Understanding SOLID Principles: Avoid Common Java Pitfalls
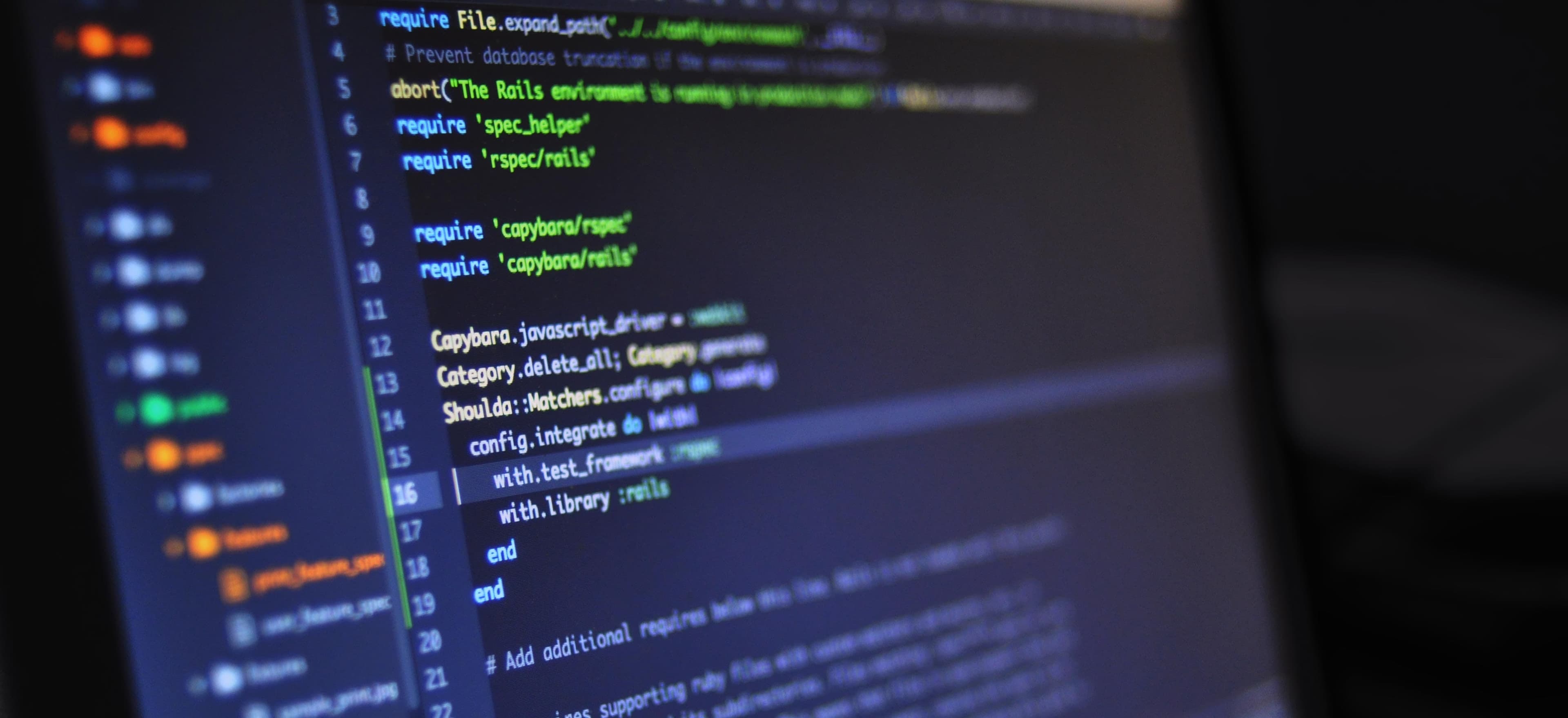
- Published on
Understanding SOLID Principles: Avoid Common Java Pitfalls
Java is a powerful and versatile programming language, widely adopted for its ability to build robust and scalable applications. However, as projects grow in complexity, adhering to best practices becomes paramount. Among these best practices, the SOLID principles stand out as essential guidelines for creating maintainable and flexible code. In this article, we will explore these principles in detail and demonstrate how they can help you avoid common pitfalls in Java programming.
What Are the SOLID Principles?
The SOLID principles are a set of five design principles intended to facilitate software development and ensure safety and clarity in programming. Introduced by Robert C. Martin, these principles help developers create code that is easy to manage and understand. The acronym "SOLID" represents the following principles:
- S - Single Responsibility Principle (SRP)
- O - Open/Closed Principle (OCP)
- L - Liskov Substitution Principle (LSP)
- I - Interface Segregation Principle (ISP)
- D - Dependency Inversion Principle (DIP)
By understanding and applying these principles, Java developers can prevent potential issues that arise from overly complex and tightly-coupled code.
