Simplifying Dropdown Handling with Java Switch Cases
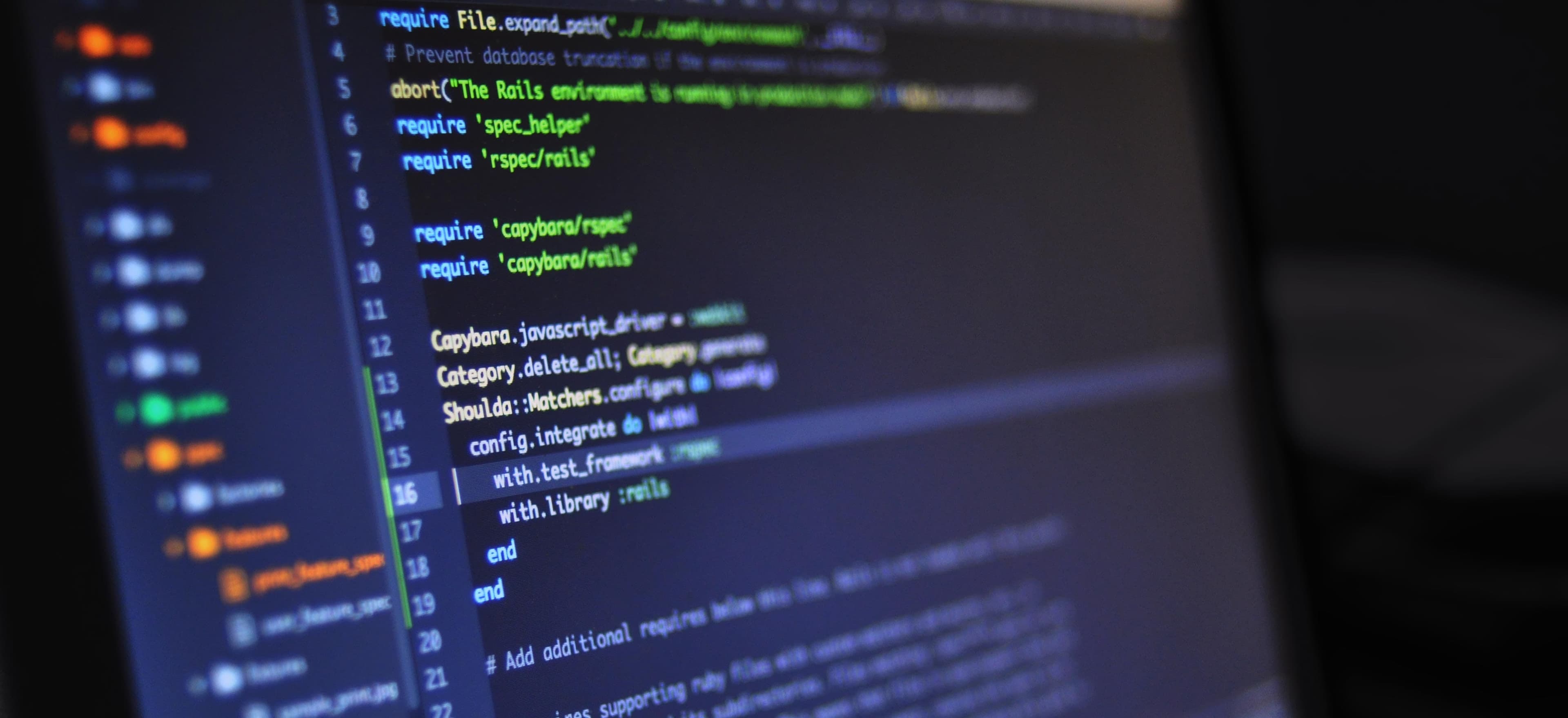
- Published on
Simplifying Dropdown Handling with Java Switch Cases
Handling dropdowns in Java applications can often feel like navigating a maze. However, with the right tools and techniques, you can streamline this process. One such method that has proven efficient is using switch cases for dropdown value extraction. If you are looking to refine your approach, consider the integration of Java switch statements into your dropdown handling logic.
