Mastering the Visitor Pattern: Common Implementation Pitfalls
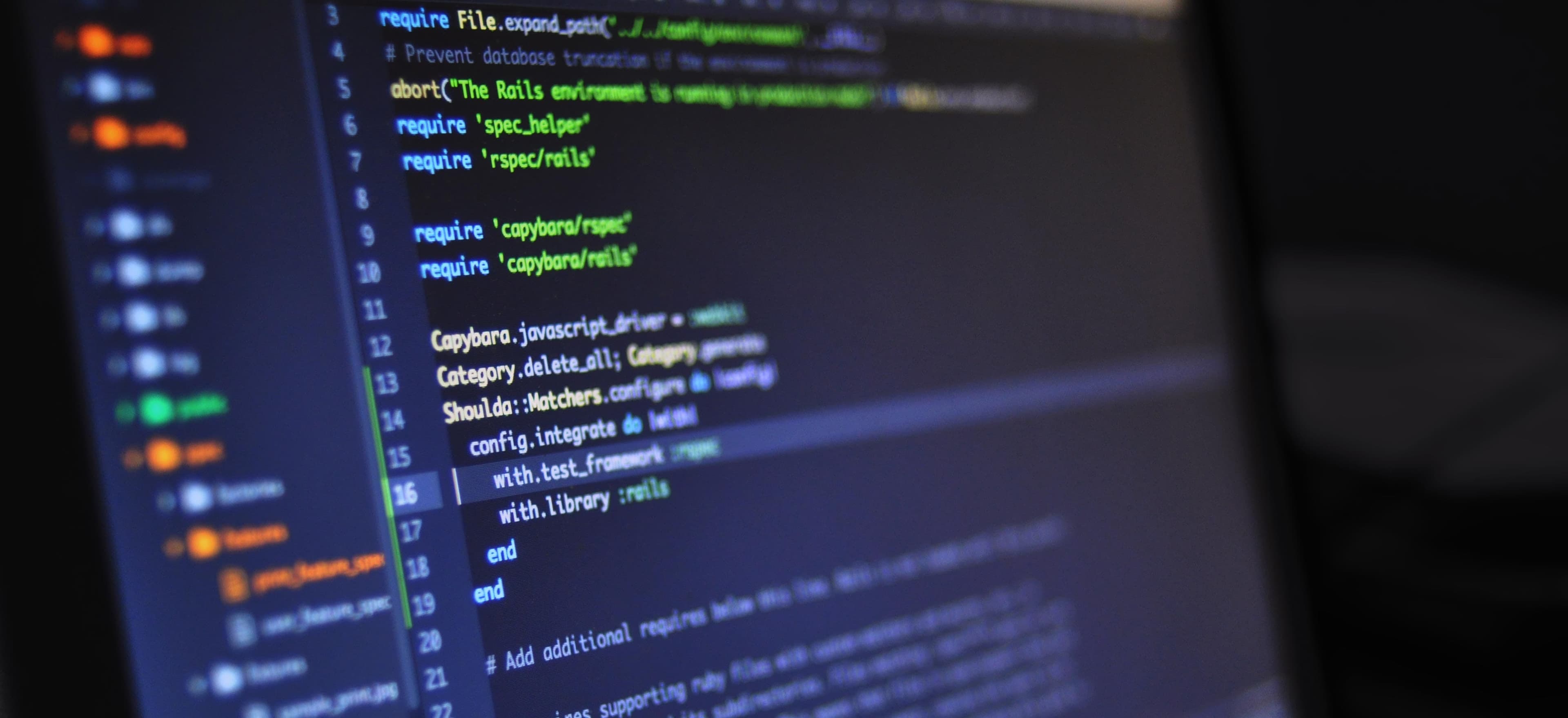
- Published on
Mastering the Visitor Pattern: Common Implementation Pitfalls
The Starting Line
In the realm of software design patterns, the Visitor Pattern stands as a powerful tool in the software architect's toolbox. It allows separation of an algorithm from the properties of the objects on which it operates. This pattern is particularly useful when you have a complex object structure and need to perform operations on these objects without modifying their classes.
However, the Visitor Pattern can also lead to pitfalls if not implemented correctly. This blog post will delve into the Visitor Pattern, discuss its benefits, and highlight common implementation mistakes, giving you a comprehensive understanding that will help you master this pattern.
Understanding the Visitor Pattern
The Visitor Pattern involves two key components:
- Visitor Interface: Defines the operations to be performed on the elements of the object structure.
- Element Interface: Each element declares an
accept
method that takes a visitor as an argument.