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. This means each class in your application should have a specific responsibility, making the codebase easier to understand, maintain, and modify.
Example
public class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Other user-related methods
}
In the code above, the User
class is responsible for holding user details. If we were to add methods for user authentication or data validation, we would violate the SRP. Instead, we can create separate classes for these responsibilities:
public class UserAuth {
public boolean authenticate(User user, String password) {
// Authentication logic
return true; // Mock implementation
}
}
public class UserValidator {
public boolean validateEmail(User user) {
// Email validation logic
return user.getEmail().contains("@");
}
}
By structuring our code this way, you avoid future complications linked to modifying user responsibilities, as discussed in the article Why Ignoring the SOLID Principles Could Break Your Code.
2. Open/Closed Principle (OCP)
The Open/Closed Principle dictates that classes should be open for extension but closed for modification. This implies that your system should allow behavior to be extended without altering existing code, promoting easier maintenance and reducing the risk of introducing bugs.
Example
Suppose you have a method that processes payments:
public class PaymentProcessor {
public void processPayment(PaymentType paymentType) {
switch (paymentType) {
case CREDIT_CARD:
// Handle credit card processing
break;
case PAYPAL:
// Handle PayPal processing
break;
}
}
}
Adding a new payment method requires modifying this already existing code. To follow the OCP, we can use the Strategy Pattern:
interface PaymentStrategy {
void pay();
}
class CreditCardPayment implements PaymentStrategy {
@Override
public void pay() {
// Credit card payment logic
}
}
class PayPalPayment implements PaymentStrategy {
@Override
public void pay() {
// PayPal payment logic
}
}
Now the PaymentProcessor
can remain unchanged while new payment methods can be seamlessly added:
public class PaymentProcessor {
public void processPayment(PaymentStrategy paymentStrategy) {
paymentStrategy.pay();
}
}
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle asserts that objects of a superclass should be replaceable with objects of a subclass without altering the desirable properties of the program. This principle emphasizes code reliability and readability as it encourages the use of inheritance appropriately.
Example
Consider a class hierarchy of shapes:
class Rectangle {
protected int width;
protected int height;
public void setWidth(int width) {
this.width = width;
}
public void setHeight(int height) {
this.height = height;
}
public int area() {
return width * height;
}
}
class Square extends Rectangle {
@Override
public void setWidth(int width) {
this.width = width;
this.height = width; // Ensures the square remains a square
}
@Override
public void setHeight(int height) {
this.width = height; // Also ensures the square remains a square
this.height = height;
}
}
In this case, substituting a Square
object where a Rectangle
is expected might break the expectation of rectangular behavior since the area calculation relies on independent height and width. To adhere to LSP, squares must not interfere with rectangle's behavior. A better design would separate these two concepts altogether.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle states that no client should be forced to depend on methods it does not use. In simpler terms, larger interfaces should be split into smaller, more specific ones, thus promoting readability and minimizing the impact of changes.
Example
Consider an interface for various devices:
interface Device {
void print();
void fax();
void scan();
}
Any class implementing this interface must implement all methods, even if it doesn't support faxing or scanning. Instead, we can create more focused interfaces:
interface Printer {
void print();
}
interface FaxMachine {
void fax();
}
interface Scanner {
void scan();
}
With this setup, you can implement only the interfaces your class requires, leading to a more maintainable design.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules; both should depend on abstractions. This promotes flexibility in the system architecture.
Example
Instead of a high-level class directly depending on a low-level class, use an abstraction:
class LightBulb {
public void turnOn() {
// Light bulb logic
}
}
class Switch {
private LightBulb bulb;
public Switch(LightBulb bulb) {
this.bulb = bulb;
}
public void operate() {
bulb.turnOn();
}
}
Ideally, the Switch
class should not directly depend on LightBulb
. Instead, we should use an interface:
interface Switchable {
void turnOn();
}
class LightBulb implements Switchable {
public void turnOn() {
// Light logic
}
}
class Switch {
private Switchable device;
public Switch(Switchable device) {
this.device = device;
}
public void operate() {
device.turnOn();
}
}
By using interfaces, we can modify or swap implementations without altering the Switch
class, ensuring a more flexible architecture.
The Bottom Line
Understanding and applying the SOLID principles is key to avoiding common pitfalls in Java development. By adhering to these principles, you create a more maintainable, extensible, and reliable codebase.
As you continue your journey in Java programming, remember the importance of these foundational principles. If you'd like to dive deeper into the ramifications of ignoring such guiding principles, be sure to check out the article Why Ignoring the SOLID Principles Could Break Your Code.
Incorporating the SOLID principles into your coding practices can elevate your Java skills significantly. The journey of becoming a proficient developer is continuous, but with a solid understanding of these design principles, you're ensuring longevity and quality in your code. Happy coding!
Checkout our other articles