5 Common Challenges in Using Drools and jBPM on KIE Apps
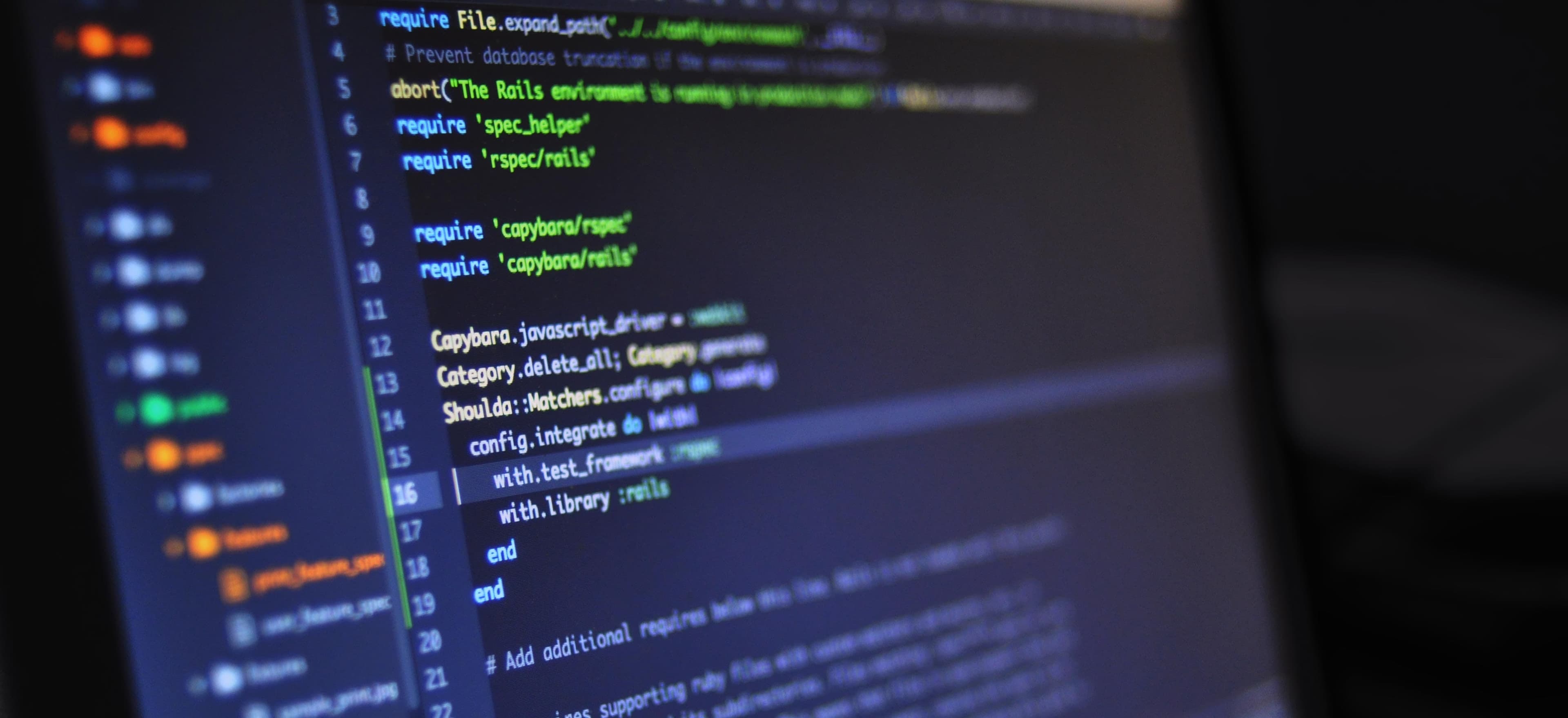
- Published on
5 Common Challenges in Using Drools and jBPM on KIE Apps
Drools and jBPM are powerful tools in the business process management (BPM) and business rules management system (BRMS) landscape. Both are part of the KIE (Knowledge Is Everything) ecosystem, enabling developers to create intelligent, dynamic applications. Although they provide substantial value, developers often face challenges when integrating Drools and jBPM into KIE applications.
