Streamlining Switch Cases: Java Dropdowns Made Easy
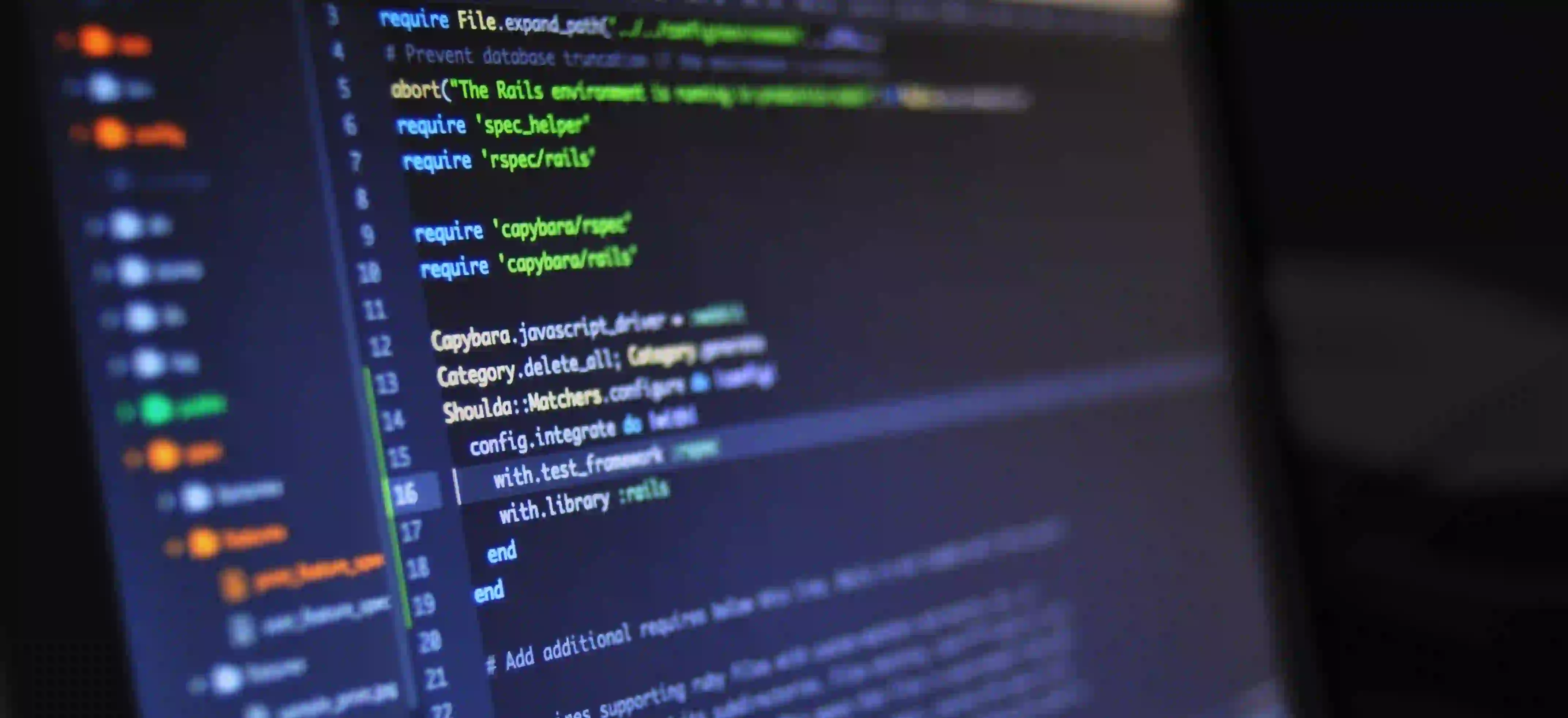
Streamlining Switch Cases: Java Dropdowns Made Easy
When developing applications in Java, handling user inputs through dropdowns is a common task. Dropdowns are integral to building user-friendly interfaces. They allow users to select from a predefined list of options, which streamlines the interaction and prevents errors from freetext inputs. However, developers often face challenges when it comes to extracting values from these dropdowns, particularly in relation to switch cases.
