Avoiding Emojis in Java: Class Names Matter for Readability
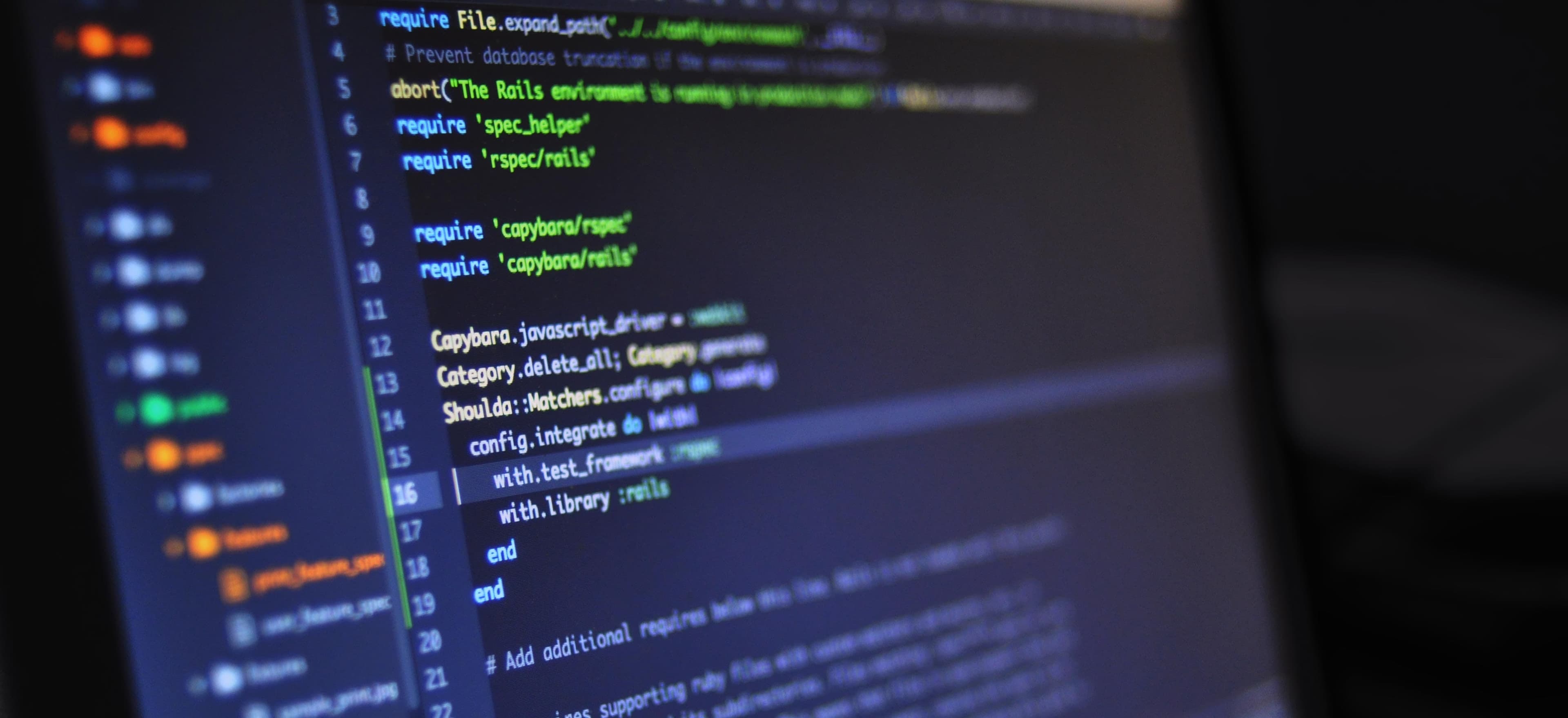
- Published on
Avoiding Emojis in Java: Class Names Matter for Readability
Java, a language revered for its portability and robustness, offers developers a clean and organized way to structure their code. However, as programming trends have evolved, some developers have entertained the idea of using emojis and other unconventional characters in their code. While this might seem like a light-hearted way to approach programming, it raises significant concerns, particularly in terms of readability and maintainability.
