Avoiding Java Integration Pitfalls in Your First Ansible Playbook
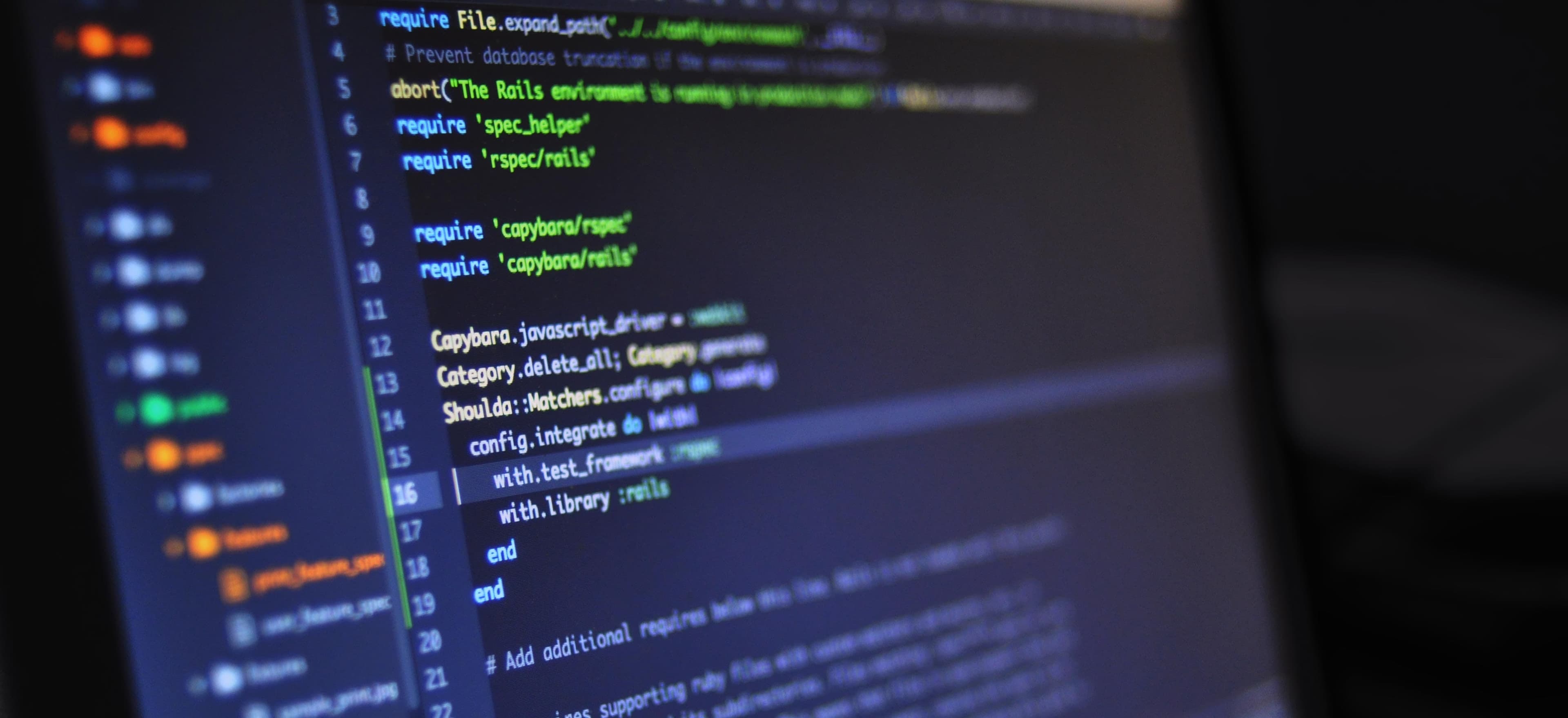
- Published on
Avoiding Java Integration Pitfalls in Your First Ansible Playbook
Java has long been a stalwart in the world of programming. Its robustness, scalability, and platform independence make it an ideal choice for application development. However, when integrating Java applications with Ansible—a powerful IT automation tool—developers often encounter challenges. This blog post aims to help you navigate those challenges and avoid common pitfalls, particularly as you embark on writing your first Ansible playbook.
Understanding Ansible and Its Relevance to Java
Ansible is an open-source automation platform that allows us to define and manage infrastructure as code. It can provision servers, deploy applications, and orchestrate complex workflows—all with a simple and human-readable language.
