Resolving MongoDB Connection Problems in Java Apps
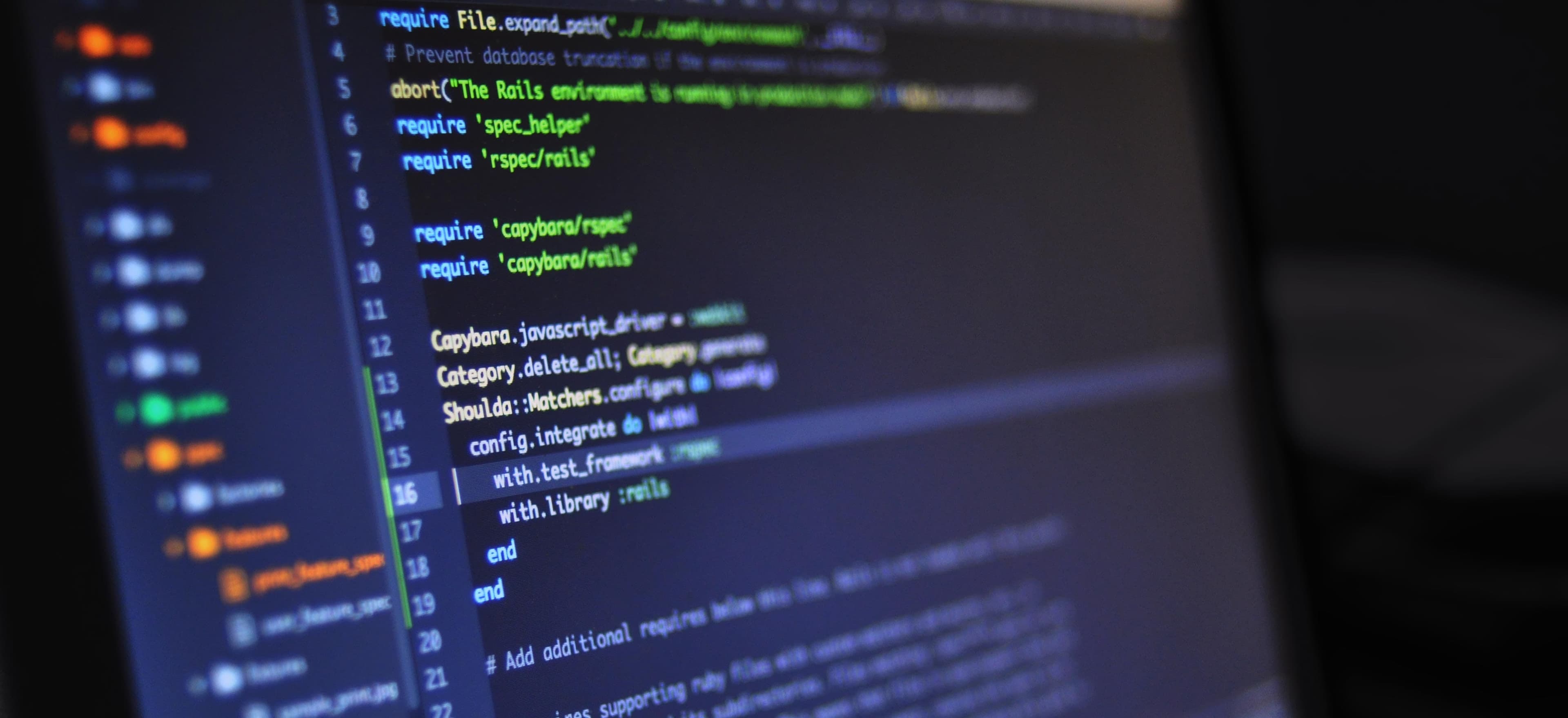
- Published on
Resolving MongoDB Connection Problems in Java Apps
In today’s data-driven world, MongoDB has emerged as one of the leading NoSQL databases, thanks to its flexibility, scalability, and powerful querying capabilities. However, integrating MongoDB with Java applications can sometimes present challenges, especially with connection problems. In this article, we will explore common issues that developers face when connecting MongoDB with Java applications and how to resolve them efficiently.
Table of Contents
- Understanding MongoDB Connection Basics
- Common Connection Issues
- Incorrect Connection String
- Firewall and Network Configuration
- Authentication Problems
- Code Example: Establishing a Connection
- Troubleshooting Connection Problems
- Conclusion and Further Reading
Understanding MongoDB Connection Basics
A typical connection to a MongoDB instance in a Java application is established using the MongoDB Java Driver. This driver provides a seamless method to connect, query, and manipulate data stored in MongoDB.
