Boost Your Java Application's Health with Proper Exception Handling
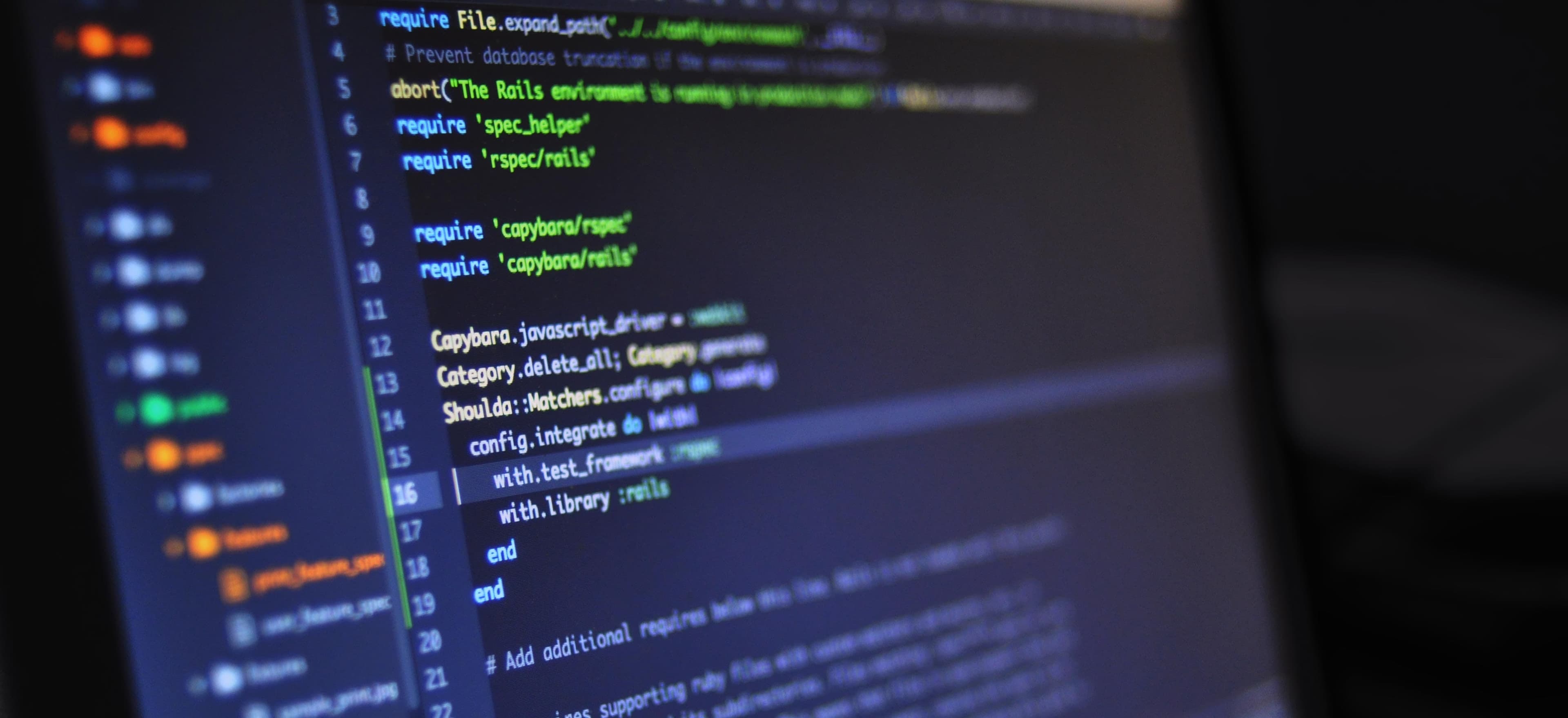
- Published on
Boost Your Java Application's Health with Proper Exception Handling
Java is a robust programming language, renowned for its efficiency and versatility. However, just like any other formidable tool, leveraging its full potential requires deep understanding and best practices—particularly in the realm of exception handling. Poor exception handling can lead to application crashes, unpredictable behavior, and ultimately a deteriorated user experience. In contrast, effective exception management enhances an application’s resilience, enabling it to handle unforeseen circumstances gracefully.
In this blog post, we’ll delve into why exception handling is critical, how you can implement it effectively in Java, and some best practices to ensure your application remains healthy and responsive.
Why Exception Handling Matters
Imagine you are sipping on a cup of chamomile tea while reading through an interesting article titled Kamillentee: Ein Wundermittel für Gesundheit & Wohlbefinden. In this article, the benefits of chamomile tea for well-being are thoroughly discussed. Now imagine this reading experience suddenly interrupted because of an application error. It would certainly break your immersion!
