Mastering Array Conversion in Java: Handle User Input Errors
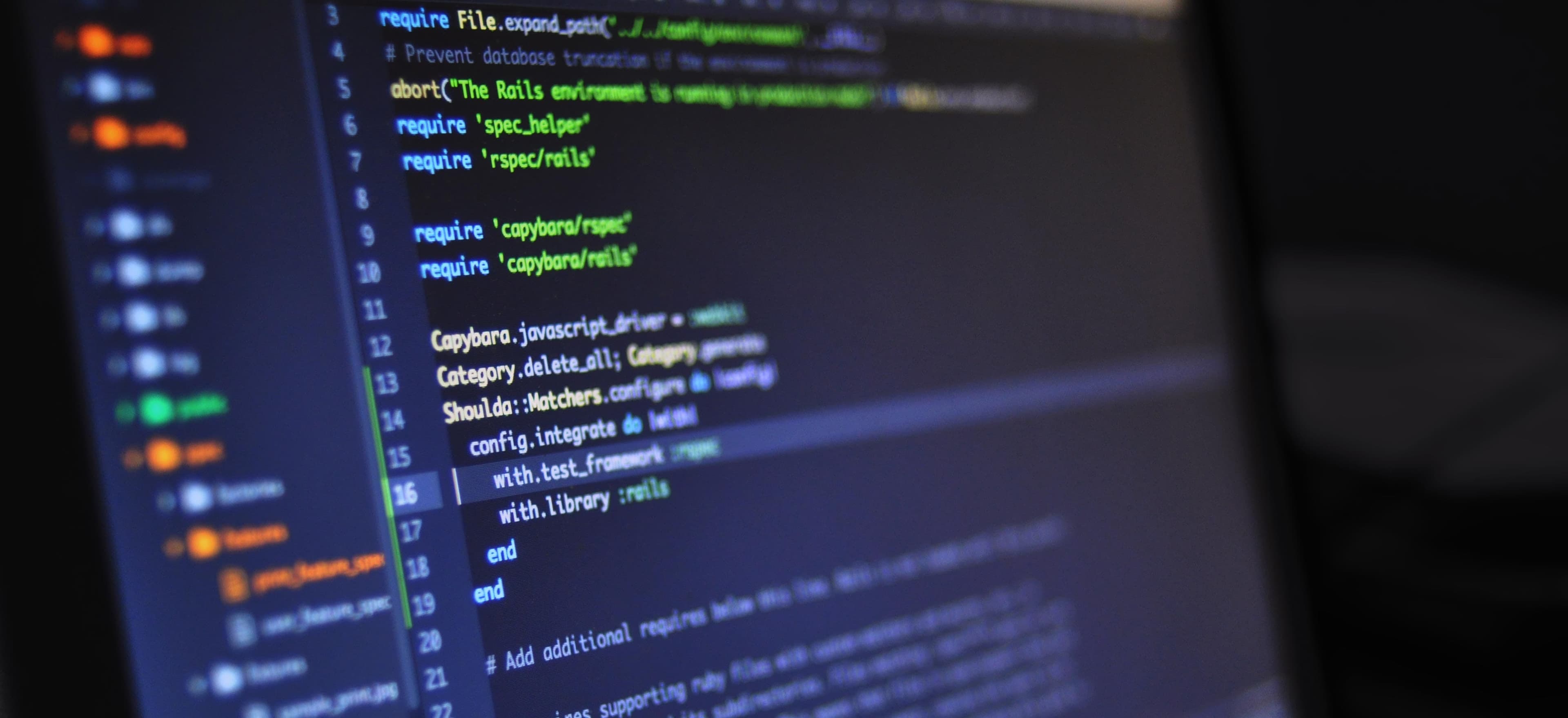
- Published on
Mastering Array Conversion in Java: Handle User Input Errors
Java is a powerful programming language that provides a rich set of features for managing data. Among these features, arrays stand out as a fundamental data structure that allows developers to store multiple values in a single variable. However, converting user input into an array can be challenging, especially when dealing with errors and exceptions that can arise during input processing.
