Enhancing Java Web Apps with Dynamic Image Annotations
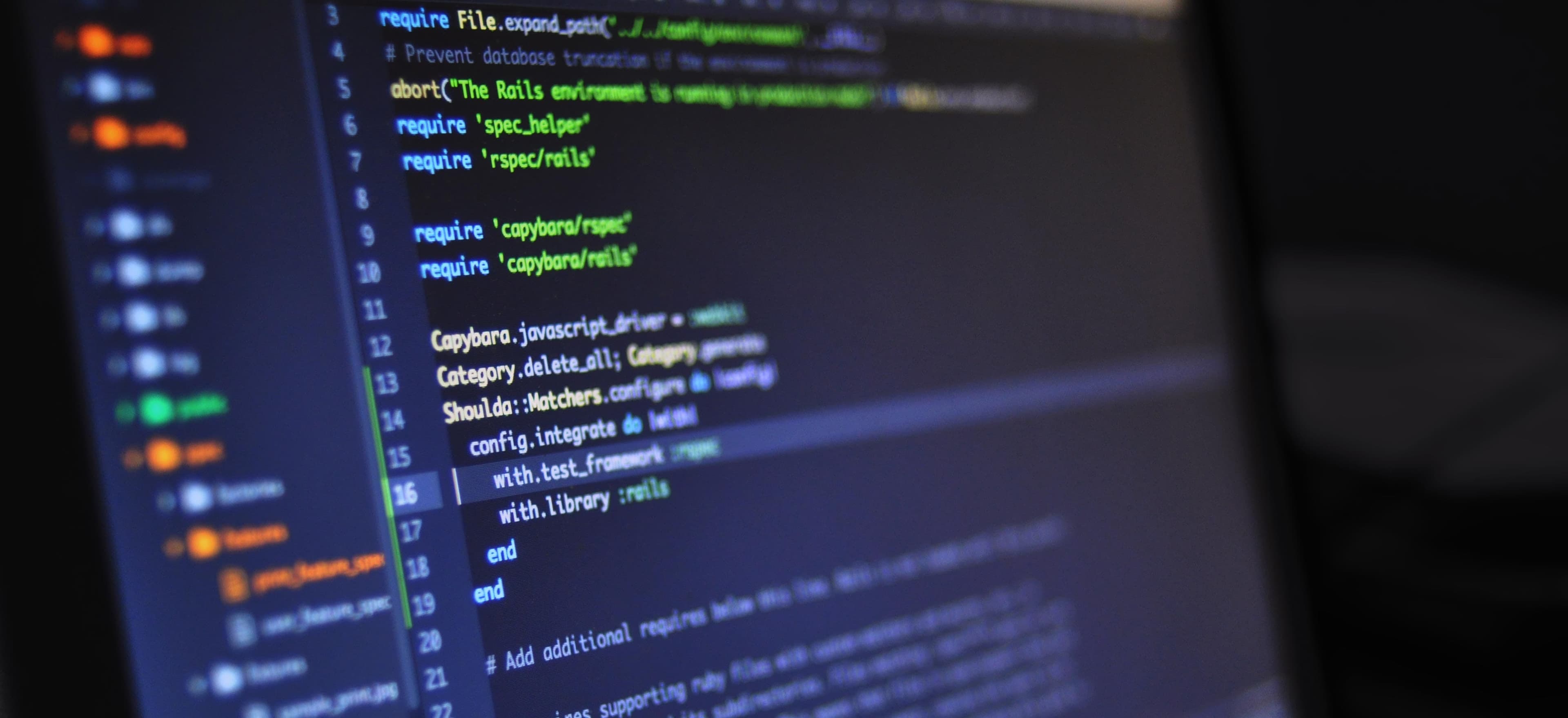
- Published on
Enhancing Java Web Apps with Dynamic Image Annotations
In the modern web landscape, creating engaging and interactive applications is paramount. Java, a versatile programming language, offers a solid foundation for developing dynamic web applications. One effective technique to enhance user experiences is through dynamic image annotations. This blog post will explore how to implement this feature in your Java web applications while focusing on the importance of user interaction and the practical use of annotations.
What Are Dynamic Image Annotations?
