Avoiding Java Integration Pitfalls in Your First Ansible Playbook
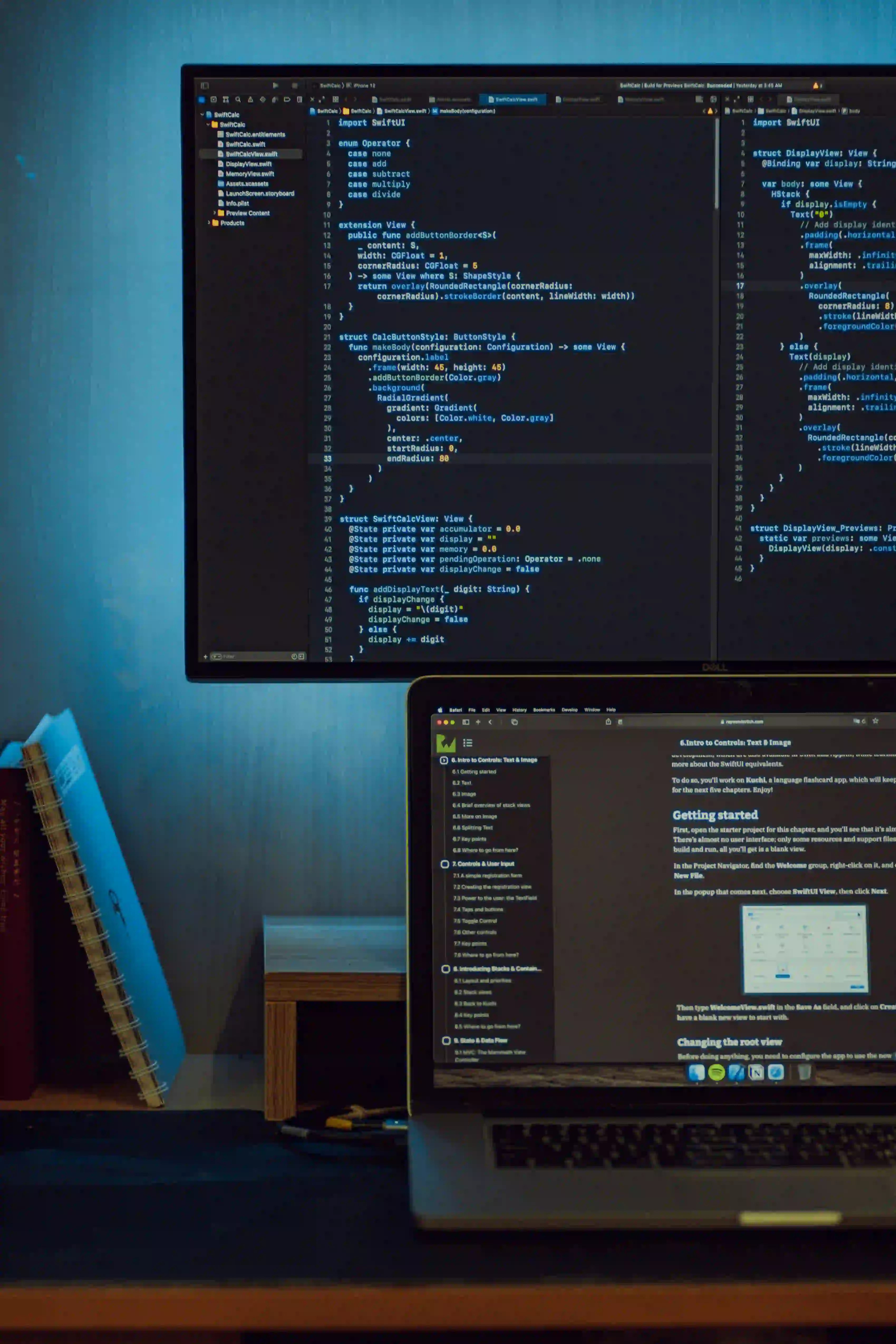
Avoiding Java Integration Pitfalls in Your First Ansible Playbook
As organizations move towards automating infrastructure management, Ansible has emerged as a robust tool that simplifies configuration management, application deployment, and task automation. However, for developers, integrating Java applications with Ansible can present its own set of challenges. This blog post explores how to avoid common pitfalls in Java integration when writing your first Ansible playbook, ensuring a smooth and efficient deployment process.
Understanding Ansible and Its Purpose
Before diving into the integration with Java, it's essential to understand the purpose of Ansible. Ansible is an open-source automation tool that allows you to define and manage the configuration of systems in an efficient and repeatable manner. It uses a declarative language to describe the system configuration and the tasks that need to be performed.