In this post, we will explore the essential techniques for handling user input errors when converting it to an array in Java. We will also refer to a crucial resource, "Converting User Input to Array: A Step-by-Step Guide", that provides deeper insights into the conversion process.
Understanding the Basics of Arrays in Java
Before diving into error handling, let's briefly cover what arrays are in Java. An array is a container object that holds a fixed number of values of a single type.
Creating an Array
To declare an array in Java, you use the following syntax:
// Declare an array of integers
int[] numbers = new int[5]; // Creates an array that can hold 5 integers
Populating an Array
You can also populate an array directly upon declaration:
// Initialize an array
String[] fruits = {"Apple", "Banana", "Cherry"};
Arrays are zero-indexed, meaning the first element is accessed using index 0
.
The Importance of Handling User Input Errors
When receiving input from users, it's important to anticipate potential errors. Incorrect input formats, unexpected data types, and other common issues can cause your application to crash if not handled properly.
Consider a scenario where a user is prompted to enter a series of numbers to be stored in an array. If the user mistakenly enters a letter instead of a number, this will lead to a NumberFormatException
.
One way to handle this is to validate the input before attempting any conversion.
Step-by-Step Guide to Error Handling in Input Conversion
Step 1: Prompting for Input
Let’s start by writing a simple method to get user input. We’ll prompt the user to enter comma-separated numbers.
import java.util.Scanner;
public class InputHandler {
public static String getUserInput() {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter numbers separated by commas: ");
return scanner.nextLine();
}
}
Step 2: Converting Input to Array
Next, we will convert the input string into an array of integers. We will also add error handling to manage any non-numeric input gracefully.
public class ArrayConverter {
public static int[] convertToIntArray(String input) {
String[] inputArray = input.split(",");
int[] numbers = new int[inputArray.length];
for (int i = 0; i < inputArray.length; i++) {
try {
// Trim whitespace and parse integers
numbers[i] = Integer.parseInt(inputArray[i].trim());
} catch (NumberFormatException e) {
System.out.println("Error: '" + inputArray[i] + "' is not a valid number.");
// Assign a default value or choose to continue based on requirements
numbers[i] = -1; // Example default value
}
}
return numbers;
}
}
Commentary on the Code
- Splitting Input: We use
input.split(",")
to get individual number strings. - Parsing with Error Handling: Inside the loop, we attempt to convert each string to an integer with
Integer.parseInt()
. If it fails, we catch the exception and notify the user without crashing the program. - Graceful Degradation: In case of an error, we assign a default value (-1 in this case), but depending on your requirements, you could skip or ask the user to re-enter.
Step 3: Integrating Everything
Now that we have both input handling and conversion methods, we can integrate them into a main method.
public class Main {
public static void main(String[] args) {
String input = InputHandler.getUserInput();
int[] resultArray = ArrayConverter.convertToIntArray(input);
System.out.println("Converted Array:");
for (int number : resultArray) {
System.out.print(number + " ");
}
}
}
Step 4: Testing the Application
It's essential to test our application with various inputs, including edge cases such as empty strings or characters:
- Input: "1,2,3" → Output: Converted Array: 1 2 3
- Input: "1, A, 3" → Output: Converted Array: 1 -1 3
- Input: "" (empty string) → Output: Converted Array: -1 -1 -1
By implementing error handling, we prevent the application from crashing unexpectedly and improve user experience.
Additional Tips for Robust Input Handling
- Use Regular Expressions: To validate input format before conversion.
- Provide User Feedback: Use meaningful messages to guide the user through the process.
- Consider Edge Cases: Test with various inputs to ensure robustness.
For more comprehensive guidance on converting user inputs to arrays, check out the article "Converting User Input to Array: A Step-by-Step Guide".
To Wrap Things Up
Handling user input errors is essential for building robust Java applications. By utilizing careful input validation and error handling techniques, you can improve your application's resilience and ensure a better user experience. Remember that programming is not just about writing code but also about anticipating issues and preparing for them.
With the techniques discussed here, you're well on your way to mastering array conversion and error handling in Java. Happy coding!
Checkout our other articles