The essence of the Visitor Pattern is encapsulated in the following UML diagram:
+----------------+
| Visitor |
+----------------+
| +visit(Element)|
+----------------+
+------------------------+
| Element |
+------------------------+
| +accept(Visitor) |
+------------------------+
+------------------------+
| ConcreteElementA |
+------------------------+
| +accept(Visitor) |
+------------------------+
+------------------------+
| ConcreteElementB |
+------------------------+
| +accept(Visitor) |
+------------------------+
The concrete elements implement the accept
method, allowing visitors to access their data.
Implementing the Visitor Pattern
Let's take a look at a simple implementation of the Visitor Pattern in Java.
Step 1: Define the Visitor Interface
// Visitor.java
public interface Visitor {
void visit(ConcreteElementA elementA);
void visit(ConcreteElementB elementB);
}
Why: This interface allows the visitor to implement different operations on different concrete elements.
Step 2: Define the Element Interface
// Element.java
public interface Element {
void accept(Visitor visitor);
}
Why: The accept
method provides a way for the visitor to interact with the element.
Step 3: Implement Concrete Elements
// ConcreteElementA.java
public class ConcreteElementA implements Element {
private String data = "Data from Element A";
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
public String getData() {
return data;
}
}
// ConcreteElementB.java
public class ConcreteElementB implements Element {
private String data = "Data from Element B";
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
public String getData() {
return data;
}
}
Why: Each concrete element implements the accept
method, allowing the visitor to perform operations based on the type of element.
Step 4: Create Concrete Visitors
// ConcreteVisitor.java
public class ConcreteVisitor implements Visitor {
@Override
public void visit(ConcreteElementA elementA) {
System.out.println("Visiting " + elementA.getData());
}
@Override
public void visit(ConcreteElementB elementB) {
System.out.println("Visiting " + elementB.getData());
}
}
Why: The visitor implementation holds the logic for operations on each concrete element.
Step 5: Putting It All Together
// Client.java
import java.util.ArrayList;
import java.util.List;
public class Client {
public static void main(String[] args) {
List<Element> elements = new ArrayList<>();
elements.add(new ConcreteElementA());
elements.add(new ConcreteElementB());
Visitor visitor = new ConcreteVisitor();
for (Element element : elements) {
element.accept(visitor);
}
}
}
Why: The client creates a list of elements and uses the visitor to perform operations, showcasing the pattern's flexibility.
Common Implementation Pitfalls
While the Visitor Pattern can be quite effective, developers often encounter pitfalls that can ruin its advantages. Let's explore common mistakes:
1. Lack of Element Extensibility
One of the main benefits of the Visitor Pattern is that adding new operations is straightforward. However, if you frequently need to add new elements as well, you’ll find that the Visitor Pattern becomes rather cumbersome. Every time you add new elements, you must update every visitor interface.
Solution: Ensure a thorough understanding of the potential growth of your object structure. If frequent additions are anticipated, consider whether the Visitor Pattern is the right fit.
2. Force Fit Applications
Developers sometimes try to apply the Visitor Pattern in situations where it doesn't make sense, confusing it with simpler patterns or even object-oriented programming practices.
Solution: Assess the complexity and whether your object structure benefits from the separation of concerns that the Visitor Pattern provides. If there are only a few operations or elements, simpler designs may be more efficient.
3. Visitor Method Overload
When implementing a visitor, it might be tempting to add multiple visit
methods for a single element type to manage variations. However, overloading could lead to confusion about which method to call.
Solution: Keep the visitor methods minimal and specific to each element type. This approach maintains clarity and helps ensure that other developers can easily grasp the code’s intention.
4. Complicated Logic in Visitors
Another common issue is including too much logic within visitors. While visitors should encapsulate the operations on elements, they should not become overloaded classes managing complex logic or states.
Solution: Delegate complex operations or state management to separate classes or utility methods. This keeps the visitor clean and focused, increasing modularity.
5. Performance Considerations
As the number of elements and visitor operations grows, the Visitor Pattern can lead to reduced performance, especially with deep object hierarchies and complex relationships.
Solution: Monitor performance and make optimizations when necessary. Depending on the application's requirements, you might need to consider alternative patterns that address performance more effectively.
Wrapping Up
Mastering the Visitor Pattern can significantly enhance your ability to manage complex data structures and encapsulate operations effectively. However, it is crucial to be aware of the common pitfalls during its implementation. By understanding the challenges and keeping the best practices in mind, you can leverage this design pattern to improve the maintainability and flexibility of your Java applications.
For further reading on design patterns in Java, consider exploring resources such as Refactoring Guru or Java Design Patterns.
Happy coding!