Mastering Java Idioms: A Key to Efficient Programming
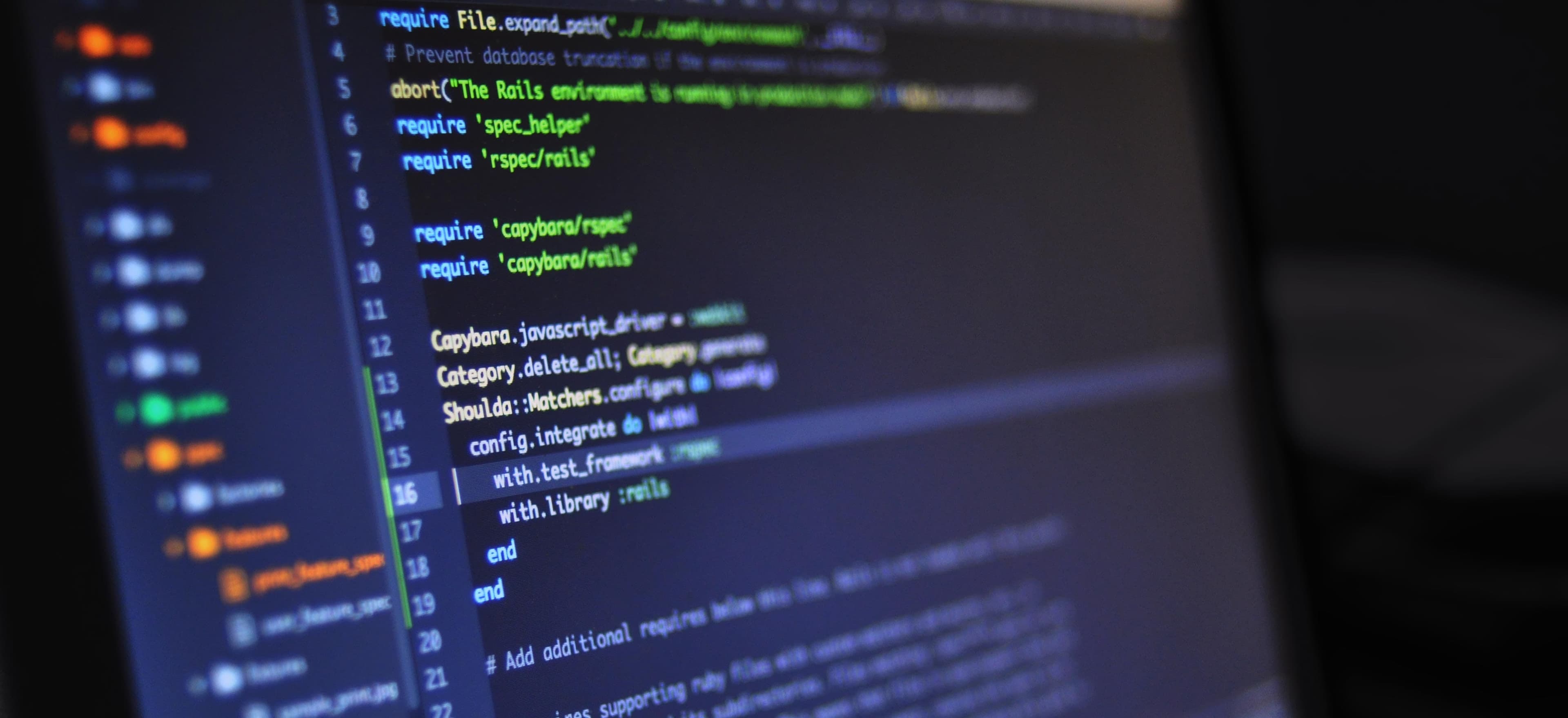
- Published on
Mastering Java Idioms: A Key to Efficient Programming
Java, one of the most widely used programming languages, is renowned for its clarity and ease of use. However, even seasoned developers can sometimes get lost in the vast sea of syntax and constructions. One effective approach to mastering Java is to understand its idioms—commonly accepted patterns and solutions that improve code readability and maintainability.
