Seamlessly Integrating Product Images in Your Java App
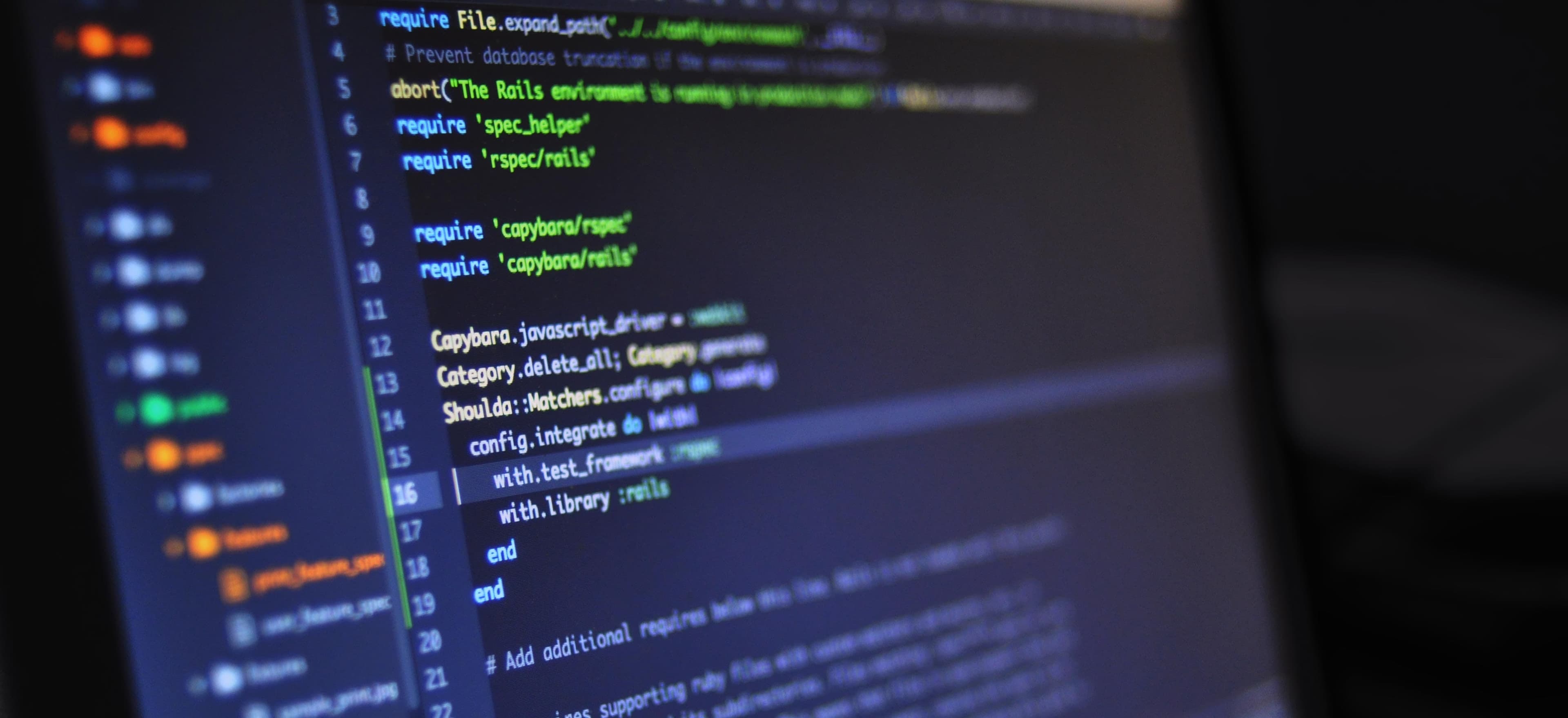
- Published on
Seamlessly Integrating Product Images in Your Java App
In today's digital world, the inclusion of images in applications is a necessity rather than a luxury. They serve as a visual representation of products, enhancing user experience and engagement. Now, when it comes to Java applications, incorporating images effectively can sometimes present a challenge. This blog post will guide you through the intricacies of integrating product images in your Java application, focusing on both the how and the why.
Why Use Images in Your Java Application?
