Solving VMware VI Java's User Name/Password Errors
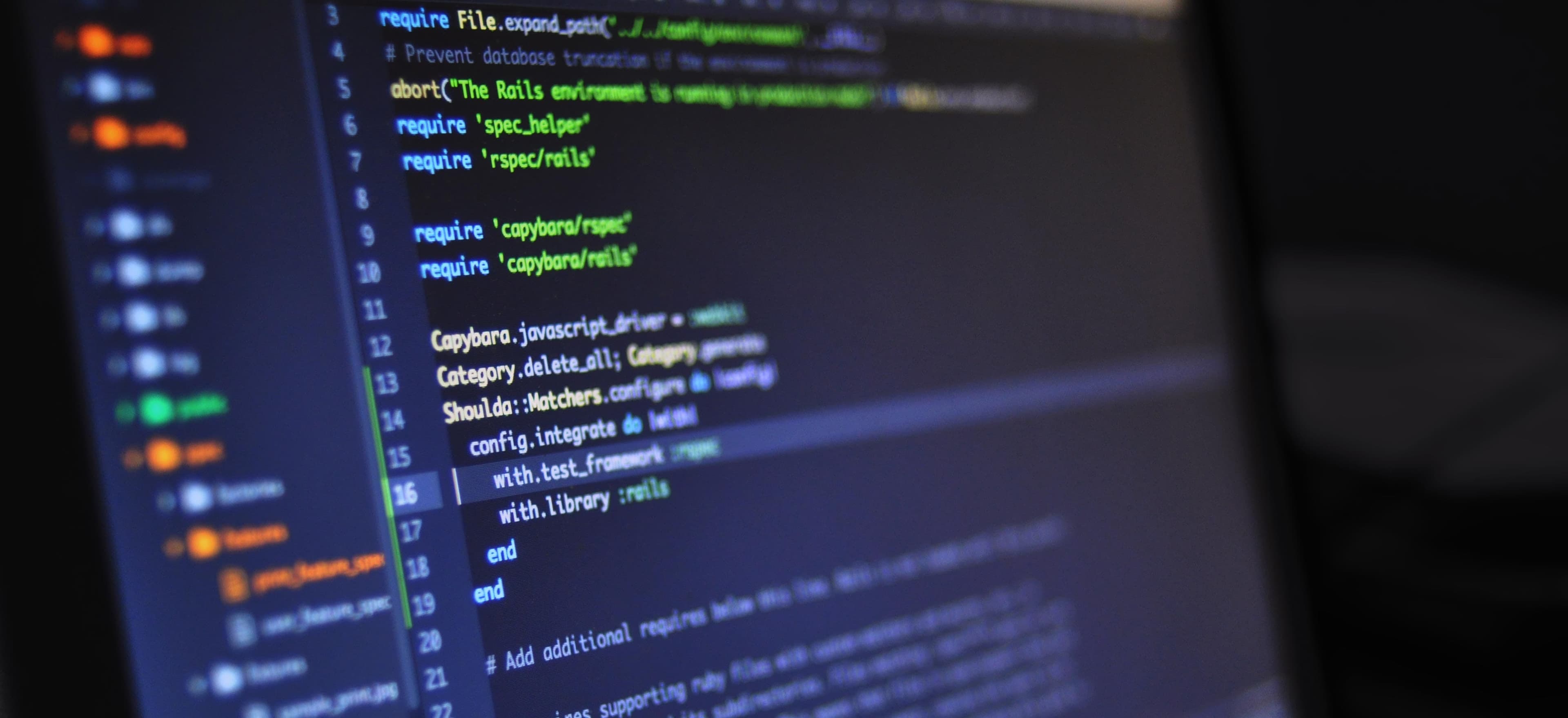
- Published on
Solving VMware VI Java's User Name/Password Errors
When working with VMware Infrastructure (VI), encountering user name and password errors is a common hiccup that can be both frustrating and time-consuming. However, identifying the root cause of these issues can simplify problem resolution, leading to smoother interactions with VMware environments. In this blog post, we will explore the typical user name/password errors, the underlying reasons for these errors, and convenient ways to resolve them using Java.
