Choosing the Right Java Testing Framework for Your Project
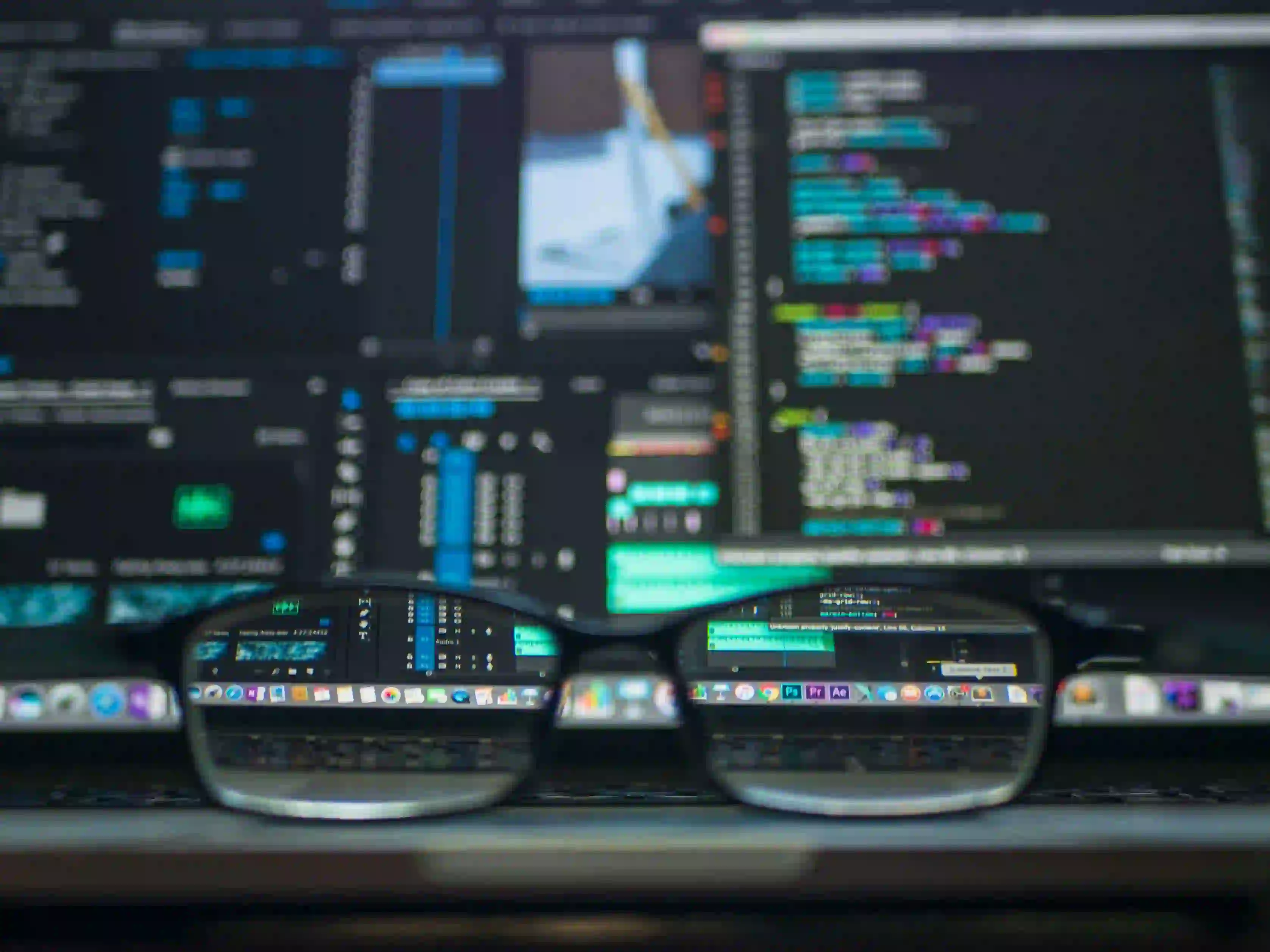
Choosing the Right Java Testing Framework for Your Project
As software development evolves, the significance of testing frameworks has become undeniable. Particularly in Java, selecting the right testing framework can enhance code quality, facilitate teamwork, and streamline workflows. With numerous options available, how do you choose the right one for your project? In this article, we will explore popular Java testing frameworks, their features, and how to decide which best suits your needs.
Understanding Java Testing Frameworks
Java testing frameworks are collections of tools and libraries designed to assist developers in writing and running tests. They provide structure and methodologies that simplify the process of validating your code. Testing plays a critical role in maintaining robustness, ensuring that your applications are bug-free and perform as expected.
Types of Testing Frameworks
Java testing frameworks can be categorized into:
-
Unit Testing Frameworks: These frameworks help in testing individual components or classes of the application in isolation. Examples include JUnit and TestNG.
-
Integration Testing Frameworks: These focus on testing how different components work together. Spring Test and Arquillian are notable examples.