Images can significantly enhance the user interface of your application. Not only do they help in better illustrating your product offerings, but they also help improve search engine optimization (SEO) by adding more value and context to your content.
Significance of Product Images
- Enhanced User Experience: High-quality images attract users and help them visualize what they are purchasing.
- Increased Conversion Rates: Stunning visuals can lead to higher conversion rates as users are more likely to engage with the product when it's visually appealing.
- SEO Benefits: Alt text and proper image descriptions contribute to SEO. Adding meaningful names to product images can enhance search engine visibility, as discussed in the article How to Effectively Add Product Names to Images.
Preparing Your Environment
Before diving into the code, you need to ensure your development environment is set up correctly. You'll need:
- Java Development Kit (JDK): Make sure you have the latest version installed.
- Integrated Development Environment (IDE): Eclipse, IntelliJ IDEA, or any preferred IDE for Java development.
Steps to Integrate Product Images
Step 1: Organize Your Image Resources
Keep your images organized in a specific directory within your project structure. This not only keeps your project tidy but also helps maintain a clear workflow when fetching and displaying images.
For example:
/project-root
/src
/resources
/images
product1.jpg
product2.png
Step 2: Load and Display Images
Java provides rich libraries for handling images. Below is a simple example of how to load and display images using the ImageIcon
class from the javax.swing
package.
import javax.swing.*;
import java.awt.*;
public class ImageDisplayExample extends JFrame {
public ImageDisplayExample() {
setTitle("Product Image Display");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Load Image
ImageIcon imageIcon = new ImageIcon("resources/images/product1.jpg"); // Path to your image
JLabel imageLabel = new JLabel(imageIcon);
// Add image to JFrame
add(imageLabel, BorderLayout.CENTER);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
ImageDisplayExample frame = new ImageDisplayExample();
frame.setVisible(true);
});
}
}
Code Commentary:
- JFrame: This is the window, and we are using it to hold the image.
- ImageIcon: A convenient way to load an image that can be used as an icon.
- JLabel: Works as a container that displays the image.
Step 3: Adding Alt Text and Captions
While Java Swing does not directly support alt text for images, you can create a caption manually utilizing a JLabel
. Here’s how to do it:
public void showImageWithCaption(String imagePath, String caption) {
ImageIcon image = new ImageIcon(imagePath);
JLabel imageLabel = new JLabel(image);
JLabel captionLabel = new JLabel(caption); // This will serve as the caption
JFrame frame = new JFrame();
frame.setLayout(new BorderLayout());
frame.add(imageLabel, BorderLayout.CENTER);
frame.add(captionLabel, BorderLayout.SOUTH);
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
Why Use Captions?
Captions serve multiple purposes:
- They provide context to the image.
- They can serve as additional SEO content if implemented in a web application.
Step 4: Responsive Images
Modern applications should prioritize responsiveness. In Java applications, you can use layout managers to adapt your image display across different window sizes. Here's how to use a GridBagLayout
to allow flexible positioning:
public ImageDisplayExample() {
setTitle("Responsive Product Image Display");
setLayout(new GridBagLayout()); // Using GridBagLayout for responsiveness
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ImageIcon imageIcon = new ImageIcon("resources/images/product1.jpg");
JLabel imageLabel = new JLabel(imageIcon);
GridBagConstraints gbc = new GridBagConstraints();
gbc.fill = GridBagConstraints.BOTH; // Fill both width and height
gbc.weightx = 1.0;
gbc.weighty = 1.0;
add(imageLabel, gbc);
}
Why Use a GridBagLayout?
- Flexibility: It allows you to define how components are resized or positioned.
- Control: You have granular control over component placement.
To Wrap Things Up
Integrating product images into your Java application is both straightforward and essential. From enhancing user experiences to boosting your SEO strategy, the significance of properly managed product images cannot be understated.
As you refine your application, always consider factors such as responsiveness, accessibility (through captions), and organization of your image assets. If you are curious to learn more about optimizing images for SEO and accessibility, check out the article How to Effectively Add Product Names to Images.
By following the steps outlined in this guide, you can ensure that your Java application's product images are presented in a professional, effective, and user-friendly manner. Keep learning, keep coding, and continue enhancing your applications!