What are Idioms in Programming?
Idioms in programming refer to conventions, best practices, and syntactical nuances that developers employ to write clear and efficient code. They help in avoiding common pitfalls and facilitate better collaboration among programmers. Just as idioms in natural languages convey meanings that go beyond mere word definitions, programming idioms encapsulate complex programming concepts in a more manageable form.
In Java, using idioms can significantly enhance the expressive power of the language. They not only improve readability but also make code less error-prone. For instance, knowing how to efficiently handle collections or implement design patterns can elevate one from a novice to a proficient programmer.
Why Study Java Idioms?
-
Maintainability: Code that's clear and adheres to idiomatic practices is easier to read and understand. This is beneficial for both the original author and others who might work on the code later.
-
Performance: Many idioms encapsulate efficient ways to perform common tasks. Knowing these idioms can lead to better-performing applications.
-
Collaboration: In a team setting, using idioms makes communication easier. Everyone uses a common vocabulary—reducing misunderstandings and errors.
Overall, idioms symbolize accumulated knowledge in the programming community, and mastering them can save time and headaches in the long run.
Common Java Idioms
Here, we'll explore a few common Java idioms, complete with code snippets and explanations.
1. The Enhancements of the For-Each Loop
The for-each loop simplifies iterating over collections and arrays. It enhances readability and reduces the chance of errors commonly associated with traditional index-based loops.
Example:
List<String> fruits = Arrays.asList("Apple", "Banana", "Cherry");
for (String fruit : fruits) {
System.out.println(fruit);
}
Why: This code snippet is cleaner and avoids potential IndexOutOfBoundsExceptions that could occur with traditional loops.
2. The Singleton Pattern
The Singleton Pattern ensures a class has only one instance and provides a global access point to it. This is particularly useful for managing shared resources like configurations or logging.
Example:
public class Singleton {
private static Singleton instance;
private Singleton() {
// private constructor
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Why: By using a private constructor and providing a static method for access, this idiom controls the instantiation process. It guarantees that no other instance of Singleton
can be created.
3. Builder Pattern for Creating Complex Objects
When dealing with complex objects, the Builder Pattern allows for step-by-step construction, enhancing code clarity.
Example:
public class User {
private String name;
private int age;
private User(UserBuilder builder) {
this.name = builder.name;
this.age = builder.age;
}
public static class UserBuilder {
private String name;
private int age;
public UserBuilder setName(String name) {
this.name = name;
return this;
}
public UserBuilder setAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(this);
}
}
}
// Usage
User user = new User.UserBuilder()
.setName("John Doe")
.setAge(30)
.build();
Why: The Builder Pattern separates the construction of a complex object from its representation, allowing for more flexible and readable code.
4. Stream API for Collection Manipulation
Since Java 8, the Stream API has provided an efficient way to handle collections. This idiom promotes a functional approach by allowing chain operations.
Example:
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.stream()
.filter(name -> name.startsWith("J"))
.forEach(System.out::println);
Why: The Stream API makes code concise and expressive. Instead of writing a verbose loop, you express your intent clearly through method chaining.
Learning and Practicing Idioms
As with any skill, mastering Java idioms requires practice and continuous learning. The world of programming idioms is vast, and it evolves as new versions of Java are released.
For more insights into idiomatic expressions, you might find it beneficial to cross-reference with "Geheimcode Englisch: Die faszinierende Welt der Idiome" here. The approach of exploring language idioms can parallel the understanding of programming idioms by enhancing your overall comprehension of both domains.
Bringing It All Together
Mastering Java idioms is a gateway to becoming a more effective and efficient programmer. Understanding and applying these idioms not only leads to better code but also fosters communication within developer teams. The key is practice—exploring these idioms in real-world applications will cement your understanding and enhance your programming abilities.
As you progress in your Java coding journey, continually revisit common idioms. They’re not just technical tricks; they're pathways to writing better code and achieving professional growth in the language you love to use.
Checkout our other articles