Mastering Resilience: Chaos Management in Java Applications
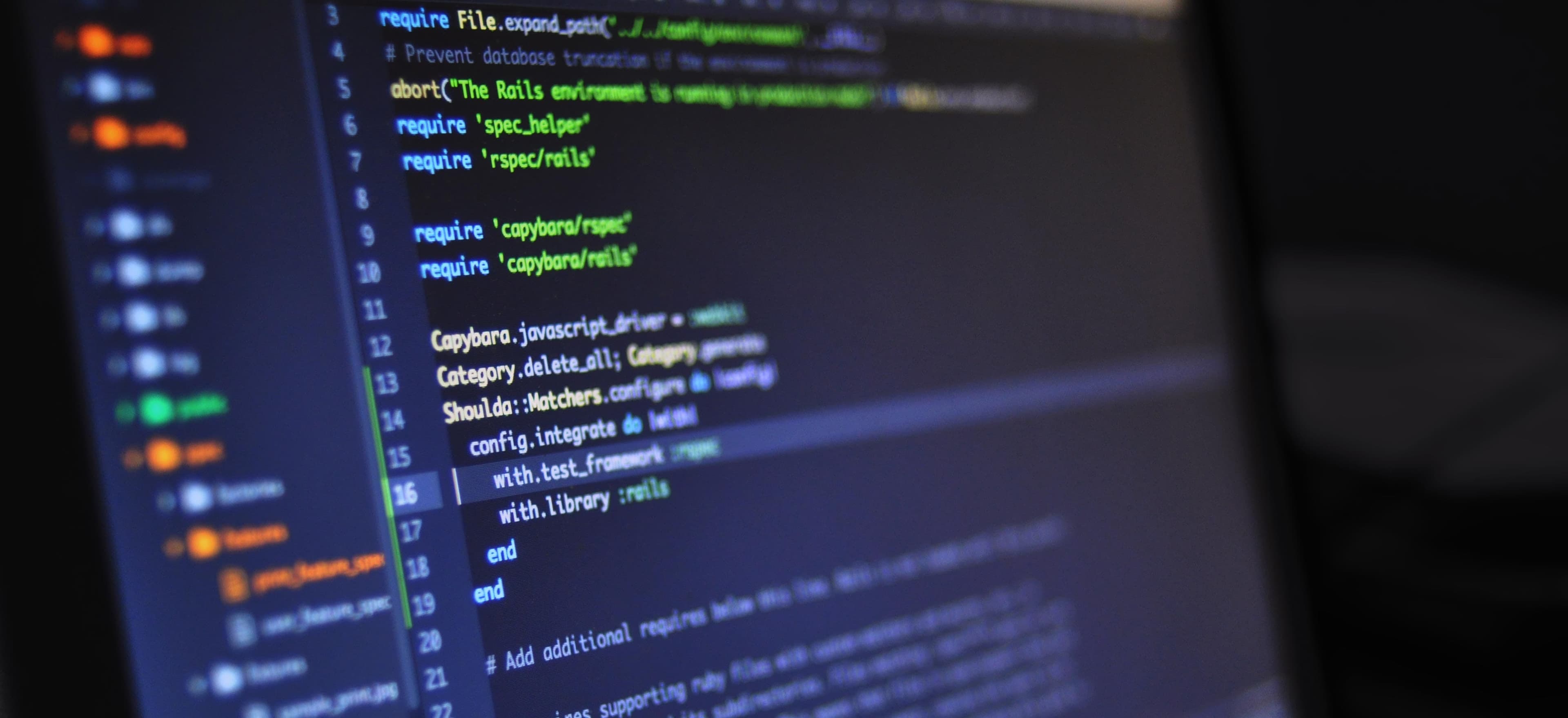
- Published on
Mastering Resilience: Chaos Management in Java Applications
In today's rapidly changing technological landscape, building resilient applications that can withstand unexpected challenges is paramount. One of the most profound lessons learned from the world of software development is not just how to create robust code but how to ensure that applications can survive chaos. This principle is notably reflected in Netflix's "Preppers," a valuable resource on managing chaos in systems. For a deeper understanding, you can check out the article titled Surviving Chaos: Key Lessons from Netflix's Preppers.
In this blog post, we'll explore how to apply chaos management principles to Java applications, emphasizing strategies and patterns that help maintain system stability under duress. We’ll discuss exceptional handling, implementing Circuit Breakers, load balancing, and leveraging resiliency frameworks. Let’s dive right in!
Understanding Chaos Engineering
At its core, chaos engineering is the discipline of experimenting on a system to build confidence in its capability to withstand turbulent conditions. This pre-emptive approach allows developers to understand failure modes before they happen. By proactively identifying these failures, we can implement procedures and code to handle them gracefully.
