Mastering Java Caching Strategies for Efficient Data Fetching
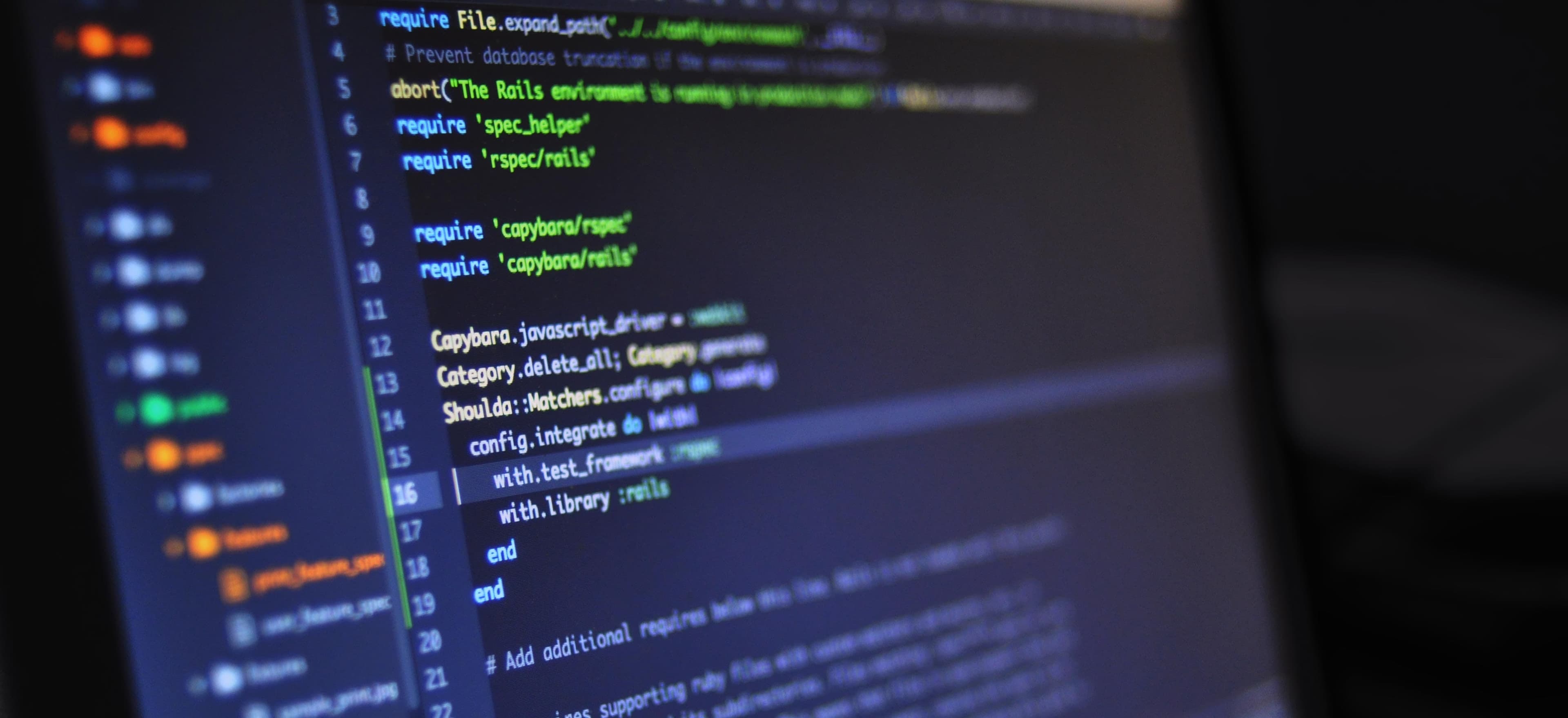
- Published on
Mastering Java Caching Strategies for Efficient Data Fetching
In the world of software development, making efficient applications is a constant challenge. One key strategy in achieving efficiency is data caching. Caching can significantly enhance the performance of Java applications by reducing the load on data sources and minimizing latency. In this blog post, we will explore various caching strategies in Java, why they are essential, and how to implement them effectively.
What is Caching?
Caching refers to the technique of storing copies of files or data in a temporary storage location, so future requests for that data can be served faster. When an application fetches data, it often goes through a complex process involving network calls and database queries, both of which can be slow. Caching helps circumvent some of these slower operations by keeping frequently accessed data in memory.
Why Use Caching?
- Performance Improvement: By reducing the time taken to retrieve data, your applications can operate more efficiently.