Java's exception handling mechanism is comparable to the soothing properties of chamomile tea; it helps calm the storm of runtime errors in your applications, ensuring a seamless user experience. It prevents application crashes and allows for graceful degradation, providing users with meaningful feedback if something goes awry.
Key Concepts of Exception Handling
-
Exception Types: Understanding the various types of exceptions is crucial.
- Checked Exceptions: These must be either handled in a try-catch block or declared in a method's
throws
clause. - Unchecked Exceptions: These include runtime exceptions that the compiler does not mandate to be handled explicitly.
- Checked Exceptions: These must be either handled in a try-catch block or declared in a method's
-
Try-Catch Blocks: The core constructs for catching exceptions in Java. A try block contains the code that may throw an exception, while the catch block contains the handling logic.
-
Finally Block: Executes regardless of whether an exception is thrown or not. Ideal for cleanup tasks, e.g., closing file streams or database connections.
-
Custom Exceptions: Occasionally, you may need to create your own exceptions to represent specific error conditions in your application.
Implementing Exception Handling in Your Java Application
Now that we understand the importance of effective exception management, let’s implement it within a simple Java application example that performs division operations:
Example Code Snippet: Safe Division
public class SafeDivision {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result is: " + result);
} catch (ArithmeticException e) {
System.err.println("Error: " + e.getMessage());
} finally {
System.out.println("Execution complete.");
}
}
public static int divide(int numerator, int denominator) {
return numerator / denominator; // May throw ArithmeticException
}
}
Commentary on the Code
Why Use Try-Catch: The try
block safely contains the code that may throw an exception. In our case, the division operation could lead to an ArithmeticException
when the denominator is zero.
Error Handling: In the catch block, we handle the ArithmeticException
gracefully by logging a clear error message. This prevents a runtime crash and offers valuable feedback for debugging.
Finalization: The finally
block ensures that cleanup code runs after the exception handling, no matter what. This is useful for freeing up resources and reinforces application stability.
Custom Exceptions
In some cases, you might want to implement custom errors. This enhances error clarity and helps in better debugging.
class DivisionByZeroException extends Exception {
public DivisionByZeroException(String message) {
super(message);
}
}
public class AdvancedDivision {
public static void main(String[] args) {
try {
int result = advancedDivide(10, 0);
System.out.println("Result is: " + result);
} catch (DivisionByZeroException e) {
System.err.println("Custom Error: " + e.getMessage());
} finally {
System.out.println("Execution completed.");
}
}
public static int advancedDivide(int numerator, int denominator) throws DivisionByZeroException {
if (denominator == 0) {
throw new DivisionByZeroException("Denominator cannot be zero!");
}
return numerator / denominator;
}
}
Why Implement Custom Exceptions?
- Clarity: Custom exceptions can specify the nature of the problem, making debugging easier.
- Separation: They allow for finer control over different exception types and error propagation.
- Maintenance: Easier to maintain and modify error-handling logic corresponding to specific exceptions.
Best Practices for Exception Handling
1. Catch Specific Exceptions
Avoid catching generic exceptions (e.g., Exception
or Throwable
). Instead, catch specific exceptions that you can handle. This is critical because it helps avoid hiding bugs.
try {
// risky code
} catch (IOException e) {
// handle IOException
} catch (SQLException e) {
// handle SQLException
}
2. Use Finally for Cleanup
Always use finally
blocks for closing resources like database connections or files. It helps prevent resource leaks, enhancing overall application health.
3. Log Exceptions
Never ignore exceptions. Log them appropriately so you can later analyze the cause and frequency.
try {
// risky code
} catch (Exception e) {
logger.error("An error occurred: ", e);
}
4. Don’t Use Exceptions for Control Flow
Exceptions should not be used to control normal application flow. They are meant for exceptional circumstances. Using exceptions for flow control can significantly degrade application performance.
5. Document Exceptions
Document the exceptions your methods may throw using Javadoc. This is particularly helpful for developers who will work with your code, allowing them to anticipate and handle exceptions properly.
Closing the Chapter
Effective exception handling in Java is not merely about wrapping code within try-catch blocks. It's a comprehensive strategy to enhance the reliability and resilience of your application. By understanding the types of exceptions, employing custom error classes, and following best practices, you can elevate your Java applications to new heights, much like how chamomile tea supports your health and well-being.
As you dive deeper into Java programming, remember that robust exception management ensures your applications remain responsive, efficient, and user-friendly. Happy coding!
For further readings and insights on health, consider checking out the article on chamomile tea and its notable benefits at Kamillentee: Ein Wundermittel für Gesundheit & Wohlbefinden.