Simplifying Dropdown Value Extraction with Java Switch Cases
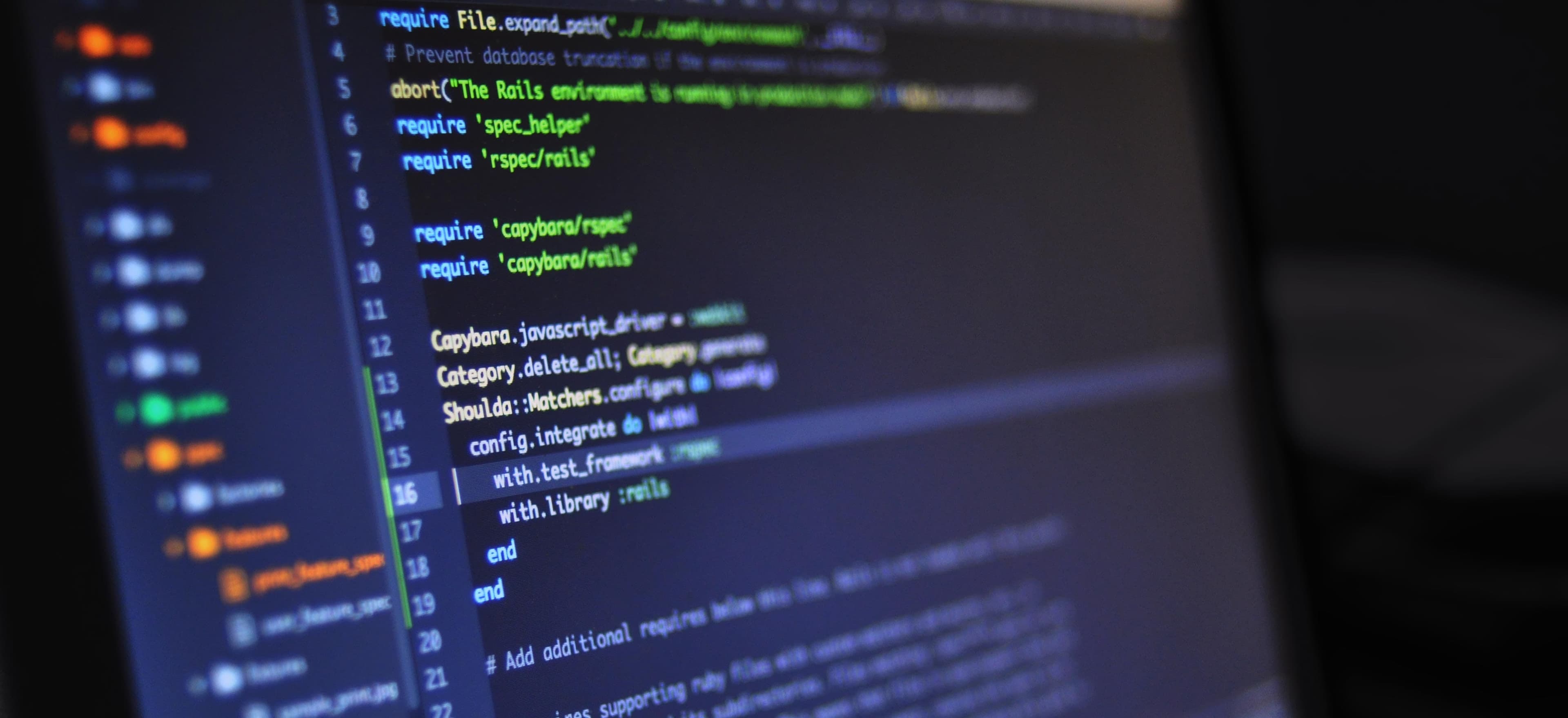
- Published on
Simplifying Dropdown Value Extraction with Java Switch Cases
Dropdown menus are a common user interface element that allows users to select from a list of options efficiently. When building applications in Java, handling dropdown selections effectively is crucial for providing a smooth user experience. A prevalent approach to manage dropdown values in Java is through the use of switch cases. In this blog post, we will explore simplified techniques for extracting values from dropdowns using Java switch cases, enhancing code readability and maintainability.
Why Use Switch Cases?
Switch cases provide a clean and efficient way to execute different blocks of code based on the value of a variable. Compared to multiple if-else
statements, switch cases enhance readability and can reduce the chance of error in complex decision-making processes.
For more insights into dropdown management in Java, I highly recommend checking out Mastering Dropdowns: Extracting Values from Switch Cases.
Understanding the Basics
In Java, a dropdown typically translates to an object with several options. When the user makes a selection, we want to execute specific logic based on that selection:
- Creating the Dropdown: You will typically use a UI library (e.g., JavaFX, Swing) to create your dropdown menu.
