Mastering SOLID Principles to Enhance Your Java Applications
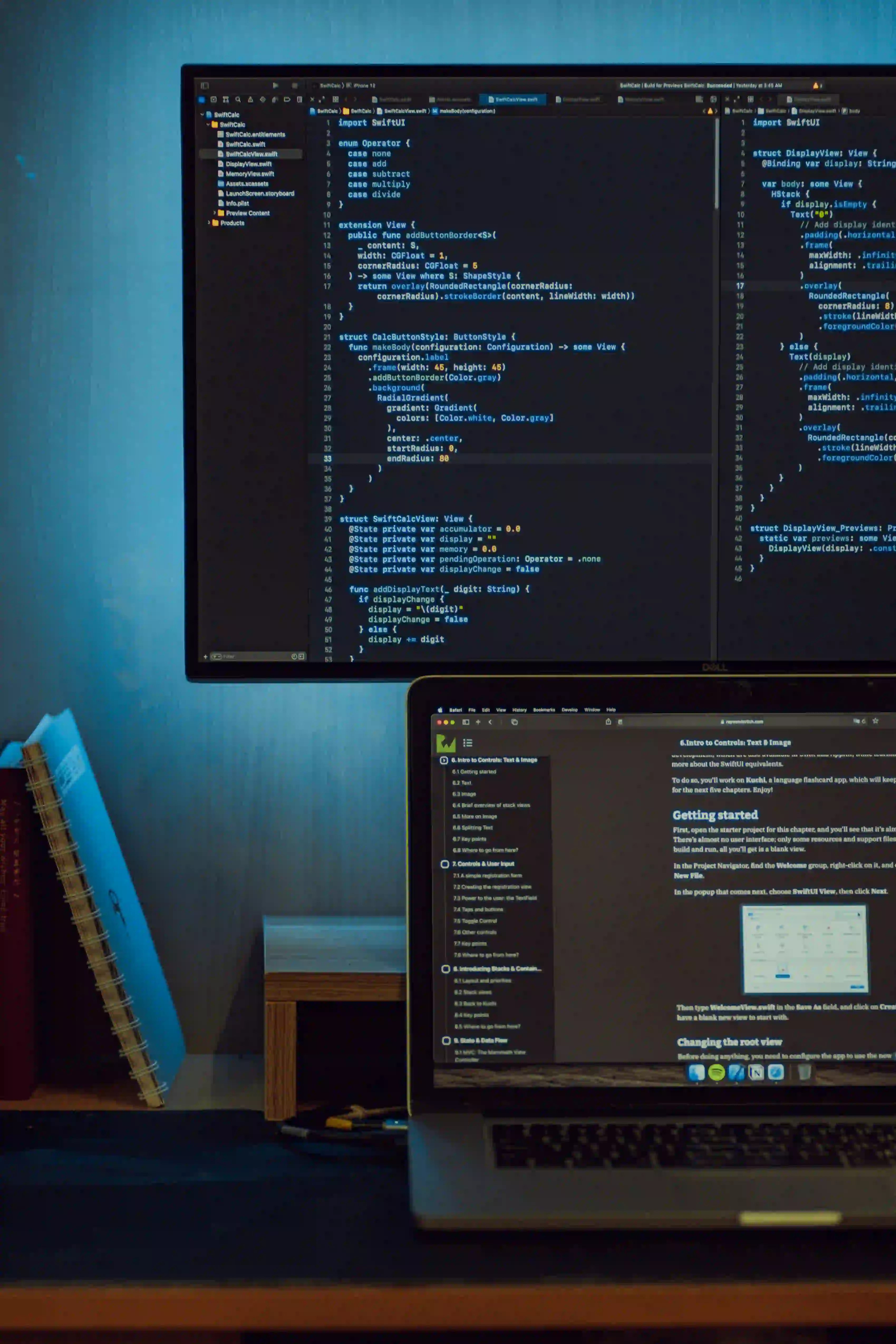
Mastering SOLID Principles to Enhance Your Java Applications
In the world of software engineering, keeping your code clean and maintainable is critical for long-term success. This is where the SOLID principles come into play. The SOLID acronym stands for five design principles intended to improve the quality of software design. In this blog post, we will explore each of the SOLID principles in the context of Java, demonstrating how they can help elevate your applications from mere code to well-architected masterpieces.
Table of Contents
- What Are SOLID Principles?
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
- Conclusion: The Importance of SOLID Principles
What Are SOLID Principles?
The SOLID principles, introduced by Robert C. Martin, serve as guidelines for creating more maintainable and understandable software. They emphasize the importance of good design and help developers avoid common pitfalls that can lead to unmanageable codebases. In a previous article titled "Why Ignoring the SOLID Principles Could Break Your Code," we discussed the ramifications of overlooking these principles. Here, we elaborate on each principle to enrich your understanding and application in Java.
Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. In simpler terms, it means that each class should only focus on one responsibility.
Why is SRP Important?
Adhering to SRP enhances code readability and maintainability. When a class does only one job, it becomes simpler and easier to test. Moreover, changes made to one part of the application won't inadvertently affect unrelated areas.
