Resolving Type Errors During Java Data Imports
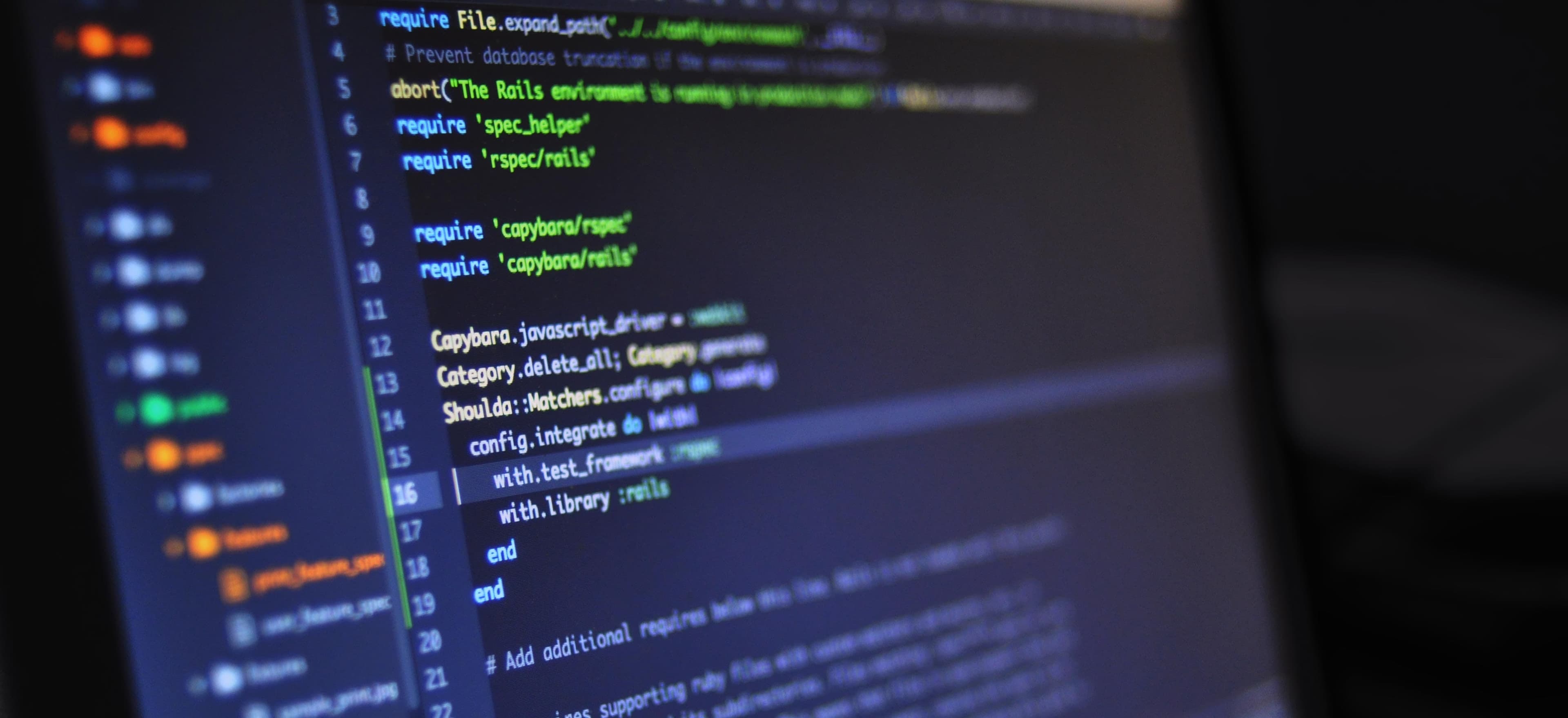
- Published on
Resolving Type Errors During Java Data Imports
Data import is a common task for Java developers, especially as applications rely on external data sources to function effectively. However, importing data is not always a straightforward process. Type errors can arise, complicating the import workflow and frustrating developers. In this blog post, we will explore common causes of type errors during Java data imports, best practices to prevent them, and practical solutions when they occur.
Understanding Type Errors
Type errors occur when there is a mismatch between the expected data type and the actual data type present in the data source. For instance, if a Java application expects an integer but receives a string representation of a number, it results in a type error.