Why Use Ansible?
- Ease of Use: Ansible uses YAML for its playbooks, making it easy to read and write.
- Agentless: Unlike other automation tools, Ansible does not require any agent to be installed on the managed systems, using SSH and WinRM access instead.
- Idempotent: Ansible ensures that repeated executions of the same playbook will yield the same state, reducing errors and enhancing reliability.
The Importance of Java in Modern Applications
Java remains one of the most popular programming languages for building enterprise-level applications. Its portability across different platforms and robust ecosystem have made it a preferred choice. Integrating Java with Ansible is a smart move, especially in environments that leverage Java applications heavily.
Common Pitfalls in Ansible Playbooks
While Ansible provides developers with a powerful way to manage configurations, it is crucial to be aware of common pitfalls that may arise during the integration process, particularly when including Java applications.
1. Ignoring Dependency Management
When deploying Java applications, it is vital to manage dependencies effectively. A common mistake is to overlook the requirements in the pom.xml
file if you're using Maven.
Code Snippet: Using Ansible to install Java dependencies
- name: Install Java dependencies
hosts: java_servers
tasks:
- name: Install required Java packages
apt:
name: "{{ item }}"
state: present
loop:
- openjdk-11-jdk
- maven
Commentary: This playbook installs OpenJDK 11 and Maven on your target Java servers. Proper dependency management ensures your application loads its libraries correctly, avoiding runtime issues. Always double-check that required packages are outlined clearly.
2. Not Setting Environment Variables
Java applications often rely on specific environment variables, such as JAVA_HOME
and CLASSPATH
. Failing to set these variables can lead to issues during deployment.
Code Snippet: Setting environment variables for a Java application
- name: Set JAVA_HOME environment variable
hosts: java_servers
tasks:
- name: Ensure JAVA_HOME is set
lineinfile:
path: /etc/environment
line: 'JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64'
state: present
Commentary: This task ensures that the JAVA_HOME
variable is set correctly in your environment. Setting the right environment variables helps your Java applications locate the necessary libraries and resources during execution.
3. Hardcoding Values
Hardcoding values into your playbooks can create maintenance headaches down the line. Instead of using hardcoded values, leverage Ansible variables.
Code Snippet: Using variables in your Ansible playbook
- name: Deploy Java application
hosts: java_servers
vars:
app_name: my_java_app
app_version: 1.0.0
tasks:
- name: Download the Java application
get_url:
url: "http://example.com/{{ app_name }}-{{ app_version }}.jar"
dest: "/opt/{{ app_name }}/{{ app_name }}.jar"
Commentary: Utilizing variables makes your playbook dynamic and easily modifiable for different deployment scenarios. It promotes reusability and clarity.
4. Not Managing Configuration Files
Often, Java applications require specific configuration files. Not including these in your playbook can lead to issues in different environments.
Code Snippet: Managing configuration files
- name: Deploy application configuration
hosts: java_servers
tasks:
- name: Copy configuration file
copy:
src: config/application.properties
dest: /opt/my_java_app/config/application.properties
owner: java_user
group: java_group
mode: '0644'
Commentary: Ensuring that necessary configuration files are deployed with correct permissions is essential. This prevents permission-related issues when the application attempts to access its configuration during runtime.
5. Skipping Error Handling
Not incorporating error-handling mechanisms in your Ansible playbook can lead to cascading failures during deployment.
Code Snippet: Implementing error handling
- name: Deploy Java application with error handling
hosts: java_servers
tasks:
- name: Start Java application
command: java -jar /opt/my_java_app/my_java_app.jar
register: app_result
failed_when: app_result.rc != 0
- name: Print outcome of the application
debug:
msg: "Application started successfully"
when: app_result.rc == 0
Commentary: By registering command results and using conditions to handle errors, you maintain visibility on failed tasks. This keeps your playbook's execution under control and allows for easier troubleshooting.
Bringing It All Together
As you embark on writing your first Ansible playbook for Java application integration, remember to avoid these common pitfalls. Proper dependency management, setting environment variables, utilizing variables, managing configurations, and implementing error handling are critical steps to ensure successful automation.
For more insights into automation pitfalls, consider checking out Common Pitfalls When Writing Your First Ansible Playbook. This reference discusses additional common challenges and how to mitigate them.
By adopting best practices, you can create robust and reliable Ansible playbooks that streamline your Java application deployments, leading to a more efficient and error-free infrastructure environment. Happy automation!