Mastering Resilience: Java Strategies for Chaos Management
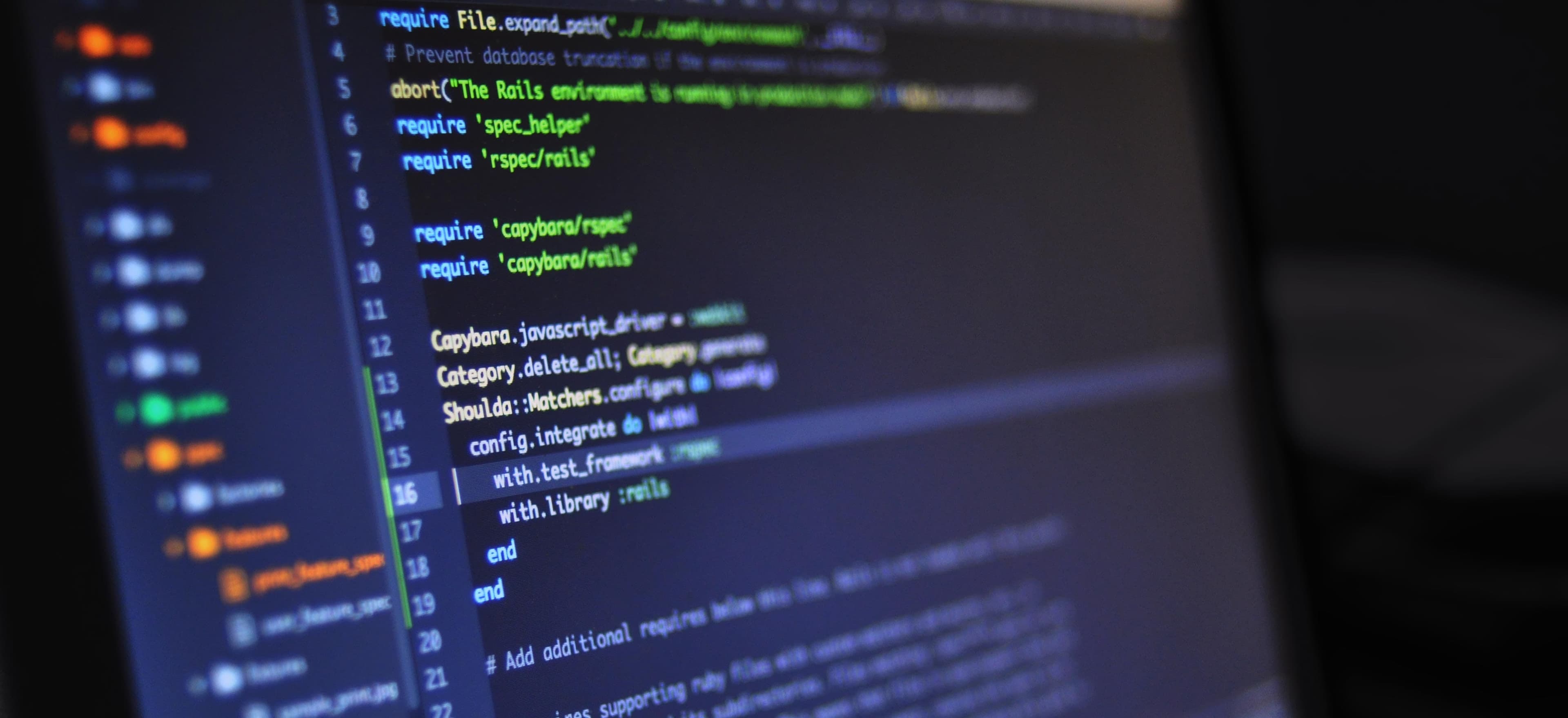
- Published on
Mastering Resilience: Java Strategies for Chaos Management
In today’s fast-paced software development landscape, harnessing resilience is essential. Much like how businesses can learn from chaos management strategies outlined in Surviving Chaos: Key Lessons from Netflix's Preppers (available here), developers can adopt resilience strategies to enhance their Java applications. This article delves into the intricacies of creating resilient Java applications while managing chaos effectively.
What is Resilience in Software Development?
Resilience refers to the ability of a system to adapt to various conditions and maintain desired functionality even under stress. In the context of Java applications, resilience can mean handling edge cases, managing failures, and ensuring consistency during high loads or unexpected interruptions.
