Mastering Jenkins for Smooth Java Deployment in the Cloud
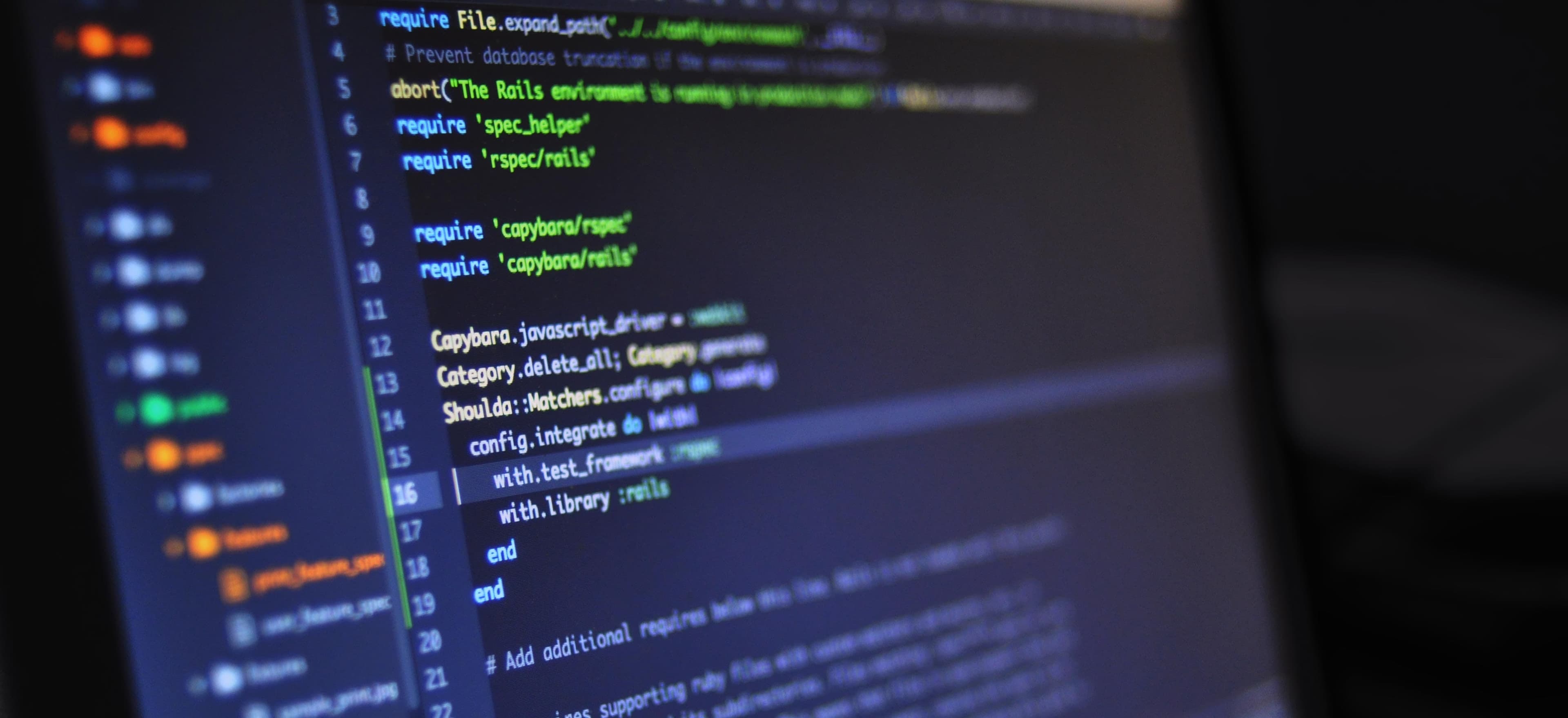
- Published on
Mastering Jenkins for Smooth Java Deployment in the Cloud
In today's rapidly evolving tech landscape, continuous integration and deployment (CI/CD) are pivotal in delivering high-quality software quickly and reliably. Among the various tools available, Jenkins stands out as a powerful automation server, especially for Java developers. This blog post will guide you through mastering Jenkins to achieve a seamless Java deployment process in the cloud.
Understanding Jenkins and Its Role in Java Development
Jenkins is an open-source automation server that aids in building, testing, and deploying software applications. With its extensive plugin ecosystem and integration capabilities, Jenkins enables developers to implement CI/CD pipelines for automated workflows.
Advantages of Using Jenkins for Java Deployment
- Scalability: Jenkins supports the deployment of applications on various cloud platforms, which is crucial for handling increased traffic demand.
- Flexibility: With numerous plugins, Jenkins can be tailored to fit any project requirement, ensuring smooth operations in diverse environments.
- Community Support: Being open-source, Jenkins has a vibrant community that contributes to improving features and offering support to users.
