
public class JythonExample {
public static void main(String[] args) {
try (PythonInterpreter pyInterp = new PythonInterpreter()) {
pyInterp.exec("import numpy as np");
pyInterp.exec("a = np.array([1, 2, 3])");
pyInterp.exec("print(a + 1)"); // Output: [2 3 4]
}
}
}
Commentary
This example demonstrates how to execute Python code within Java. It allows for straightforward access to numpy, a popular Python library for numerical computations. The ability to use Python’s rich ecosystem significantly enhances Java’s capabilities in machine learning.
Jython expands Java's boundaries and offers a means to utilize robust libraries without losing Java's advantages.
3. Utilizing Apache Spark for Scalability
Apache Spark is a powerful tool for distributed computing and can bring significant benefits to your Java applications. It provides an API for Java to handle big data operations while integrating with machine learning libraries.
Code Example: Machine Learning with Spark in Java
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.ml.classification.LogisticRegression;
import org.apache.spark.sql.SparkSession;
public class SparkMLExample {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("Java Spark ML Example")
.getOrCreate();
// Load training data
Dataset<Row> training = spark.read().format("libsvm") // Load dataset in LibSVM format
.load("data/mllib/sample_libsvm_data.txt");
// Create Logistic Regression Model
LogisticRegression lr = new LogisticRegression();
LogisticRegressionModel model = lr.fit(training); // Model fitting
spark.stop();
}
}
Commentary
In this example, we demonstrate how to use Apache Spark for modeling:
- Logistic Regression is selected as a model here, a foundation technique in machine learning.
- By leveraging Spark, you can handle larger datasets without performance degradation.
Harnessing Apache Spark not only elevates Java's capabilities in machine learning but also ensures scaling through a distributed framework.
To Wrap Things Up
While Java may have its limitations in the machine learning sphere, it has the potential to rise above these challenges through strategic use of existing libraries, interoperability with other languages, and the power of distributed frameworks like Apache Spark.
By leveraging options such as Deeplearning4j, Jython, and Apache Spark, you can create robust machine learning frameworks that play well within the Java ecosystem.
The future of machine learning is expansive and exciting, and Java developers have the tools at their disposal to engage thoroughly with it.
For further reading, check out Deeplearning4j documentation and Apache Spark's official website to deepen your knowledge and explorations in this space.