- Load Reduction: Caching can reduce the number of calls made to a database, which decreases load and resource consumption.
- User Experience: Faster applications lead to a better experience for users, enhancing satisfaction and engagement.
Now, let’s dive deeper into different caching strategies available in Java.
Memory Caching with ConcurrentHashMap
The simplest form of caching is done in-memory, and ConcurrentHashMap
is a great tool for this purpose. It offers thread-safe operations, making it suitable for multi-threaded applications.
Basic Example of Memory Caching
import java.util.concurrent.ConcurrentHashMap;
public class MemoryCache {
private final ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
public void put(String key, String value) {
cache.put(key, value);
}
public String get(String key) {
return cache.get(key);
}
public boolean containsKey(String key) {
return cache.containsKey(key);
}
}
Why Use ConcurrentHashMap?
- Thread-Safety: It allows safe concurrent reads and updates without explicit synchronization.
- Performance: It is optimized for concurrent access, making it more efficient under contention compared to other hash map implementations.
Using Caffeine for Advanced Caching
For more advanced caching capabilities, libraries like Caffeine offer a high-performance caching library for Java.
Caffeine Caching Example
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class CaffeineCache {
private final Cache<String, String> cache;
public CaffeineCache() {
cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(1000)
.build();
}
public void put(String key, String value) {
cache.put(key, value);
}
public String get(String key) {
return cache.getIfPresent(key);
}
}
Why Use Caffeine?
- Eviction Policies: It offers various eviction policies based on size, time, or access patterns.
- High Efficiency: Caffeine is known for better performance than other existing cache libraries, such as Guava, especially for high-throughput scenarios.
Distributed Caching with Redis
As applications scale, data caching often becomes distributed. Redis, an in-memory data structure store, is a popular choice. Integrating Redis into your Java application is straightforward, thanks to libraries like Jedis.
Redis Caching Example
import redis.clients.jedis.Jedis;
public class RedisCache {
private final Jedis jedis;
public RedisCache(String host, int port) {
this.jedis = new Jedis(host, port);
}
public void put(String key, String value) {
jedis.set(key, value);
}
public String get(String key) {
return jedis.get(key);
}
}
Why Use Redis?
- Scalability: Redis can handle a large number of connections simultaneously, making it suitable for microservices architecture.
- Complex Data Structures: Redis supports various data types beyond simple key-value pairs, like lists, sets, and hashes.
Implementing Cache Invalidation
Cache invalidation is a critical component of effective caching. It ensures that stale data does not remain in the cache after updates. This can be achieved utilizing a time-to-live (TTL) strategy to automatically remove data or manual invalidation techniques upon data updates.
Example of Cache Invalidation
Here’s a simple example that demonstrates programmatic cache invalidation:
import java.util.concurrent.ConcurrentHashMap;
public class CacheWithInvalidation {
private final ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
private final ConcurrentHashMap<String, Long> timestamps = new ConcurrentHashMap<>();
private final long ttl = 60000; // 1 minute
public void put(String key, String value) {
cache.put(key, value);
timestamps.put(key, System.currentTimeMillis());
}
public String get(String key) {
if (isExpired(key)) {
cache.remove(key);
timestamps.remove(key);
return null;
}
return cache.get(key);
}
private boolean isExpired(String key) {
Long timestamp = timestamps.get(key);
if (timestamp == null) return true;
return System.currentTimeMillis() - timestamp > ttl;
}
}
Why is Cache Invalidation Important?
- Data Freshness: Ensures that users always get the most recent data.
- Resource Optimization: Helps avoid unnecessary load on the primary data source.
The Last Word
Mastering caching strategies in Java is an essential skill for developers aiming to build efficient and responsive applications. Whether you choose in-memory caching with ConcurrentHashMap
, employ advanced libraries like Caffeine, or opt for distributed caching using Redis, each has its merits.
Additionally, implementing cache invalidation strategies is crucial for maintaining data integrity throughout the application's lifecycle. For frontend developers looking to improve their own caching techniques, consider checking out the article titled "Struggling with Cache Management in React Query?" for insights tailored to React applications.
Final Thoughts
Caching is a powerful method to improve the performance of your Java applications. By analyzing your needs and selecting the appropriate caching strategy, you could see significant improvements in efficiency. So, whether it's improving load times or enhancing user experience, remember that effective caching is often at the heart of a well-functioning application. Start implementing caching tactics in your projects today and experience the benefits first-hand!
Checkout our other articles