Setting Up Jenkins for Java Deployment
Before diving into the Jenkins configurations, you need to ensure that you have Java and Jenkins installed on your machine. You can refer to the official Jenkins installation documentation for detailed steps.
Step 1: Install Required Plugins
Jenkins' strength lies in its plugins. Installing the right plugins streamlines Java deployment efforts significantly. Start by installing:
- Git: For source code management.
- Maven Integration: Helps in building Java projects using Maven.
- Pipeline: Allows you to define your deployment pipeline as code.
Navigate to Jenkins' dashboard, go to Manage Jenkins > Manage Plugins
, and search for the above plugins to install them.
Step 2: Create Your First Job
A pipeline in Jenkins represents your automation process. Here's how to create your first pipeline job:
- On the Jenkins dashboard, select
New Item
. - Enter a name for your job and select
Pipeline
. - Click
OK
to proceed.
Step 3: Defining the Pipeline
In this section, we will define a Jenkins pipeline using a simple declarative syntax. Below is a basic example you can use and expand upon:
pipeline {
agent any
stages {
stage('Clone Repository') {
steps {
git 'https://github.com/yourusername/your-java-repo.git'
}
}
stage('Build') {
steps {
sh './mvnw clean package'
}
}
stage('Deploy') {
steps {
sh 'your-deployment-script.sh'
}
}
}
}
Code Commentary
-
agent any: This directive tells Jenkins to run the pipeline on any available agent. It can be customized based on specific requirements.
-
stage('Clone Repository'): This stage clones the source code from the specified Git repository. It's essential to have the correct permissions set for the repository.
-
stage('Build'): This stage uses Maven to clean and package the application. The use of
./mvnw
ensures that users always utilize the project’s specific Maven version, making the build process consistent across different environments. -
stage('Deploy'): The final stage executes a shell script that you define for deployment. This could include transferring files to a server, starting a service, etc.
Configuring Jenkins for Cloud Deployment
In recent years, deploying applications to the cloud has become a widely adopted practice. Various cloud platforms (like AWS, Azure, and Google Cloud) integrate seamlessly with Jenkins to facilitate continual deployment.
Step 1: Connect to Your Cloud Provider
Jenkins provides plugins for various cloud platforms, allowing you to link your Jenkins instance with your cloud provider of choice. For example, if you're deploying to AWS, install the AWS Steps Plugin.
Step 2: Create a Cloud Credentials
- Go to
Manage Jenkins > Manage Credentials
. - Click on
(global) > Add Credentials
. - Fill in the necessary fields for your cloud credentials.
Step 3: Modify Your Deployment Stage
You may modify your deployment stage in the Jenkins pipeline to incorporate cloud commands. An example for AWS deployment might look like:
stage('Deploy') {
steps {
sh 'aws s3 cp target/your-app.jar s3://your-bucket-name/your-app.jar'
sh 'aws lambda update-function-code --function-name your-function-name --s3-bucket your-bucket-name --s3-key your-app.jar'
}
}
Code Commentary
- aws s3 cp: This command uploads your packaged JAR file to an S3 bucket, thus enabling easy access during deployment.
- aws lambda update-function-code: This line updates the AWS Lambda function with the new application version from the previously uploaded S3 object.
Efficient Monitoring and Error Handling
With Jenkins, the job monitoring feature is invaluable. Implement notifications to alert your team about the job’s status:
post {
success {
mail to: 'team@example.com',
subject: "Successfully Deployed ${env.JOB_NAME} Build #${env.BUILD_NUMBER}",
body: "Check out the changes at ${env.BUILD_URL}"
}
failure {
mail to: 'team@example.com',
subject: "Failed to Deploy ${env.JOB_NAME} Build #${env.BUILD_NUMBER}",
body: "Please check the logs at ${env.BUILD_URL}"
}
}
Code Commentary
- post: This section allows you to define actions that occur after the pipeline's execution.
- mail: Set this up to automatically notify your team about successful jobs or failures, ensuring immediate attention if something goes wrong.
Troubleshooting Common Jenkins Configuration Challenges
While Jenkins is a powerful tool, configuration issues can arise—especially in cloud deployments. Many developers face challenges while setting up Jenkins in the cloud. You can find insights into addressing these hurdles in the article titled Overcoming Jenkins Configuration Challenges in the Cloud.
Lessons Learned
Mastering Jenkins for Java deployment in the cloud is not just about knowing how to set up pipelines; it involves understanding how Jenkins can simplify and enhance your development workflow. By embracing Jenkins and its integration capabilities, you can deliver applications with confidence.
Whether you are a seasoned professional or new to CI/CD, the outlined strategies and best practices can elevate your deployment game and significantly improve productivity. Start implementing these techniques today and watch your Java deployment experience transform dramatically!
Checkout our other articles