Code Example
Consider a User
class:
public class User {
private String name;
private String email;
public void sendEmail(String message) {
// Code to send email
}
public void saveUser() {
// Code to save user in database
}
}
This class violates the SRP. It has two responsibilities: sending emails and saving the user data.
Refactored Code
public class User {
private String name;
private String email;
// Getter and Setter methods
}
public class UserService {
public void saveUser(User user) {
// Code to save user in database
}
}
public class EmailService {
public void sendEmail(User user, String message) {
// Code to send email
}
}
In the refactored code, we have separated the responsibilities. User
maintains user data, UserService
handles persistence, and EmailService
takes care of email functionality. This makes the codebase more organized and easier to maintain.
Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
Why is OCP Important?
This principle allows developers to add new functionality without altering existing code, promoting greater stability and reducing the risk of introducing bugs.
Code Example
Let's consider a simple implementation of a payment system:
public class PaymentProcessor {
public void processPayment(String paymentType) {
if (paymentType.equals("credit")) {
// Process credit card payment
} else if (paymentType.equals("paypal")) {
// Process PayPal payment
}
}
}
This code violates OCP. To add a new payment method, we must modify the existing class.
Refactored Code
interface Payment {
void pay();
}
class CreditCardPayment implements Payment {
public void pay() {
// Process credit card payment
}
}
class PayPalPayment implements Payment {
public void pay() {
// Process PayPal payment
}
}
class PaymentProcessor {
public void processPayment(Payment payment) {
payment.pay();
}
}
In this version, the PaymentProcessor
is closed for modification. To introduce a new payment method, simply implement the Payment
interface. This not only adheres to OCP but also increases the flexibility of your application.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle asserts that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. Essentially, subclasses should extend the behavior of their superclass without altering the expected functionality.
Why is LSP Important?
LSP ensures interoperability between classes. If a subclass does not honor the contract established by its parent class, it breaks the consumer's expectations.
Code Example
class Bird {
public void fly() {
// Code to fly
}
}
class Ostrich extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Ostriches can't fly");
}
}
This code violates LSP, as substituting Bird
with Ostrich
leads to runtime exceptions.
Refactored Code
interface Bird {
void move();
}
class FlyingBird implements Bird {
public void move() {
fly();
}
public void fly() {
// Code to fly
}
}
class Ostrich implements Bird {
public void move() {
run();
}
public void run() {
// Code to run
}
}
Now, both FlyingBird
and Ostrich
adhere to the LSP. Each class implements the Bird
interface, providing appropriate behavior for their contexts.
Interface Segregation Principle (ISP)
The Interface Segregation Principle posits that no client should be forced to depend on methods it does not use.
Why is ISP Important?
By adhering to ISP, you can minimize the impact of changes. Smaller, more focused interfaces encourage better organization and promote ease of testing.
Code Example
Imagine a notification interface:
interface Notification {
void sendEmail();
void sendSMS();
}
class UserNotification implements Notification {
public void sendEmail() {
// Send email
}
public void sendSMS() {
// Send SMS
}
}
This approach forces the UserNotification
class to implement both methods, regardless of whether both are needed.
Refactored Code
interface EmailNotification {
void sendEmail();
}
interface SMSNotification {
void sendSMS();
}
class UserEmailNotification implements EmailNotification {
public void sendEmail() {
// Send email
}
}
class UserSMSNotification implements SMSNotification {
public void sendSMS() {
// Send SMS
}
}
In this refactored code, we have separate interfaces for email and SMS notifications. This promotes higher cohesion and makes your code easier to maintain and extend.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle indicates that high-level modules should not depend on low-level modules. Both should depend on abstractions. Additionally, abstractions should not depend on details; details should depend on abstractions.
Why is DIP Important?
DIP promotes loose coupling, which makes code easier to refactor. It also enhances testability, as dependencies can be replaced with mocks or stubs during testing.
Code Example
class NotificationService {
private EmailService emailService;
public NotificationService() {
this.emailService = new EmailService();
}
public void notify(String message) {
emailService.sendEmail(message);
}
}
Here, NotificationService
directly depends on the implementation of EmailService
, making it less flexible.
Refactored Code
interface MessageService {
void sendMessage(String message);
}
class EmailService implements MessageService {
public void sendMessage(String message) {
// Code to send email
}
}
class NotificationService {
private MessageService messageService;
public NotificationService(MessageService messageService) {
this.messageService = messageService;
}
public void notify(String message) {
messageService.sendMessage(message);
}
}
Now, NotificationService
depends on the abstraction (MessageService
). You can easily implement new message services, like SMS or push notifications, without modifying the NotificationService
class.
Bringing It All Together: The Importance of SOLID Principles
Mastering the SOLID principles can significantly improve the quality of your Java applications. They create a framework for writing clean, maintainable, and scalable code. Understanding and applying these principles helps prevent code rot and enhances team collaboration.
Incorporating these principles into your development process is not merely a good practice; it's essential for sustainable growth and development. If you want to explore more about the implications of ignoring these principles, I highly recommend checking out the article "Why Ignoring the SOLID Principles Could Break Your Code."
By embracing the SOLID principles, you not only invest in your current project but also pave the way for future success. Happy coding!