Unlocking the Hidden Emotions Through Java's Data Analysis
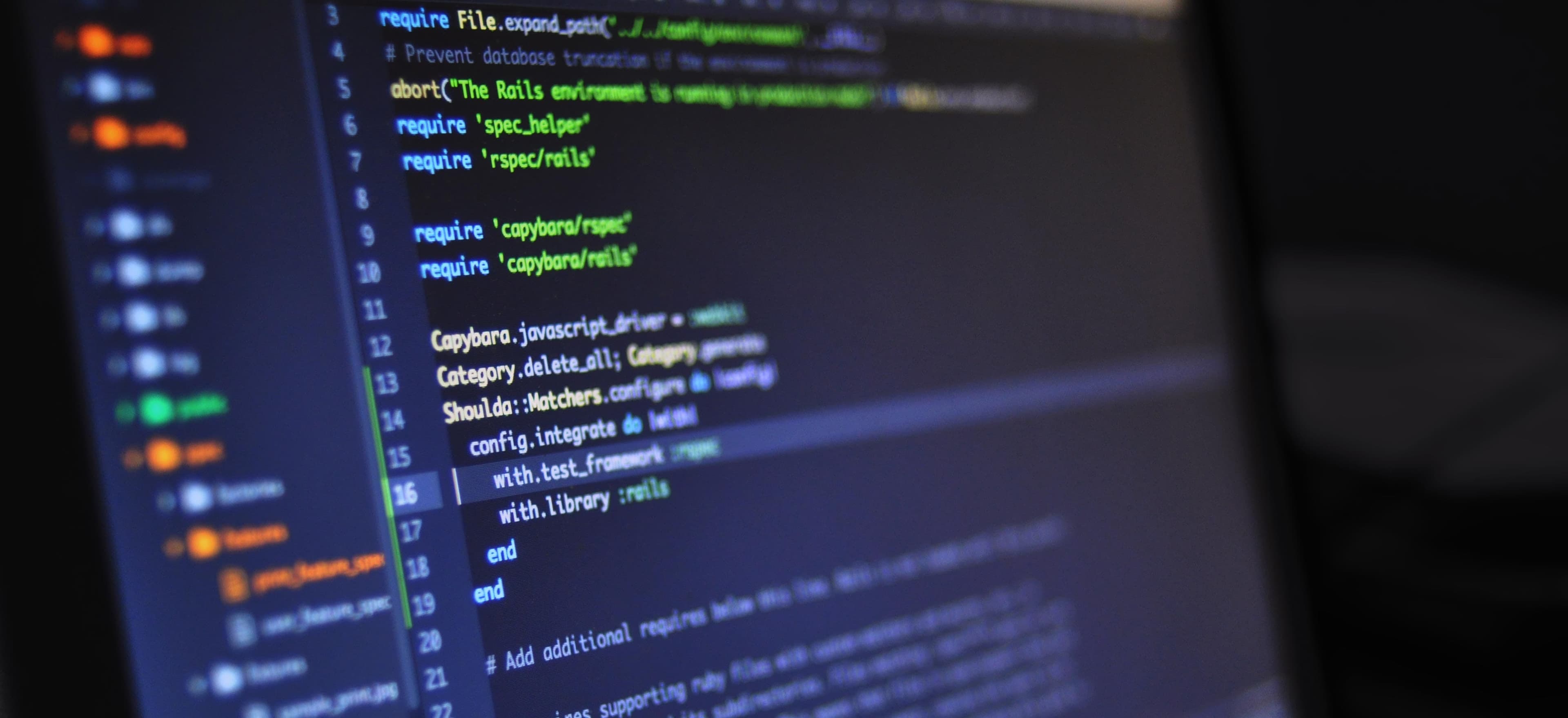
- Published on
Unlocking the Hidden Emotions Through Java's Data Analysis
In today's digital age, the need to analyze emotional content has become increasingly important. Businesses collect vast amounts of data, often filled with insights about customer sentiments and preferences. This article delves into how Java can be utilized effectively to unlock hidden emotions within data through data analysis. We will explore practical examples, necessary libraries, and efficient techniques to process and analyze data seamlessly.
Understanding Data Analysis
Data analysis refers to the process of inspecting, cleansing, transforming, and modeling data to discover useful information, inform conclusions, and support decision-making. This process is paramount in various fields, including marketing, finance, healthcare, and even arts. As detailed in the article "Träume entziffern: Tor zum geheimnisvollen Innenleben" (accessible at auraglyphs.com/post/traeume-entziffern-tor-zum-geheimnisvollen-innenleben), understanding subjective experiences can change how we interact with the world around us. Through Java, we can uncover the emotional undertones of raw data.
