Avoiding Performance Pitfalls: jQuery Loops in Java Apps
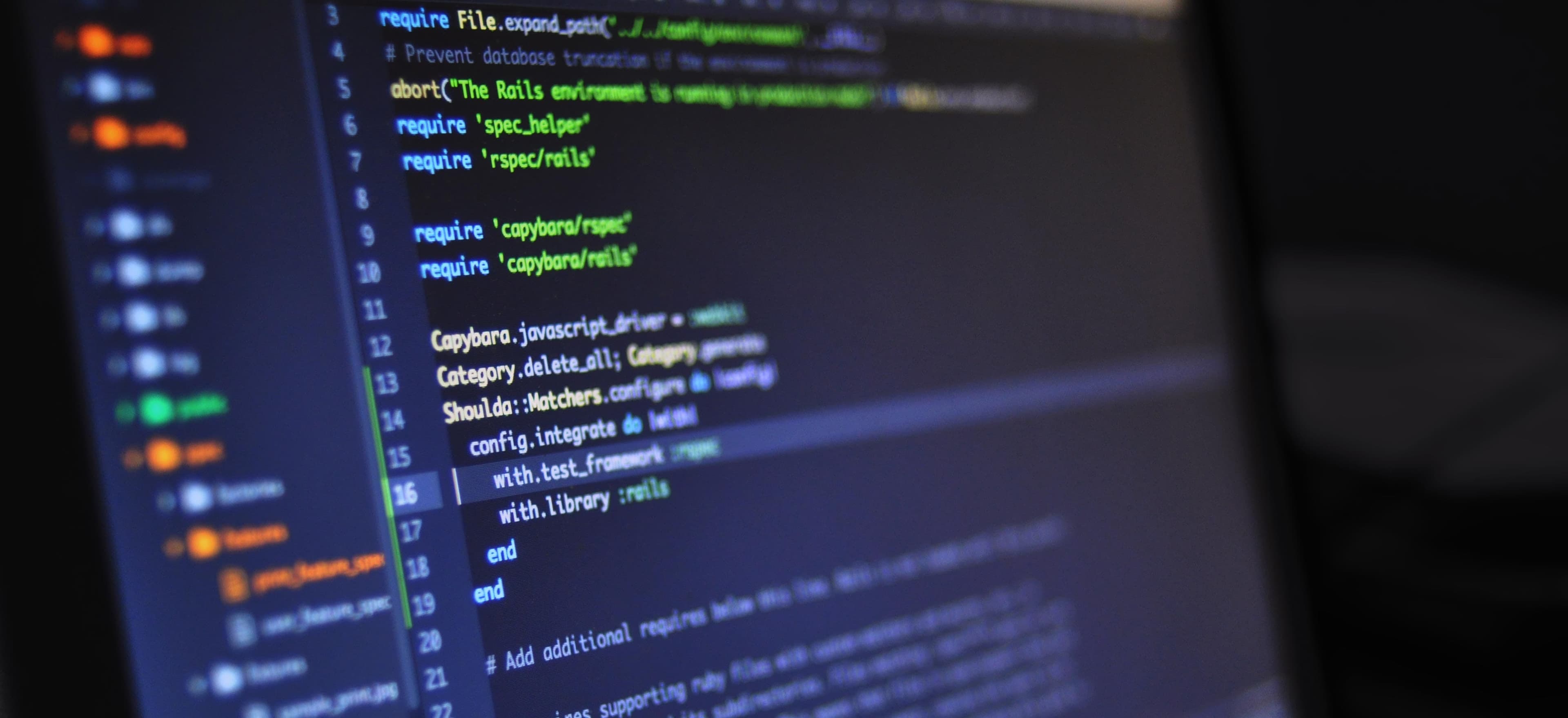
- Published on
Avoiding Performance Pitfalls: jQuery Loops in Java Apps
Java is a powerful language that can serve as the backbone for web applications. However, integrating front-end libraries such as jQuery into Java applications can lead to challenges, particularly when it comes to performance. In this article, we will explore how jQuery loops can affect performance and share strategies to optimize them for Java applications.
Understanding the Basics of jQuery
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies things like HTML document traversal and manipulation, event handling, and animation. However, its performance can degrade significantly if not used strategically, especially within loops.
Common Mistakes to Avoid
To learn more about the common mistakes when using jQuery functions in loops, let's take a closer look at how these errors can impact your Java applications.
