Class Naming in Java: The Cost of Emoji Confusion
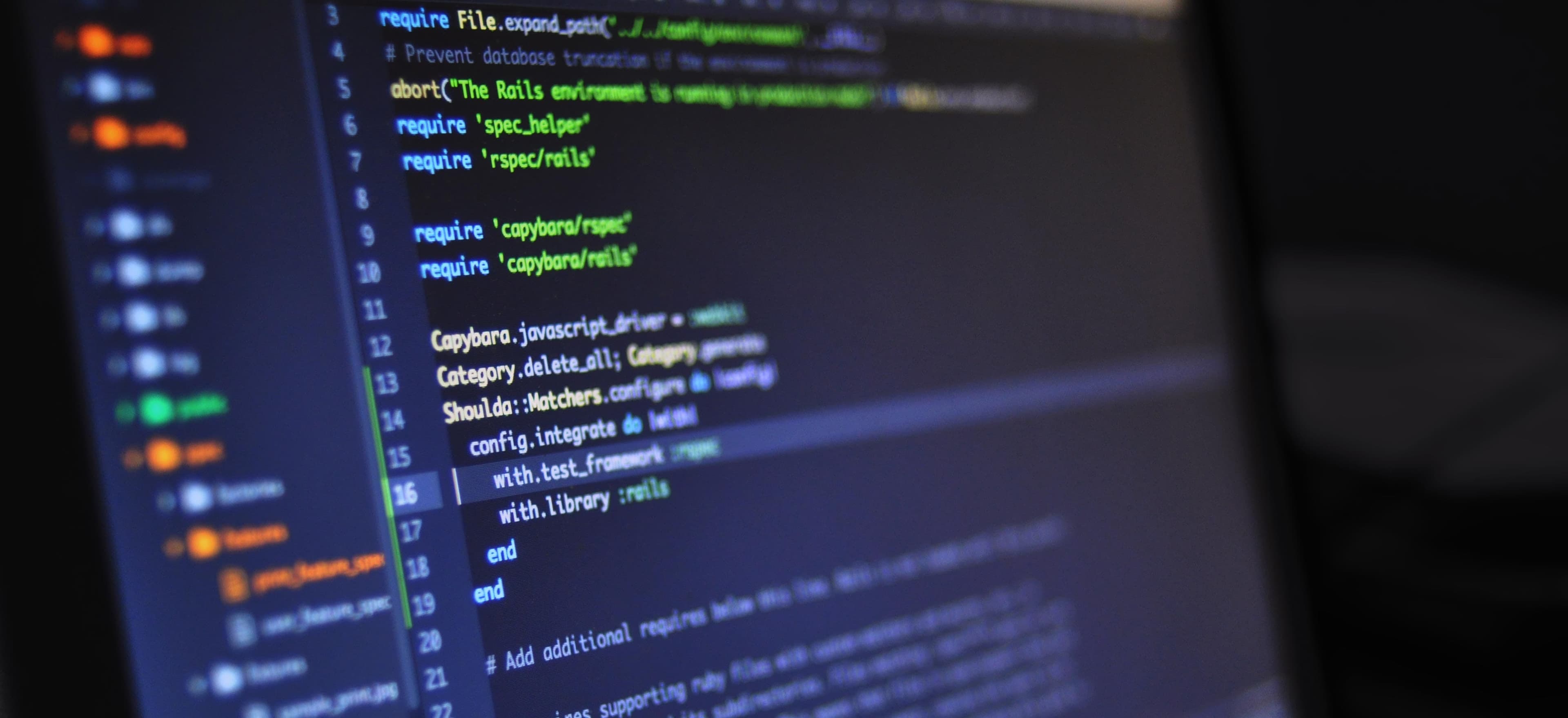
- Published on
Class Naming in Java: The Cost of Emoji Confusion
Class naming in Java might seem like a trivial concern at first glance. However, it plays a pivotal role in code readability, maintainability, and overall quality. One of the emerging debates revolves around the usage of emojis in class names. To shed light on this topic, we will reference an insightful article, "Why You Should Avoid Emojis in Class Names" (available at infinitejs.com/posts/avoid-emojis-class-names). This post explores the implications of such a choice and offers practical guidelines for effective class naming in Java.
The Essence of Good Class Naming
Why Class Names Matter
A well-named class provides immediate insight into its purpose or functionality. Here are some critical reasons why class naming is essential:
- Clarity: Clear names help others (and your future self) understand the code quickly.
- Maintainability: Descriptive names reduce the cognitive load for developers working on the codebase later.
- Convention: Following naming conventions enables better collaboration among team members.
For instance, consider the following contrasting examples:
Bad naming:
public class A {
// code logic
}
Good naming:
public class UserProfile {
// code logic
}
In the examples above, UserProfile
conveys explicit meaning compared to the generic A
.
The Case Against Emojis in Class Names
The Confusion Factor
Using emojis in class names may appear playful or modernized, but it introduces confusion. Let's break down the reasons:
- Readability: Not everyone interprets emojis in the same way, leading to misunderstandings.
- Tool Compatibility: Many tools and code analyzers may not handle emojis correctly, resulting in potential errors down the line.
Imagine you have a class named 😊HappyCustomer
. While it may evoke a sense of positivity, its function may be lost on anyone unfamiliar with emoji interpretations.
The Professional Standard
Java is a strongly typed language with a focus on professionalism. Introducing emojis can diminish the seriousness and impact of your code:
public class 😊HappyCustomer {
private String name;
public 😊HappyCustomer(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Critically, using an emoji here takes away from the straightforward understanding of this class. Instead, a more descriptive name could be HappyCustomer
.
public class HappyCustomer {
private String name;
public HappyCustomer(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
The Documentation Dilemma
Another crucial consideration is documentation. Documentation tools may struggle to render class names with emojis correctly, leading to ambiguities in API references. A clear naming strategy fosters proper documentation and consistent understanding among developers.
Emphasizing Conventions and Best Practices
Following Naming Conventions
Consistency is key in naming conventions. Here are some best practices:
- Use CamelCase for class names: Start each word with an uppercase letter.
- Keep names descriptive but concise: Aim for clarity while being brief.
- Avoid abbreviations and jargon unless widely recognized.
Example of a Convention:
public class CustomerService {
// code logic
}
Avoid Using Emojis in Place of Descriptive Names
Maintain professionalism by refraining from the whimsical use of emojis in class names. Instead, rely on descriptive words to capture the class's purpose effectively.
public class
Checkout our other articles