Why Choose Java for Data Analysis?
Java is a robust, platform-independent, and widely-used programming language, making it an excellent choice for data analysis tasks.
Benefits of Using Java:
- Portability: Write once, run anywhere. Java applications can easily be deployed on various platforms.
- Performance: Java's performance is often impressive due to its Just-In-Time (JIT) compiler and excellent memory management.
- Libraries: There are numerous libraries tailored for data analysis, like Apache Commons Math, Weka, and Deeplearning4j.
- Community Support: Java has a vast community, ensuring you will find help, tutorials, and documentation.
Essential Libraries for Data Analysis in Java
To effectively perform data analysis, leveraging existing libraries can significantly simplify your work. Here are some notable libraries to consider:
- Apache Commons Math: This library provides tools for various statistical analyses, solving linear systems, and optimization.
- Weka: A comprehensive suite for machine learning algorithms, Weka is perfect for carrying out data mining tasks.
- Deeplearning4j: This library facilitates deep learning applications in Java, making it flexible for complex data analysis tasks.
Let's exemplify how we can perform sentiment analysis, a crucial aspect of identifying hidden emotions within text data.
Example: Sentiment Analysis with Weka
Sentiment analysis is a natural language processing (NLP) task that involves determining the emotional tone behind a body of text. Below, we present a simple Java application to perform sentiment analysis on textual data using the Weka library.
Step 1: Setting Up Weka
Before diving into the code, ensure that you have the Weka library included in your Java project. If you're using Maven, you can add the following dependency in your pom.xml
:
<dependency>
<groupId>nz.ac.waikato.cms.weka</groupId>
<artifactId>weka-stable</artifactId>
<version>3.8.6</version>
</dependency>
Step 2: Creating the Data Classifier
Here's how to perform sentiment analysis using a simple Naive Bayes classifier:
import weka.classifiers.Classifier;
import weka.classifiers.bayes.NaiveBayes;
import weka.core.DenseInstance;
import weka.core.Instance;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import java.util.Arrays;
public class SentimentAnalysis {
private Classifier classifier;
public SentimentAnalysis(String modelFile) throws Exception {
DataSource source = new DataSource(modelFile);
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1);
classifier = new NaiveBayes();
classifier.buildClassifier(data);
}
public String classify(String[] features) throws Exception {
Instance instance = new DenseInstance(1.0, Arrays.stream(features)
.mapToDouble(Double::parseDouble)
.toArray());
instance.setDataset(data);
double result = classifier.classifyInstance(instance);
return data.classAttribute().value((int) result);
}
}
Explanation of the Code
- Classifier Initialization: We initialize a Naive Bayes classifier and train it with a dataset. A dataset must be in Weka's .arff format.
- Feature Extraction: The
classify
method takes an array of string features that represent text data transformed into numerical values. - Class Prediction: Finally, we classify the instance and return the predicted sentiment label.
Step 3: Using the Classifier
To use the classifier and see it in action:
public static void main(String[] args) {
try {
SentimentAnalysis sentimentAnalysis = new SentimentAnalysis("path/to/model.arff");
String[] features = {"0.5", "0.4", "0.1"}; // Example feature values
String sentiment = sentimentAnalysis.classify(features);
System.out.println("Predicted Sentiment: " + sentiment);
} catch (Exception e) {
e.printStackTrace();
}
}
In this example, we initiate our sentiment analysis by passing feature values to the classifier and printing out the predicted sentiment.
Closing the Chapter
Data analysis opens the door to understanding hidden emotions in text data. With Java, the process becomes more straightforward utilizing libraries like Weka, which simplify complex tasks such as sentiment analysis.
Whether you're exploring consumer sentiments or analyzing literary texts, Java equips you with the necessary tools to harness the power of data. This analysis not only enhances business decisions but also deepens our understanding of human experiences as highlighted in "Träume entziffern: Tor zum geheimnisvollen Innenleben".
For more information on analyzing emotions and insights, refer to the comprehensive resource at auraglyphs.com/post/traeume-entziffern-tor-zum-geheimnisvollen-innenleben.
By unlocking these insights, you're not just analyzing data – you're gaining a deeper understanding of the thoughts and feelings that drive human behavior. Implement these strategies in your projects and see how Java can transform your approach to data analysis.
Checkout our other articles