Key Practices for Java Applications
- Graceful Error Handling
- Circuit Breaker Pattern
- Load Balancing
- Service Discovery
- Utilizing Resilience Libraries
Let's break these concepts down further.
1. Graceful Error Handling
One of the first steps toward resilience is the effective management of errors. Any Java application worth its salt should have comprehensive error handling, which ensures that the application remains operational, even when problems arise.
Example: Exception Handling
public void fetchData() {
try {
// Simulate API call
String data = callExternalService();
System.out.println(data);
} catch (IOException e) {
System.err.println("Network error occurred. Please retry later.");
// Implement retry logic or fallback mechanism
} catch (Exception e) {
System.err.println("An unexpected error occurred: " + e.getMessage());
// Additional logging or fallback mechanism
}
}
Why it Matters: By catching specific exceptions, we not only prevent the application from crashing but can also implement specific recovery strategies. This contributes significantly to maintaining service availability.
2. Circuit Breaker Pattern
The Circuit Breaker pattern is a design pattern used to detect failures and encapsulate the logic of preventing the application from performing actions likely to fail. This prevents a failing service from being repeatedly called, which can exacerbate the problem.
Example: Simple Circuit Breaker Implementation
public class CircuitBreaker {
private final long timeout;
private final int threshold;
private int failureCount = 0;
private long lastFailureTime;
public CircuitBreaker(long timeout, int threshold) {
this.timeout = timeout;
this.threshold = threshold;
}
public void callService() {
if (shouldOpenCircuit()) {
throw new RuntimeException("Circuit is open. Service is down.");
}
try {
// Simulate service call
performServiceCall();
} catch (Exception e) {
failureCount++;
lastFailureTime = System.currentTimeMillis();
if (failureCount >= threshold) {
System.out.println("Circuit opened after " + failureCount + " failures.");
}
throw e;
}
}
private boolean shouldOpenCircuit() {
return failureCount >= threshold && System.currentTimeMillis() - lastFailureTime < timeout;
}
private void performServiceCall() {
// Simulate successful service call
System.out.println("Service called successfully.");
}
}
Why it Matters: The circuit breaker prevents further calls to a failing service, allowing it time to recover and reducing unnecessary strain on both the service and your application. This embedded self-protection maintains overall system resilience.
3. Load Balancing
In a microservices architecture, distributing workloads across multiple instances is vital to prevent any single point of failure. Load balancing improves the availability and reliability of your application.
Example: Using Spring Cloud Load Balancer
If you're working with Spring Boot, the Spring Cloud Load Balancer can automatically handle this for you.
@Service
public class ProductService {
@LoadBalanced
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
public Product getProduct(String productId) {
ResponseEntity<Product> response = restTemplate()
.getForEntity("http://product-service/products/" + productId, Product.class);
return response.getBody();
}
}
Why it Matters: The @LoadBalanced
annotation allows Spring to distribute requests effectively across service instances. This increases application availability while providing fault tolerance.
4. Service Discovery
For applications in microservices architecture, service discovery plays a critical role in ensuring that services can dynamically find and communicate with each other without hardcoded address definitions.
Example: Using Eureka for Service Discovery
@SpringBootApplication
@EnableEurekaClient
public class InventoryServiceApplication {
public static void main(String[] args) {
SpringApplication.run(InventoryServiceApplication.class, args);
}
}
Why it Matters: By using tools like Eureka, services can register themselves, making it easier for other services to locate them. This dynamic nature prevents cascading failures when a service goes down.
5. Utilizing Resilience Libraries
There are several libraries designed to help with implementing resilience patterns. Libraries like Resilience4j provide a comprehensive set of tools for fault tolerance.
Example: Using Resilience4j
import io.github.resilience4j.circuitbreaker.annotation.CircuitBreaker;
@Service
public class ExternalServiceClient {
@CircuitBreaker
public String callExternalService() {
// Call to an external service that might fail
return restTemplate.getForObject("http://external-service/data", String.class);
}
}
Why it Matters: By incorporating these resilience patterns via libraries, you significantly reduce the boilerplate code and improve the maintainability of your application.
In Conclusion, Here is What Matters
Mastering chaos management in Java applications is essential for creating resilient systems. Developers are often faced with unexpected disruptions, and the strategies outlined in this blog post—like effective error handling, Circuit Breakers, and leveraging load balancers—are all essential for preparing for these challenges.
To learn more about resilience in system design, the lessons from Netflix's "Preppers" provide an enlightening perspective (check out Surviving Chaos: Key Lessons from Netflix's Preppers). The principles of chaos engineering not only enhance your application but also instill confidence in your architecture.
It's not just about surviving chaos; it's about mastering resilience. By implementing these strategies, you will not only enhance the robustness of your Java applications but also ensure a better experience for your users, even in times of trouble. Whether you're building new applications or revisiting existing ones, these lessons can make a world of difference. Happy coding!
Checkout our other articles