In this blog post, we will explore how to simplify managing dropdowns in Java applications using switch cases. We will build on the principles discussed in the article "Mastering Dropdowns: Extracting Values from Switch Cases", providing you with an engaging and practical guide.
What Are Dropdowns?
Dropdowns, or dropdown lists, are UI elements that allow the user to select one value from a predefined list. They are commonly used in forms, configurations, and other applications requiring user input. When a user makes a selection, the application should react accordingly, often executing several lines of code for each possible choice.
Why Use Switch Cases?
When dealing with multiple conditions, the traditional if-else
structure can become cumbersome. Here are some benefits of using switch cases:
- Readability: A switch case makes your code more organized and understandable at a glance.
- Maintainability: Adding or modifying cases is more straightforward.
- Performance: In certain scenarios, switch cases can be optimized more effectively than multiple
if-else
statements.
Switch cases can simplify the logic of dropdown handling, especially when managing numerous selections.
Basic Syntax of Switch Cases in Java
Before diving into implementation, let’s recap the basic syntax of a switch case in Java:
switch (expression) {
case value1:
// Code block for value1
break;
case value2:
// Code block for value2
break;
// More cases
default:
// Default block
}
Why This Matters: Each case represents a potential value of the dropdown, allowing you to execute specific code for that value without cluttering your logic.
Example: Dropdown Handling with Switch Cases
Let's assume you are creating a simple program that converts units of measurement. The user can select a unit from a dropdown, and the application will perform the conversion.
Step 1: Setup the User Interface
For simplicity, we’ll focus on the logical handling part here. In a real-world application, this would typically be integrated into a GUI framework.
Step 2: Dropdown Value Extraction with Switch Case
Here's a code snippet demonstrating how to handle dropdown selections using a switch case:
public class UnitConverter {
public static void convertUnit(String selectedUnit, double value) {
switch (selectedUnit) {
case "Meters to Kilometers":
System.out.printf("%.2f Meters = %.5f Kilometers%n", value, value / 1000);
break;
case "Kilograms to Grams":
System.out.printf("%.2f Kilograms = %.2f Grams%n", value, value * 1000);
break;
case "Liters to Milliliters":
System.out.printf("%.2f Liters = %.2f Milliliters%n", value, value * 1000);
break;
default:
System.out.println("Selected unit conversion is not supported!");
}
}
public static void main(String[] args) {
// Example use-case, in a real scenario this would come from dropdown selection
convertUnit("Meters to Kilometers", 1500);
convertUnit("Kilograms to Grams", 2);
convertUnit("Liters to Milliliters", 1);
convertUnit("Feet to Inches", 1); // Unsupported case
}
}
Commentary
In the convertUnit
method, we employ a switch case to handle unit selections. Each case contains a relevant conversion calculation based on the selected unit. If none of the cases match the input, the default block executes, providing feedback to users about unsupported selections.
Key Takeaways
- Clarity: The switch statement is much clearer than a series of
if-else
statements. - Scalability: You can easily add more conversions as needed, simply by adding more cases.
When to Use Switch Cases over If-Else
While switch cases offer clear advantages, they aren't always the best choice. Use switch cases when:
- You are evaluating the same variable.
- The variable holds a limited number of discrete values (like a dropdown).
- Performance is a concern, and you need to optimize for numerous conditions.
On the other hand, if you have ranges of values or complex conditions, stick with if-else
statements.
To Wrap Things Up
Using switch cases to simplify dropdown handling in Java applications is an effective technique to improve readability and maintainability. Whether you are building a unit conversion tool or a more complex application, switch cases can streamline your decision-making logic.
If you want to delve deeper into dropdown handling, consider reading "Mastering Dropdowns: Extracting Values from Switch Cases" for more detailed insights and best practices.
By embracing structured yet straightforward code, you'll not only enhance your applications but also elevate your coding proficiency. Remember, simplicity is key to effective software development. Happy coding!
Checkout our other articles