- Using the Selection: The switch case structure will allow you to handle the selected item accordingly.
Example: Using Switch Cases with a Dropdown
Let’s consider a simple scenario where we have a dropdown for selecting a fruit, and we want to print a message based on the selection.
Setting Up the Environment
Before diving into the code, let's make sure your environment can run Java Swing applications:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class FruitSelector {
public static void main(String[] args) {
JFrame frame = new JFrame("Fruit Selector");
String[] fruits = {"Apple", "Banana", "Cherry", "Date"};
JComboBox<String> fruitComboBox = new JComboBox<>(fruits);
fruitComboBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String selectedFruit = (String) fruitComboBox.getSelectedItem();
handleFruitSelection(selectedFruit);
}
});
frame.add(fruitComboBox);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
private static void handleFruitSelection(String fruit) {
switch (fruit) {
case "Apple":
System.out.println("You selected an Apple.");
break;
case "Banana":
System.out.println("You selected a Banana.");
break;
case "Cherry":
System.out.println("You selected a Cherry.");
break;
case "Date":
System.out.println("You selected a Date.");
break;
default:
System.out.println("Unknown selection.");
}
}
}
Code Commentary
- Creating the GUI: We set up a simple JFrame that hosts a JComboBox for the dropdown selection.
- Event Handling: We attach an
ActionListener
to the JComboBox to listen for user interactions. When a selection is made, thehandleFruitSelection
method is invoked with the selected item. - Switch Case Logic: Inside the
handleFruitSelection
method, we use a switch case to check the selected fruit. This structure is both intuitive and easy to expand with new cases if we need to add more fruits.
Advantages of This Approach
- Readability: The switch statement is clearer than a long chain of
if-else
statements. - Maintainability: Adding or modifying cases is straightforward and less prone to errors.
- Performance: In some scenarios, especially with larger datasets, switch cases can be more efficient than multiple
if-else
checks.
Adding Complexity: Handling Multiple Values
Let’s say you want to allow users to select multiple fruits. You may consider using a Set
to store selections and manage cases accordingly.
Modified Example for Multiple Selection
We’ll adjust our logic to handle multi-value selections. Let’s enhance our previous example:
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.HashSet;
import java.util.Set;
public class MultiFruitSelector {
public static void main(String[] args) {
JFrame frame = new JFrame("Multi Fruit Selector");
String[] fruits = {"Apple", "Banana", "Cherry", "Date"};
JComboBox<String> fruitComboBox = new JComboBox<>(fruits);
// Enable single selection for simplicity. Modify as needed for multiple selections.
fruitComboBox.setEditable(false);
Set<String> selectedFruits = new HashSet<>();
fruitComboBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String selectedFruit = (String) fruitComboBox.getSelectedItem();
selectedFruits.add(selectedFruit);
handleFruitSelections(selectedFruits);
}
});
frame.add(fruitComboBox);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
private static void handleFruitSelections(Set<String> fruits) {
for (String fruit : fruits) {
switch (fruit) {
case "Apple":
System.out.println("You selected an Apple.");
break;
case "Banana":
System.out.println("You selected a Banana.");
break;
case "Cherry":
System.out.println("You selected a Cherry.");
break;
case "Date":
System.out.println("You selected a Date.");
break;
default:
System.out.println("Unknown selection.");
}
}
}
}
Code Commentary
Unlike the simple example, this one allows the user to manage multiple selections. The core enhancements involve:
- Using a Set: We store the selections in a
HashSet
, ensuring no duplicates while providing amortized constant time complexity for add operations. - Iterating Through Selections: The
handleFruitSelections
method now iterates through each selected fruit, applying the switch logic to each one.
Best Practices for Dropdown Handling
- Provide Default Values: Always initialize your dropdown with a default or placeholder value.
- Validate Selections: Ensure that the selected values are valid before proceeding with further logic.
- Consider UI/UX: Ensure your dropdown options are relevant to the user context, and avoid overloading them with choices.
In Conclusion, Here is What Matters
Using switch cases to extract values from dropdown menus in Java effectively simplifies your code and enhances maintainability. The real power of this approach lies in its ability to handle multiple scenarios in a clear and structured manner. Whether you're maintaining a simple application or developing a complex system, this technique can significantly boost your development productivity.
For additional insights into managing dropdowns and switch cases in Java, don't forget to check out the insightful article Mastering Dropdowns: Extracting Values from Switch Cases.
Incorporating structured handling using Java's switch cases makes your application robust yet simple. Start integrating this invaluable technique into your projects and experience the improvements firsthand. Happy coding!
Checkout our other articles