Why is Resilience Important?
- User Trust: Users expect applications to be reliable. When systems fail, user trust diminishes.
- Cost Efficiency: Handling failures gracefully can reduce troubleshooting and support costs.
- Competitive Advantage: Resilient applications stand out in a crowded marketplace.
Essential Strategies for Achieving Resilience with Java
1. Implementing Circuit Breaker Patterns
The circuit breaker pattern prevents an application from performing an action that is likely to fail. Just like a circuit breaker in electrical systems, when too many failures are detected, the application "breaks" to avoid cascading failures.
Example Code Snippet
import java.util.concurrent.TimeoutException;
public class CircuitBreaker {
private int failureThreshold;
private int currentFailures = 0;
private boolean isOpen = false;
public CircuitBreaker(int failureThreshold) {
this.failureThreshold = failureThreshold;
}
public void call(Runnable action) throws TimeoutException {
if (isOpen) {
throw new TimeoutException("Circuit is open, action cannot be performed.");
}
try {
action.run();
currentFailures = 0; // Reset on success.
} catch (Exception e) {
currentFailures++;
if (currentFailures >= failureThreshold) {
isOpen = true; // Open the circuit.
}
throw e; // Rethrow the exception.
}
}
}
Commentary
In the code above, we define a CircuitBreaker
class that tracks the number of failures. If the failures exceed a certain threshold, subsequent calls are disallowed until the circuit is "closed" again. This ensures that the system remains responsive by avoiding retries on failing operations.
2. Employing Retries with Exponential Backoff
When facing transient failures, a simple retry mechanism can be effective. Using exponential backoff can ensure that retries don't overwhelm the system.
Example Code Snippet
import java.util.Random;
public class RetryHandler {
public static void executeWithRetry(Runnable action, int retries) {
Random random = new Random();
for (int i = 0; i < retries; i++) {
try {
action.run();
return; // Success, exit the method.
} catch (Exception e) {
System.out.println("Retrying... attempt " + (i + 1));
try {
// Exponential backoff: wait for 2^i seconds.
Thread.sleep((long) Math.pow(2, i) * 100 + random.nextInt(100));
} catch (InterruptedException ignored) {}
}
}
throw new RuntimeException("All retry attempts failed.");
}
}
Commentary
Here, a RetryHandler
is crafted to execute an action with a defined number of retries. The use of exponential backoff helps to distribute the load over time, thus preventing potential bottlenecks caused by immediate retries.
3. Bulkheads for Isolation
Bulkheads are a concept borrowed from shipbuilding where compartments are isolated to prevent flooding. Similarly, in software design, they prevent one failing component from affecting others.
Example Code Snippet
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
public class Bulkhead {
private final ExecutorService executorService;
public Bulkhead(int limit) {
executorService = Executors.newFixedThreadPool(limit);
}
public void execute(Runnable task) {
executorService.submit(task);
}
public void shutdown() {
executorService.shutdown();
}
}
Commentary
The Bulkhead
class restricts the number of concurrent tasks running. This can prevent resource exhaustion and ensure that critical systems remain operational during high-load conditions.
Monitoring and Observability
Effective monitoring of your applications can inform you about their health and performance. It consists of:
- Logging: Track events and errors.
- Metrics: Collect data on usage and performance.
- Alerting: Notify when certain thresholds are breached.
Utilizing tools like Prometheus for metrics and Grafana for visualization can greatly enhance your observability strategy.
The Last Word: Resilience is an Ongoing Journey
Mastering resilience in Java applications demands a proactive approach and continuous refinement. Adopting strategies like the circuit breaker, retries, and bulkheads will provide a robust foundation. Furthermore, leveraging monitoring tools will help you stay ahead of potential issues before they become critical.
Resilience in software is akin to the preparedness strategies highlighted in Surviving Chaos: Key Lessons from Netflix's Preppers. Just as these individuals equipped themselves to navigate tumultuous situations, developers must prepare their applications to face the challenges posed by real-world usage.
For further reading, explore more about chaos management in software development through the insights shared in Surviving Chaos: Key Lessons from Netflix's Preppers.
By following these strategies and continually evaluating your systems, you will enhance your applications' resilience and ensure they thrive amid chaos.
Checkout our other articles