Before diving into the connection issues, let’s recap how to set up the MongoDB Java Driver in your project. You can add the dependency via Maven:
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongo-java-driver</artifactId>
<version>3.12.10</version> <!-- Use the latest stable version -->
</dependency>
This snippet adds the necessary driver to your project, allowing you to interact with MongoDB effortlessly.
Common Connection Issues
While setting up MongoDB in your Java application, you may encounter a series of issues. Below are some common problems and their solutions.
1. Incorrect Connection String
One of the most frequent problems developers face is an incorrect connection string format. The connection string is a URI that provides MongoDB with pertinent information on where to connect.
A typical MongoDB connection string looks like this:
mongodb://username:password@localhost:27017/databaseName
- Solution: Check your connection string for typos. Make sure the username, password, hostname, and database name are correct.
2. Firewall and Network Configuration
Firewalls and network settings may block the connection to MongoDB. By default, MongoDB runs on port 27017, and this port may not be open or accessible.
- Solution: Ensure that the firewall is configured to allow traffic on port 27017. You can either disable the firewall or allow access specifically for this port.
3. Authentication Problems
If your MongoDB instance requires authentication, you’ll need to ensure that the correct credentials are provided in your connection string.
- Solution: Double-check your credentials. If your MongoDB setup uses roles and permissions, make sure the user you are connecting with has the necessary access rights.
Code Example: Establishing a Connection
Now, let's look at a code snippet that establishes a connection to a MongoDB database using the MongoDB Java Driver.
import com.mongodb.MongoClient;
import com.mongodb.MongoClientURI;
import com.mongodb.client.MongoDatabase;
public class MongoDBExample {
public static void main(String[] args) {
// Setting up the connection string
String uriString = "mongodb://username:password@localhost:27017/myDatabase";
// Create a MongoClientURI object
MongoClientURI uri = new MongoClientURI(uriString);
// Establish the MongoClient connection
MongoClient mongoClient = new MongoClient(uri);
// Access the database
MongoDatabase database = mongoClient.getDatabase("myDatabase");
System.out.println("Connected to the database successfully!");
// Always close the client to free resources
mongoClient.close();
}
}
Commentary on the Code
This simple example allows you to connect to a MongoDB database. We begin by defining a connection URI—pay close attention to the username, password, and the database we want to access.
The MongoClientURI
class allows for easy parsing of the connection string, providing a more structured approach to connect to MongoDB. With the established MorganClient
, you can access your database and perform operations.
Importance of Resource Management
It's crucial to close the MongoClient
after use. Failing to do so can lead to resource leaks and other performance issues. Always remember to handle your connections prudently.
Troubleshooting Connection Problems
When facing MongoDB connection issues, there are some steps you can take to effectively troubleshoot:
-
Check Logs: MongoDB server logs can provide insights into connection attempts and errors. Look for error messages that can guide you in resolving the issue.
-
Ping MongoDB: Use tools like
ping
ortelnet
to verify that you can reach your MongoDB server from the client machine. This helps eliminate network-related issues. -
Test Connection in Isolation: Create a minimal Java application that only establishes a connection to MongoDB. This can help determine if the problem is with your broader application or isolated to MongoDB connectivity.
-
Consult the Documents: For in-depth issues, the official MongoDB documentation contains solutions for various setup and connection problems.
-
Review Relevant Articles: Articles such as “Common Installation Issues with MongoDB on Oracle Linux” provide helpful insights and could address issues stemming from the installation process. You can read more here.
To Wrap Things Up and Further Reading
MongoDB integration in Java applications can be seamless if you address connection problems judiciously. Whether it's the connection string, network firewalls, or authentication issues, there’s always a path to resolution.
As you continue working with MongoDB and Java, consider diving into topics like connection pooling and optimizing your database queries for performance. A solid understanding of these concepts will further enhance your application’s capabilities.
For continuing education on MongoDB and best practices, be sure to explore MongoDB University for free courses.
The world of databases may seem daunting, but with practice, knowledge, and resources at your disposal, resolving MongoDB connection issues in Java applications will become second nature.
Feel free to reach out with any questions in the comments below, and good luck with your MongoDB and Java journey!
Checkout our other articles