Performance Issues with jQuery Loops
-
Repeated DOM Access: Each time you access the DOM inside a loop, it incurs a performance cost. This is because the browser has to search through the tree of elements again, which can be resource-intensive.
-
Inefficient Event Handling: Attaching events inside a loop can lead to multiple listeners for the same element, causing memory leaks and performance hits.
-
Using jQuery for Heavy Computational Tasks: jQuery is optimized for manipulating DOM elements and handling events. Using it for heavy computations may not yield the expected results in speed.
Optimizing jQuery Loops
Use Caching
One of the simplest optimizations is to cache jQuery selectors. Instead of querying the DOM multiple times, you can store the result of the selector in a variable.
var items = $('.item'); // Cache the selection
items.each(function(index, element) {
// Now `element` can be used without querying the DOM again
$(element).text('Item ' + (index + 1));
});
Why Cache? Caching the selection minimizes DOM access, speeding up the loop execution, especially for elements accessed repeatedly.
Utilize Event Delegation
Instead of binding events to multiple elements inside a loop, use event delegation. This binds the event to a parent element, which then captures events triggered by its children.
$('#parent').on('click', '.child', function() {
alert('Child clicked');
});
Why Delegate? Event delegation reduces the memory overhead that comes from attaching multiple event listeners, allowing your application to scale better.
Minimize DOM Manipulation
Batch your DOM manipulations. Instead of updating the DOM after each iteration, you can build a fragment and update it all at once.
var itemsFragment = document.createDocumentFragment();
$('.item').each(function(index, element) {
var newItem = document.createElement('div');
newItem.textContent = 'Item ' + (index + 1);
itemsFragment.appendChild(newItem);
});
document.getElementById('itemContainer').appendChild(itemsFragment);
Why Batch Updates? This approach reduces the number of reflows and repaints the browser has to perform, leading to better performance.
Implementing Promises for Asynchronous Loops
Loops that rely on asynchronous operations can be managed more effectively through promises. Instead of nesting callbacks, you can leverage promises to manage your asynchronous flow cleanly.
function fetchData(url) {
return new Promise((resolve) => {
$.get(url, function(data) {
resolve(data);
});
});
}
const urls = ['url1', 'url2', 'url3'];
Promise.all(urls.map(fetchData)).then(function(results) {
console.log(results);
});
Why Use Promises? Promises simplify the management of asynchronous operations, avoiding the callback hell scenario and improving code readability.
Profiling Java Applications with jQuery
For developers working with extensive Java applications that incorporate jQuery, performance profiling becomes critical. Tools like Chrome DevTools can help you measure performance bottlenecks caused by inefficient jQuery loops.
- Open Chrome DevTools: Right-click on your page and select "Inspect".
- Go to the Performance tab: Click on the Record button and interact with your page to capture a performance profile.
- Analyze the calls: Look for long-running jQuery functions and DOM manipulations in your recorded actions.
These metrics can guide you in optimizing the parts of your application that require the most attention.
Integration with Java
When integrating jQuery with Java, especially using frameworks like Spring Boot, ensuring that performance across layers is maintained is key. Java’s robust back-end capabilities must align well with jQuery’s front-end manipulations for optimal user experience.
-
Perform Server-side Operations: Heavy computations should be performed on the server-side in Java, and only the resultant data should be sent to the client.
-
Load Data Asynchronously: Use Ajax calls to fetch data and update only the necessary parts of your webpage dynamically.
// Java Controller in Spring Boot
@GetMapping("/data")
public ResponseEntity<List<Item>> getData() {
List<Item> items = itemService.getAllItems();
return new ResponseEntity<>(items, HttpStatus.OK);
}
This method limits client-side loading time and keeps the user interface responsive.
The Closing Argument
Performance optimization is a multidimensional challenge that can significantly affect user experience in your Java applications. When using jQuery, it's crucial to avoid common pitfalls that can lead to performance degradation. By caching DOM elements, utilizing event delegation, batching DOM manipulations, using promises for asynchronous tasks, and effectively profiling your application, you can enhance performance.
For more insights on optimizing jQuery functions, refer to the common mistakes when using jQuery functions in loops.
With these strategies, you can ensure that your Java applications leverage jQuery effectively while maintaining performance, providing end-users with a smooth and efficient experience.
Checkout our other articles