Dynamic image annotations are interactive overlays that provide additional information on images. This can take the form of text, graphs, or even links that pop up when a user hovers over or clicks on an image. This feature can enhance the usability and informational depth of web applications, making images not just visually appealing but also functional.
Why Use Dynamic Image Annotations?
- Improved User Engagement: Interactive content is proven to keep users on-site longer, which can lead to better conversion rates.
- Enhanced Information Delivery: You can convey more information without cluttering the user interface. This allows for a cleaner design while still being informative.
- Support for SEO: Adding relevant annotations can help search engines index your images better, improving your site’s search rankings.
Setting Up Your Java Web Application
Before we start implementing dynamic image annotations, ensure you have your Java web application set up with relevant dependencies. Here, we are using Spring Boot, Thymeleaf for templating, and a basic JavaScript library for dynamic functionality.
Prerequisites
- Java Development Kit (JDK) 11 or later
- Maven for dependency management
- Spring Boot framework
- A web browser for testing
Project Dependencies
First, you will need to add the following dependencies in your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.webjars</groupId>
<artifactId>jquery</artifactId>
<version>3.6.0</version>
</dependency>
</dependencies>
Sample Project Structure
Your project structure should resemble the following:
src/main/java/com/example/imageannotations
└── ImageAnnotationsApplication.java
src/main/resources/templates
└── index.html
Creating the Application
Initializing the Application
In ImageAnnotationsApplication.java
, set up your Spring Boot application:
package com.example.imageannotations;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ImageAnnotationsApplication {
public static void main(String[] args) {
SpringApplication.run(ImageAnnotationsApplication.class, args);
}
}
Adding a Basic Controller
Create a simple controller to serve your webpage:
package com.example.imageannotations.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class ImageController {
@GetMapping("/")
public String index(Model model) {
// Placeholder for dynamic content
model.addAttribute("imageSrc", "https://example.com/image.jpg");
model.addAttribute("annotations", "Dynamic Image Annotations Example");
return "index";
}
}
HTML Template with Image Annotations
Now, let’s create the index.html
file in the templates directory that utilizes Thymeleaf for dynamic data:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Dynamic Image Annotations</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
.annotation {
display: none;
position: absolute;
background-color: #fff;
border: 1px solid #ccc;
padding: 4px;
z-index: 10;
}
.image-container {
position: relative;
}
</style>
</head>
<body>
<h1>Dynamic Image Annotations</h1>
<div class="image-container">
<img th:src="${imageSrc}" alt="Sample Image" id="mainImage" width="600">
<div id="annotation" class="annotation"></div>
</div>
<script>
$(document).ready(function(){
$('#mainImage').hover(function(e){
$('#annotation').css({
top: e.pageY + 10 + 'px',
left: e.pageX + 10 + 'px'
}).text('[[${annotations}]]').show();
}, function(){
$('#annotation').hide();
});
});
</script>
</body>
</html>
Code Explanation
- HTML Structure: The HTML template sets up a basic structure with an image and a div for annotations.
- CSS Styles: The styles make sure the annotation is neatly positioned when it appears.
- JavaScript Logic: The jQuery code manages the hover events, showing and hiding the annotations. The use of
e.pageY
ande.pageX
allows the annotation to follow the mouse cursor.
Further Functionality
Now that you have a working example, let's explore adding more functionalities:
-
Multiple Annotations: Instead of a single annotation, you could create multiple annotations for different parts of the same image. This would involve dynamically setting the position and text based on user interactions over specified coordinates.
-
Interactivity: Consider enabling users to click on annotations to explore additional content or gather feedback, thereby creating a more interactive experience.
-
Loading Annotations from Database: You could connect to a database to fetch annotation data and display it dynamically.
Lessons Learned
Dynamic image annotations can significantly enrich your Java web applications by providing interactivity and enhancing user engagement. With the straightforward implementation demonstrated, you can now build upon this foundation.
If you’re interested in further enhancing your web application, check out the article "How to Effectively Add Product Names to Images" at tech-snags.com/articles/how-to-add-product-names-to-images for additional insights on improving image functionalities.
By utilizing these techniques, not only will your applications be user-friendly, but they will also stand out in today's competitive web development landscape. Here’s to creating engaging and dynamic applications with Java!
Checkout our other articles