Mastering Java: Tackling Object Equivalence Issues
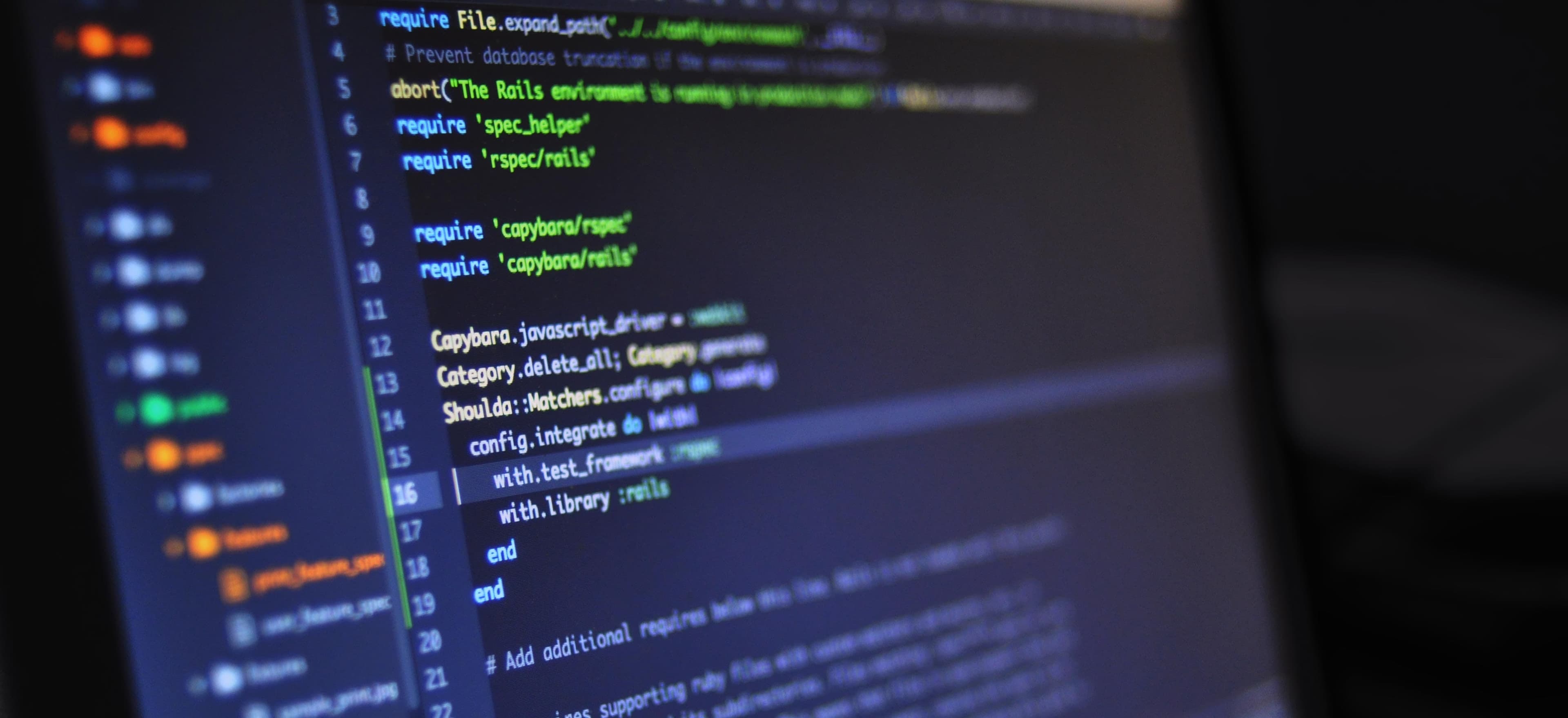
- Published on
Mastering Java: Tackling Object Equivalence Issues
In the world of Java programming, one of the most critical aspects to master is object equivalence. Understanding how to properly assess whether two objects are equivalent can save you from numerous bugs and performance issues. This blog post will explore the nuances of object equivalence, focusing on the equals()
method, the hashCode()
method, and the importance of implementing these methods effectively.
What Is Object Equivalence?
Object equivalence in Java refers to the way we determine if two instances of a class are considered “equal." By default, the equals()
method in the Object
class compares object references. In most cases, this isn't sufficient for class instances, especially when they carry meaningful data.