-
Behavior-Driven Development (BDD) Frameworks: These frameworks are designed to facilitate communication between technical and non-technical stakeholders. Cucumber and JBehave are popular choices.
-
Mocking Frameworks: These frameworks allow for simulating behaviors of software components. Mockito and EasyMock are widely used.
Popular Java Testing Frameworks
1. JUnit
JUnit is perhaps the most famous unit testing framework in the Java ecosystem. Its simplicity and ease of use make it a go-to choice for beginners and seasoned developers alike.
Key Features:
- Annotations: JUnit utilizes annotations like
@Test
,@Before
, and@After
to easily define test methods and their lifecycle events. - Assertions: Provides a wide range of assertion methods to verify expected outcomes.
- Test Suites: You can group tests into suites for batch execution.
Example Code Snippet:
import org.junit.Assert;
import org.junit.Test;
public class CalculatorTest {
private Calculator calculator = new Calculator();
@Test
public void testAddition() {
int result = calculator.add(5, 3);
Assert.assertEquals(8, result); // Checking if the result is as expected
}
}
In this example, we create a simple test for an add
method in a Calculator class. The use of Assert.assertEquals
allows us to confirm that the method works correctly.
When to Use JUnit:
- Ideal for simple unit testing scenarios.
- Suitable for projects that are already based on JUnit or require minimal configuration.
2. TestNG
TestNG is another powerful testing framework that provides more flexibility compared to JUnit. It is designed to cover a broader range of testing needs.
Key Features:
- Annotations: TestNG also uses annotations but provides more options like
@DataProvider
, enabling parameterized tests. - Parallel Execution: Offers built-in features for running tests in parallel, reducing execution time.
- Dependency Testing: Allows specifying test dependencies, making it easier to manage the order of test execution.
Example Code Snippet:
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import static org.testng.Assert.assertEquals;
public class CalculatorTest {
@DataProvider(name = "additionData")
public Object[][] additionData() {
return new Object[][] {
{1, 2, 3},
{2, 3, 5},
{5, 7, 12}
};
}
@Test(dataProvider = "additionData")
public void testAddition(int a, int b, int expectedResult) {
Calculator calculator = new Calculator();
assertEquals(calculator.add(a, b), expectedResult); // Validate results
}
}
This snippet demonstrates a parameterized test with TestNG, allowing us to validate multiple cases in a cleaner manner.
When to Use TestNG:
- Good for complex test scenarios, especially when you need a high level of control over execution.
- Beneficial in projects that require parallel execution or sophisticated test configuration.
3. Mockito
Mockito is a popular mocking framework that complements unit testing by helping developers isolate specific components. It is particularly useful for testing parts of the code that rely on external components like services or databases.
Key Features:
- Easy to Use: Mockito is learner-friendly and allows for behavioral verification.
- Flexible Options: You can create mock objects and define their behaviors effortlessly.
Example Code Snippet:
import static org.mockito.Mockito.*;
import org.junit.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
UserRepository mockUserRepo = mock(UserRepository.class); // Create mock
when(mockUserRepo.findById(1)).thenReturn(new User("John", "Doe")); // Define behavior
UserService userService = new UserService(mockUserRepo);
User user = userService.getUser(1); // Call the method
assertEquals("John", user.getFirstName()); // Validate the result
}
}
In this example, we mock the UserRepository
to test the UserService
, maintaining isolation and ensuring that our test only focuses on the service logic.
When to Use Mockito:
- Ideal when testing code with external dependencies.
- Perfect for integration into projects that require heavy usage of dependency injection.
Factors to Consider When Choosing a Framework
Selecting the right testing framework involves assessing several factors:
-
Project Size and Complexity: Larger and more complex projects may benefit from frameworks like TestNG or a combination of JUnit with Mockito.
-
Team Familiarity: If your team is experienced with certain frameworks, sticking to those can improve productivity and code quality.
-
Community Support: Consider the framework's community and documentation. Robust support can greatly ease the learning curve.
-
Integration with CI/CD Pipelines: Look for frameworks that easily integrate with your existing tools and systems.
-
Performance: Certain frameworks allow for better performance in extensive test suites, such as TestNG's parallel testing capabilities.
The Closing Argument
Choosing the right Java testing framework can significantly impact the efficiency and effectiveness of your development process. Whether you opt for JUnit for its simplicity, TestNG for advanced features, or Mockito for mocking capabilities, ensure your selection aligns with your project goals and team strengths.
Investing time in establishing a robust testing framework will result in fewer bugs, higher code quality, and increased productivity. As your expertise grows, you might even find that a combination of these frameworks best suits your needs.
For more detailed insights into Java testing, consider checking out JUnit Documentation and Mockito Documentation.
As you dive into testing your Java applications, remember that the goal is not just to write tests, but to create a culture of quality and reliability in your software development lifecycle. Happy coding!