In this article, we will delve into effective strategies for managing switch cases tied to dropdown selections in Java. Additionally, we will explore how these methods can enhance code readability, maintainability, and performance. For a foundational understanding, refer to our previous article titled Mastering Dropdowns: Extracting Values from Switch Cases.
Understanding Switch Cases in Java
Switch cases allow developers to create cleaner and more efficient conditional statements. Instead of employing multiple if-else
constructs, a switch statement evaluates the value of a particular expression in a single call. Here's a basic example of how a switch case functions:
int day = 3;
String dayName;
switch (day) {
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
default:
dayName = "Invalid day";
}
System.out.println("The day is: " + dayName);
Why Use Switch Cases?
- Clarity: Switch statements are more organized than long chained
if-else
statements. They allow you to see all possible values in one place. - Performance: In some cases, switch statements can perform better than if-else chains, especially when the choices to be evaluated are numerous.
- Easier Maintenance: Adding a new case is straightforward, which simplifies future updates to your code.
Implementing Dropdowns in Java
In Java, dropdowns are commonly implemented using graphical user interface frameworks such as Swing, JavaFX, or AWT. In a Swing application, you can create a dropdown using the JComboBox
class.
Example: Creating a Simple Dropdown
Below is an example showcasing a simple dropdown in a Swing application that utilizes a switch case based on user selection:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class DropdownExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Dropdown Example");
String[] options = {"Option 1", "Option 2", "Option 3"};
JComboBox<String> dropdown = new JComboBox<>(options);
dropdown.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String selectedOption = (String) dropdown.getSelectedItem();
String message;
switch (selectedOption) {
case "Option 1":
message = "You selected Option 1.";
break;
case "Option 2":
message = "You selected Option 2.";
break;
case "Option 3":
message = "You selected Option 3.";
break;
default:
message = "No option selected.";
break;
}
JOptionPane.showMessageDialog(frame, message);
}
});
frame.add(dropdown);
frame.setSize(200, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Code Breakdown
- JComboBox: This class creates a dropdown element.
- ActionListener: By adding an ActionListener, you can perform actions when a dropdown option is selected.
- switch-case: The selected option values are evaluated in a switch case to determine the appropriate action.
This straightforward approach enhances user experience by providing immediate feedback based on their selection.
Enhancing the Dropdown Functionality
While the example above covers the basics, we can enhance our dropdown functionality using enums, which allow for better code practices and robustness. Enums can be combined with switch cases for a tidier approach.
Working with Enums
Enums provide a way to define a set of named constants. In the context of dropdowns, enums can be used to avoid hardcoding strings in your switch cases.
public enum Option {
OPTION_1("Option 1"),
OPTION_2("Option 2"),
OPTION_3("Option 3");
private final String displayName;
Option(String displayName) {
this.displayName = displayName;
}
public String getDisplayName() {
return displayName;
}
}
// Usage in JComboBox
String[] options = {Option.OPTION_1.getDisplayName(), Option.OPTION_2.getDisplayName(), Option.OPTION_3.getDisplayName()};
switch (Option.valueOf(selectedOption.toUpperCase().replace(" ", "_"))) {
case OPTION_1:
// Handle Option 1
break;
// Similarly for others...
}
Benefits of Using Enums
- Type Safety: Enums provide strong typing, minimizing the risk of errors when passing options.
- Better Readability: Each option can be self-documented with a meaningful name.
- Ease of Changes: Should a new option be needed, you can add it in one centralized location.
Final Considerations
Managing dropdowns in Java through switch cases doesn't need to be convoluted. By leveraging Swing for user interface creation and utilizing enums for clarity, you can streamline the process significantly.
In this article, we've explored key concepts and practical examples that make handling dropdowns an easier task. For further information and techniques on dropdowns, don't forget to check our previous article Mastering Dropdowns: Extracting Values from Switch Cases.
Whether you’re building a small desktop application or a large enterprise software solution, applying these strategies can lead to cleaner, more efficient, and maintainable Java code. Happy coding!