In this blog post, we will explore why using emojis in Java class names is detrimental to code quality, and why adhering to standard naming conventions is essential for clarity and professionalism. We will also delve into some best practices for class naming in Java, ensuring that your code remains understandable for you and your teammates.
The Importance of Class Names
Class names in Java play a crucial role in conveying the purpose and function of the code. A well-chosen class name can instantly inform a developer about what the class represents, the functionality it offers, and how it might interact with other components. For instance:
public class UserProfile {
private String username;
private String email;
// Constructors, getters, and setters
}
In this example, UserProfile
is clear and straightforward. Anyone reading this code can easily discern that the class is intended to represent a user's profile, enhancing overall understanding.
Now, consider a hypothetical class name adorned with emojis:
public class 😃UserProfile🎉 {
private String username;
private String email;
// Constructors, getters, and setters
}
At first glance, it may look entertaining. However, it jumps into a realm of confusion—especially for new developers or those unfamiliar with the project. As highlighted in the article "Why You Should Avoid Emojis in Class Names", using emojis can lead to misunderstanding and misinterpretation, making it harder for developers to collaborate effectively.
Readability Over Trendiness
While emojis may be trendy and visually appealing, they can also compromise readability:
-
Internationalization: Developers around the world speak various languages and may have different interpretations of what an emoji represents. Consequently, using emojis can lead to ambiguity.
-
Tooling Issues: Some IDEs, version control systems, or code formatting tools may not handle emojis properly. This can create errors or unexpected behavior during compilation.
-
Code Reviews: When colleagues review your code, anything that deviates from conventional practices can lead to misunderstandings or miscommunications.
Consider opting for meaningful names that reflect the class's role. Rather than 🌍LocationMapper
, default to LocationMapper
:
public class LocationMapper {
// Logic for mapping geographic locations
}
This method enhances clarity and keeps your code aligned with standard practice.
Naming Conventions: Best Practices
To foster clear communication through code, adhere to established Java naming conventions. Here are some best practices to guide you:
1. Use CamelCase
CamelCase is a practice where the first letter of each word is capitalized, and no spaces are included between words. This technique is essential for class names in Java:
public class OrderProcessor {
// Class implementation
}
2. Be Descriptive, Yet Concise
Your class names should clearly convey their purpose without being excessively verbose. Aim for clarity:
public class PaymentService {
// Service-related methods for processing payments
}
3. Avoid Ambiguity
Choose names that clearly indicate what the class is doing. Avoid generics like Helper
or Manager
, as they can apply to numerous scenarios:
public class EmailSender {
// Logic for sending emails
}
4. Use Nouns for Class Names
Classes typically represent entities or objects, making nouns the most suitable choices for naming:
public class Product {
// Product attributes and methods
}
5. Avoid Special Characters
Stick to alphanumeric characters and underscores where necessary. Refrain from using emojis, spaces, or punctuation:
public class ShoppingCart {
// Class implementation
}
ESLint and Code Linters
To ensure that your team adheres to established conventions, consider using code linters and formatters. Tools like ESLint can be configured to enforce naming conventions and catch potential issues early in the development cycle.
Example ESLint Configuration
While primarily used for JavaScript, the principles apply across programming contexts. Here’s a basic ESLint configuration for naming conventions:
{
"rules": {
"id-match": ["error", "^[a-zA-Z][a-zA-Z0-9]*$", {
"properties": true,
"onlyDeclarations": true,
"exceptions": ["^_"] // Allow leading underscore for private variables
}]
}
}
This rule enforces that variable and function names match the specified regex pattern, rejecting anything that contains emojis or special characters.
The Bottom Line
In the realm of Java development, code clarity and maintainability are of utmost importance. Avoiding emojis in class names is not merely a personal preference; it is a means of ensuring that code remains accessible, understandable, and professional. Stick to naming conventions that convey the intent of your classes and improve collaboration in your development team.
By adhering to best practices for class naming, you can foster a working environment that supports clear communication, comprehension, and productivity. For an in-depth discussion on why emojis in class names can negatively impact your projects, refer to the article "Why You Should Avoid Emojis in Class Names".
In summary, embrace the professionalism of traditional naming conventions and take the time to choose clear, descriptive names for your Java classes. Your future self, and your team, will thank you!
Checkout our other articles