Example of Type Error:
String data = "100"; // This is a String
int number = Integer.parseInt(data); // This works
number = Integer.parseInt("NotANumber"); // This will throw a NumberFormatException
Why Type Errors Matter
Type errors can lead to application crashes, incorrect data processing, or severe performance issues. For developers, addressing these issues swiftly is crucial for maintaining the integrity and reliability of the application.
Common Causes of Type Errors in Java Data Imports
-
Inconsistent Data Formats
- Data sources may not adhere to a consistent formatting standard, leading to discrepancies.
-
User Input Errors
- When users provide input, they may unintentionally input incorrect data types, especially in web forms.
-
Database Schema Changes
- If there are changes in the database schema without corresponding updates to the application, this can lead to type mismatches.
-
API Responses
- When consuming data from APIs, the response types may differ from expected types.
Best Practices to Avoid Type Errors
To prevent type errors during data imports in your Java applications, consider the following best practices:
1. Input Validation
Ensure that data is validated before being processed. Implementing robust input validation can catch errors before they lead to type mismatches.
Example Code for Input Validation:
public static Integer validateAndParseInt(String input) {
try {
return Integer.parseInt(input);
} catch (NumberFormatException e) {
System.err.println("Invalid input for Integer parsing: " + input);
return null; // or handle it as needed
}
}
// Usage
Integer number = validateAndParseInt("123");
2. Use Type-Safe Libraries
Utilizing type-safe libraries can reduce the risk of type errors. Libraries such as Apache Commons Lang and Guava can provide utility methods that encourage safe type conversions.
3. Consistent Data Representation
Ensure that your application and external data sources agree on data formats. For example, if your application expects a date in the format YYYY-MM-DD, ensure that this convention is maintained throughout the application and any APIs it interacts with.
Diagnosing Type Errors During Data Imports
When errors do occur, diagnosing them promptly is essential. Here are a few steps to identify type errors effectively:
1. Logging
Incorporate logging to track data flow through your application. Use logging frameworks like Log4j or SLF4J to log the data being imported and any related errors.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DataImporter {
private static final Logger logger = LoggerFactory.getLogger(DataImporter.class);
public void importData(String data) {
logger.info("Importing data: {}", data);
// Import logic here
}
}
2. Debugging Tools
Use debugging tools integrated within IDEs like Eclipse or IntelliJ IDEA to step through the code. This can help isolate where type mismatches occur.
3. Stack Trace Analysis
When errors occur, analyzing stack traces can provide invaluable insights into the root cause. Understanding where the error originated can guide you toward a resolution.
Fixing Common Type Errors
Below, we discuss common type errors and provide examples of how to fix them.
1. Number Format Exception
This occurs when converting a non-numeric string to a number. You can use the example provided earlier: validateAndParseInt.
2. Class Cast Exception
This error arises when attempting to cast an object to a class of which it is not an instance. Utilize the instanceof
operator to check types before casting.
Example Code for Class Casting:
Object obj = getObjectFromDataSource(); // Assume this returns an Object
if (obj instanceof String) {
String str = (String) obj;
// Process string data
} else {
System.err.println("Expected a String, but got " + obj.getClass().getName());
}
3. Handling JSON Responses
When working with JSON data from APIs, it is imperative to parse the data correctly. Use libraries such as Jackson or Gson to deserialise JSON into Java objects in a safe manner.
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonImporter {
private ObjectMapper objectMapper = new ObjectMapper();
public MyObject importJson(String jsonString) throws IOException {
return objectMapper.readValue(jsonString, MyObject.class);
}
}
4. Fixing Database Schema Mismatches
If your application interacts with a database, ensure that your entity classes map correctly to your database schema. Consider using Java's JPA for better type handling with an ORM (Object-Relational Mapping) approach.
@Entity
public class User {
@Id
private Long id;
@Column
private String name;
@Column
private Integer age; // Ensure this matches the database schema
}
The Last Word
Dealing with type errors during Java data imports can be challenging, but with the right strategies and best practices, you can mitigate risks and ensure smooth, reliable data handling. Remember, the key is to implement robust validation, leverage type-safe libraries, and consistently manage data formats.
By following the insights presented in this blog, you can enhance the stability and integrity of your Java applications. Should you encounter specific issues or want to explore related topics further, check out the article titled "Taming JavaScript Blobs: Fixing Bulk Import Errors" for additional strategies and tips.
Feel free to share your thoughts or questions in the comments below! Happy coding!
Checkout our other articles