Why Is Object Equivalence Important?
When working on collections or data structures, understanding how Java evaluates equality can affect performance and accuracy. For instance, using the wrong equivalence logic can lead to:
- Incorrect collection operations: Such as duplicate entries or failed searches.
- Logic errors: Where a program behaves unexpectedly.
- Inconsistent data: Which can lead to hard-to-trace bugs.
Implementing equals()
The Basics
The equals()
method needs to be overridden to define what equality means for your objects. Typically, this involves comparing the fields of the objects being compared. Here is a basic example:
@Override
public boolean equals(Object obj) {
if (this == obj) return true; // Check for reference equality
if (obj == null || getClass() != obj.getClass()) return false; // Null and type check
MyClass other = (MyClass) obj; // Cast and compare fields
return this.field1.equals(other.field1) && this.field2.equals(other.field2);
}
Why This Implementation?
- Reference Check First: It's efficient to check if the two references point to the same object.
- Type Safety: By checking if
obj
is null or of the same class type, we avoid potentialClassCastException
. - Field Comparison: Here, you're defining what it means for two instances to be equivalent. It’s critical to ensure you're comparing the right fields.
An Example Class
To solidify your understanding, let’s consider a more thorough example with a Person
class.
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && (name != null ? name.equals(person.name) : person.name == null);
}
}
In this example, we’re defining equivalence based on both the name
and age
attributes. Note how we handle potential null
values safely.
Implementing hashCode()
After overriding equals()
, it is essential to override hashCode()
. This is crucial when objects are used in hash-based collections such as HashMap
and HashSet
.
The Contract between equals()
and hashCode()
- If two objects are equal according to
equals()
, they must have the samehashCode
. - If two objects are not equal according to
equals()
, they may have the samehashCode
(as collisions can occur).
Example of hashCode()
Here’s how you might implement hashCode()
for our Person
class:
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + age; // 31 is a prime number
return result;
}
Why This Matters
Using name.hashCode()
ensures that the hash code is based on the fields defining equality, while multiplying the interim result by a prime (31) helps in distributing hash codes more evenly, which minimizes collisions.
Complete Example: The Person
Class
Here is the complete Person
class with both equals()
and hashCode()
properly implemented:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && (name != null ? name.equals(person.name) : person.name == null);
}
@Override
public int hashCode() {
int result = name != null ? name.hashCode() : 0;
result = 31 * result + age;
return result;
}
}
Tips for Implementing Object Equivalence
- Consistency: Ensure that the
equals()
andhashCode()
implementations are consistent in their logic. - Use
@Override
Annotation: Always use the@Override
annotation when overriding these methods. It improves readability and prevents mistakes. - Don't Use
==
to Compare Objects: Using==
checks if both references point to the same object and won't yield the correct result for object equivalence checks. - Consider Immutable Fields: If your class contains mutable fields, consider whether object equivalence should include these. Immutable classes can greatly simplify equality logic.
Advanced Topics
Composition Over Inheritance
When designing classes, consider composition over inheritance. This approach allows you to create more flexible and interchangeable pieces of code, simplifying the equality logic since you can directly leverage composed object equality rather than dealing with inherited structures.
Custom Comparators
In some cases, you may want to implement comparison logic that is not strictly equivalent. Creating a custom comparator using the Comparator
interface allows for more nuanced equality checks. This approach is useful when sorting collections.
public class PersonComparator implements Comparator<Person> {
@Override
public int compare(Person p1, Person p2) {
int nameComparison = p1.getName().compareTo(p2.getName());
return nameComparison != 0 ? nameComparison : Integer.compare(p1.getAge(), p2.getAge());
}
}
Using Java 7 and Beyond
With Java 7 and higher, consider using Objects.equals()
and Objects.hash()
utility methods. These can greatly simplify your code.
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && Objects.equals(name, person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
Final Thoughts
Understanding object equivalence is a vital skill for any Java developer. It’s the foundation upon which many critical components of Java are built, particularly those related to collections. By properly overriding the equals()
and hashCode()
methods, you'll create classes that behave as expected in a variety of contexts. By utilizing these principles, you can avoid bugs, enhance performance, and ultimately create clearer and more efficient Java applications.
Further Reading
By mastering these concepts, you set the stage for advanced Java programming and a deeper understanding of the language's capabilities. Happy coding!
Checkout our other articles