Why is this significant for Java developers? Most Java applications require a robust infrastructure for deployment, whether it’s configuring application servers or managing dependencies. Ansible simplifies these tasks and makes them repeatable. By leveraging Ansible, you can automate your Java deployments, reduce downtime, and enhance overall efficiency.
Common Pitfalls When Integrating Java with Ansible
1. Overlooking Java Environment Variables
One of the most critical aspects of running Java applications is ensuring that the correct environment variables are set. Many developers often forget to define these variables in their playbooks.
- name: Set up the Java environment
hosts: all
tasks:
- name: Ensure JAVA_HOME is set
lineinfile:
path: "/etc/profile"
line: "export JAVA_HOME=/usr/lib/jvm/java-8-openjdk-amd64"
state: present
Why This Matters: Without these environment variables, your Java application may fail to locate necessary libraries, resulting in runtime errors. Ensuring JAVA_HOME
, PATH
, and other environment variables are properly set can save you a headache down the line.
2. Ignoring Dependency Management
Java applications often depend on third-party libraries. If these dependencies are not managed correctly, your application may not run as expected. Ansible can help here.
- name: Install Maven dependencies
hosts: all
tasks:
- name: Ensure Maven is installed
yum:
name: maven
state: present
- name: Deploy Java application
shell: mvn clean install
args:
chdir: /path/to/your/project
Why This Matters: By using Maven (or Gradle), you can ensure that all your dependencies are resolved. This snippet not only installs Maven but also builds your Java application, ensuring that all dependencies are accounted for.
3. Failing to Handle Version Compatibility
Java applications may rely on specific versions of Java or other libraries. Failing to handle version compatibility can lead to application failure or bugs.
- name: Ensure correct Java version is installed
hosts: all
tasks:
- name: Install specific Java version
yum:
name: java-1.8.0-openjdk
state: present
- name: Verify the Java version
command: java -version
register: java_version_check
- debug:
var: java_version_check.stdout
Why This Matters: Ensure your playbook includes checks for Java versions and installs the required versions. This can prevent unexpected behavior and compatibility issues in production.
4. Neglecting Testing and Validation
Before deploying your application, it's crucial to test it. Ansible can execute test scripts to validate the deployment.
- name: Test Java application
hosts: all
tasks:
- name: Run application test suite
shell: mvn test
args:
chdir: /path/to/your/project
Why This Matters: Running tests as part of your deployment process ensures that your application is functioning as expected before it reaches the end user.
5. Inadequate Log Management
Logging is an essential part of maintaining Java applications. However, many developers overlook configuring proper log management in their playbooks.
- name: Configure log rotation
hosts: all
tasks:
- name: Set up log rotation for Java application
copy:
src: /path/to/logrotate.conf
dest: /etc/logrotate.d/java-app
Why This Matters: Setting up proper logging mechanisms helps in troubleshooting issues as they arise. Ansible can automate this setup for you, ensuring logs do not consume excessive disk space.
6. Using Incorrect Module Choices
Ansible has a wide array of modules that can simplify tasks. Using the wrong module can complicate the playbook unnecessarily.
Instead of running shell commands to manage services, leverage the built-in Ansible modules.
- name: Ensure the application service is running
hosts: all
tasks:
- name: Start Java application service
service:
name: your-java-app
state: started
Why This Matters: Using the appropriate modules increases clarity and reliability in your playbook, ensuring tasks are executed correctly.
Additional Resources
As you navigate the complexities of deploying Java applications with Ansible, it can be beneficial to understand common pitfalls in related tools. For excellent insights, refer to Common Pitfalls When Writing Your First Ansible Playbook. Understanding these challenges in broader contexts can help hone your skills.
A Final Look
Integrating Java applications with Ansible can streamline your deployment process, minimize errors, and enhance performance. However, pitfalls do exist. From environment variables to dependency management, being aware of these challenges can lead to more successful outcomes.
By following the practices outlined in this blog, you can avoid the common integration pitfalls. As you continue your journey with Ansible and Java, remember that meticulousness and testing are keys to successful automation.
Feel free to drop your queries or experiences in the comments below. Happy coding, and may your Ansible playbooks run smoothly!
Checkout our other articles