Java Solutions: Handling Application Errors to Ease Headaches
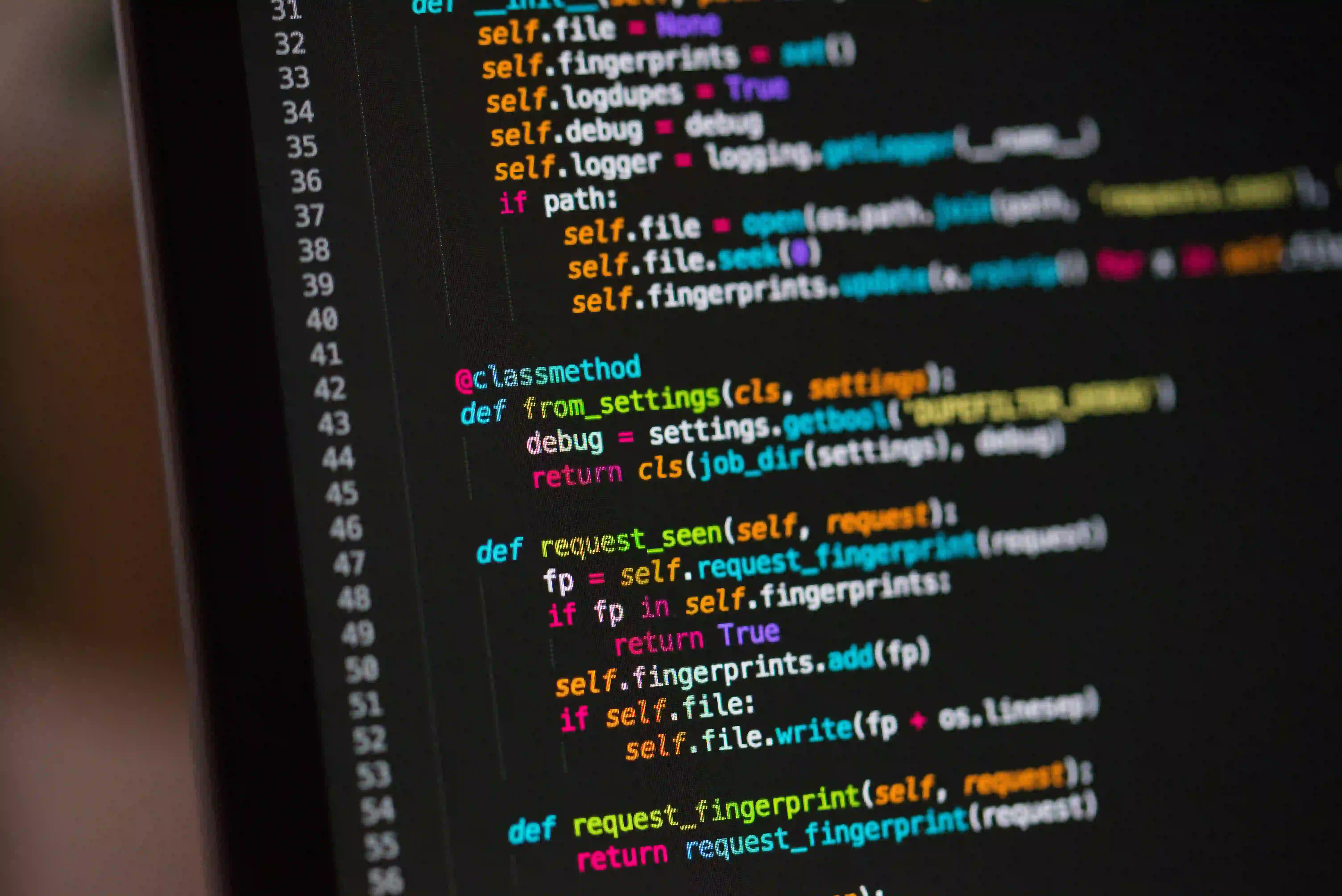
Java Solutions: Handling Application Errors to Ease Headaches
When developing applications in Java, one of the major challenges we face is managing errors effectively. Just like a physical headache might prompt you to reach for a remedy—be it a painkiller or a soothing cup of herbal tea—software errors require thoughtful solutions to minimize their impact on the user and the system.