Understanding the User Name/Password Errors
Before diving into solutions, it's essential to grasp the types of user name/password errors that might occur:
- Invalid Credentials: This occurs when the username or password provided is incorrect.
- Account Lockout: Repeated incorrect login attempts may lead to account lockout due to security protocols.
- Expired Password: User accounts often have expiration policies; using an outdated password can result in errors.
- Connection Issues: Sometimes, the underlying problem may not stem from credentials, but rather network connectivity to the VMware server.
Quick Tip
Always double-check your credentials. It might seem trivial, but a simple typo can lead to authentication failures.
Why Java for VMware VI?
Java is a versatile programming language that's both scalable and robust, making it suitable for handling various tasks in managing VMware infrastructure. Moreover, Java's extensive API libraries facilitate complex operations and integrations.
To manage the VMware VI SDK effectively, it is crucial to keep the following libraries on your classpath:
- vSphere SDK for Java
- VMware vCenter Operations (optional for extended analytics)
Common Causes of Authentication Errors in VMware VI Java
- Character Encoding Issues: Sometimes, special characters in passwords can lead to encoding incompatibilities.
- Java Version Compatibility: Ensure you are using a compatible version of Java with your VMware SDK.
- Network Time Protocol (NTP): Time discrepancies between your machine and the VMware server can lead to authentication errors due to security procedures.
Implementing a Robust Connection in Java
Here's how to establish a connection using the VMware SDK in Java, along with error handling for username and password issues.
Sample Code Snippet
import com.vmware.vim25.*;
import com.vmware.vim25.mo.*;
public class VMwareConnector {
private String vmIpAddress;
private String userName;
private String password;
private ServiceInstance serviceInstance;
public VMwareConnector(String vmIpAddress, String userName, String password) {
this.vmIpAddress = vmIpAddress;
this.userName = userName;
this.password = password;
}
public void connect() {
try {
String url = "https://" + vmIpAddress + "/sdk/vimService";
serviceInstance = new ServiceInstance(new URL(url), userName, password, true);
System.out.println("Connected to VMware VI successfully!");
} catch (RemoteException e) {
System.err.println("Remote exception: " + e.getMessage());
handleError(e);
} catch (Exception e) {
System.err.println("Exception: " + e.getMessage());
}
}
private void handleError(RemoteException e) {
// Custom error handling
if (e.getMessage().contains("incorrect username or password")) {
System.err.println("Error: Invalid Username/Password.");
} else {
System.err.println("An error occurred: " + e.getMessage());
}
}
public void disconnect() {
if (serviceInstance != null) {
serviceInstance.getServiceContent().getSessionManager().logout();
System.out.println("Disconnected from VMware VI.");
}
}
}
Why This Code Works
In the above snippet:
- The
VMwareConnector
class initiates a connection using the provided VM IP, username, and password. - The
connect()
method builds the URL for the connection and attempts to create aServiceInstance
object. - In case of a
RemoteException
, we not only log the error but also have custom logic to check for authentication-related issues.
Important Considerations
- SSL Certificates: It’s advisable to ensure that SSL certificates are handled properly. Consider adding an SSL context if you’re using HTTPS.
- Error Handling: Efficient error handling can prevent application crashes and provide meaningful feedback to users.
Common Resolutions for User Name/Password Errors
- Reset the Credentials: If you forget your password, reset it, and ensure consistency in your stored credentials.
- Verify User Permissions: Ensure that your user account has the proper permissions to access the required resources on the VMware infrastructure.
- Check for Account Lockouts: If necessary, consult your system administrator to unlock your account or to review your login attempts.
- Monitor Network Connectivity: Make sure that your local machine can reach the VMware server without issues.
Additional Resources
To further deepen your understanding of the VMware infrastructure and Java integration, consider checking out:
- VMware Developer Documentation
- Java and VMware Integration.
In Conclusion, Here is What Matters
Solving user name/password errors in VMware VI using Java may initially seem cumbersome, but understanding the root causes and implementing robust error handling mitigates most issues. By following the step-by-step guide outlined in this post, you can navigate through these common errors effectively.
Remember, troubleshooting is not just about fixing code—it's about enhancing your understanding of the entire system. So keep learning, and happy coding as you delve into the fascinating world of VMware and Java integration!
Checkout our other articles