In this article, we will delve into various Java error handling strategies, explore best practices, and provide code snippets to elevate your programming prowess. By the end, you’ll gain a comprehensive understanding of how to mitigate application errors, making your coding journey smoother and less headache-inducing.
Understanding Java Exceptions
Java uses a robust exception handling model that separates regular code from error-handling code. Exceptions are events that disrupt the normal flow of the program. They can be classified into checked exceptions, unchecked exceptions, and errors.
- Checked exceptions: These are exceptions the compiler requires you to handle. They usually represent recoverable conditions like
IOException
in file operations. - Unchecked exceptions: These are not required to be caught explicitly. They typically represent programming errors, such as
NullPointerException
. - Errors: These are serious issues that a reasonable application should not try to catch, such as
OutOfMemoryError
.
Why Use Exception Handling?
Effective exception handling is crucial because:
- It enhances code readability by separating the error-handling logic from regular business logic.
- It allows programmatic response to different types of errors, potentially leading to graceful degradation.
- It assists in debugging, as exceptions can carry detailed information about the error context.
The Exception Handling Blocks
Java provides several keywords for managing exceptions: try
, catch
, finally
, throw
, and throws
. Let's break this down using a code snippet.
public void readFile(String filePath) {
FileReader fileReader = null;
try {
fileReader = new FileReader(filePath);
// Simulate reading the file
int data = fileReader.read();
while (data != -1) {
System.out.print((char) data);
data = fileReader.read();
}
} catch (IOException e) {
System.err.println("An I/O error occurred: " + e.getMessage());
} finally {
try {
if (fileReader != null) {
fileReader.close();
}
} catch (IOException e) {
System.err.println("Failed to close the file reader: " + e.getMessage());
}
}
}
Explanation:
-
Try Block:
- The
try
block contains the code that may throw exceptions. - It's where we attempt the file reading operation.
- The
-
Catch Block:
- If an
IOException
occurs, the control goes to thecatch
block. - It captures the exception and allows you to log the error or notify the user.
- If an
-
Finally Block:
- The
finally
block runs regardless of whether an exception occurred, useful for resource cleanup (e.g., closing file readers).
- The
By implementing the above structure, we can elegantly manage exceptions and keep our applications running smoothly.
Custom Exceptions for a Cleaner Approach
In some cases, you may want to define your own exceptions to handle specific application errors more effectively. This allows your application to throw clear, meaningful errors while maintaining clean separation from Java’s built-in exceptions.
Here’s how to create a custom exception:
public class FileReadException extends Exception {
public FileReadException(String message) {
super(message);
}
}
To use your custom exception, modify the previous method:
public void readFile(String filePath) throws FileReadException {
try {
// File reading logic
} catch (IOException e) {
throw new FileReadException("Could not read the file: " + filePath);
}
}
Why Create Custom Exceptions?
- Clarity: Custom exceptions can convey specific error messages relevant to your business logic.
- Granularity: By throwing custom exceptions, you can provide more granular control and a tailored response to errors, improving debugging and user experience.
Best Practices for Exception Handling
-
Avoid Using Exception for Control Flow: Exceptions should be used for exceptional conditions only, not for controlling regular application flow.
-
Log Exceptions: Logging provides insight into problems as they occur in production. Use frameworks such as Log4j or SLF4J for robust logging solutions.
-
Provide User-Friendly Messages: While technical messages are useful for debugging, users should see clear, user-friendly messages that explain the problem without overwhelming them.
-
Do Not Catch General Exceptions: Avoid catching general exceptions (like
Exception
orThrowable
). This practice can hide bugs in your code and makes it harder to diagnose issues. -
Graceful Recovery: If possible, design your application to recover from exceptions. Simple failures shouldn’t always crash the application.
Bringing It All Together
With the strategies discussed, you are now equipped to handle exceptions effectively in your Java applications. By utilizing structured error handling, including custom exceptions, and adhering to best practices, you can minimize the headaches that come with application errors.
Like the article, "Natürliche Hilfe: 5 Teesorten gegen Kopfschmerzen," discusses natural remedies for headaches (found at auraglyphs.com/post/natuerliche-hilfe-5-teesorten-gegen-kopfschmerzen), handling exceptions can be viewed as employing remedies that ease the pain caused by unexpected problems in your code.
By treating exceptions thoughtfully, you improve both the resilience of your applications and the satisfaction of your users. Happy coding!