In this blog post, we'll delve into the five common challenges you might encounter while using Drools and jBPM on KIE apps, along with solutions and code snippets to improve your development experience.
1. Complex Rule Management
Challenge
Drools allows the definition of rules in a natural language format, making it easier to understand for non-technical stakeholders. However, as the number of rules grows, managing them can become cumbersome.
Solution
Adopting a modular approach to rule management can help. You can organize rules into packages or use a directory structure. This enhances maintainability and readability. You can also make use of KIE Workbench, which provides a web-based interface for managing rules.
Code Snippet
Here is a simple example of creating a rule in Drools:
package com.example.rules;
import com.example.model.Customer;
rule "Customer Discount Rule"
when
$customer: Customer(age < 18)
then
$customer.setDiscount(10);
System.out.println("10% discount applied for underage customer.");
end
Why this approach?
This encapsulates the rule within a specific package, making it easier to find and manage. Additionally, using the when-then
format maintains clarity.
2. Performance Issues
Challenge
Drools relies heavily on its inference engine, which can lead to performance bottlenecks, especially as the rule base grows complex. The Rule-based inference engines can become the slowest part of your application if not optimized correctly.
Solution
Utilizing indexed fields can significantly speed up rule evaluation. Leveraging salience for prioritizing rules can also improve performance. Plus, avoid using "complex" conditions unnecessarily.
Example of Indexed Fields
declare Customer
@key
String id;
int age;
end
Why this improvement?
Defining the Customer
class allows the rule engine to utilize indexes for quicker lookups and evaluations, reducing the processing time during rule execution.
3. jBPM Workflow Complexity
Challenge
Implementing complex workflows can be a daunting task with jBPM. Managing various states, timers, and human tasks can quickly turn a simple workflow into a convoluted mess.
Solution
Using sub-processes can simplify complex workflows by compartmentalizing tasks. Event listeners and error handling also play a vital role in managing workflow complexity.
Code Snippet
Here’s an example of a jBPM process:
<process id="exampleProcess" name="Example Process" version="1.0">
<startEvent id="StartEvent_1" />
<sequenceFlow id="flow1" sourceRef="StartEvent_1" targetRef="Task_1" />
<userTask id="Task_1" name="Approve Request" >
<documentation>Task to approve a request</documentation>
</userTask>
<sequenceFlow id="flow2" sourceRef="Task_1" targetRef="EndEvent_1" />
<endEvent id="EndEvent_1" />
</process>
Why use sub-processes?
Incorporating sub-processes allows you to encapsulate complexity. It helps in modularizing your workflow, making it manageable and reusable across different processes.
4. Integration with Other Systems
Challenge
Integrating Drools and jBPM with existing applications can become challenging. Compatibility issues and differing data formats often arise.
Solution
Focusing on RESTful services for integration can help. Using JSON or XML data formats ensures better compatibility between systems.
Code Snippet
Here is an example of making a REST call from a jBPM process:
String response = restTemplate.postForObject("http://api.example.com/resource", requestBody, String.class);
Why REST APIs?
RESTful services are stateless and can easily interact with different systems, providing flexibility in integration. This makes it easier to develop, deploy, and maintain your KIE applications.
5. Debugging and Testing Challenges
Challenge
Debugging rules can be complex, as the logic encapsulated in these rules can lead to unexpected behaviors. jBPM workflows can present similar challenges, with tracking the flow of process states being non-trivial.
Solution
Utilizing the built-in logging mechanisms and rule trace features in Drools can aid in debugging. Furthermore, comprehensive testing strategies, including unit and integration tests, can catch issues early.
Example of Test Case for a Rule
Here’s an example of writing a unit test for our earlier defined discount rule:
import org.junit.Test;
import org.kie.api.runtime.KieSession;
import org.kie.api.KieServices;
public class DiscountRuleTest {
@Test
public void testCustomerDiscountRule() {
KieServices ks = KieServices.Factory.get();
KieSession kieSession = ks.getKieClasspathContainer().newKieSession("ksession-rules");
Customer customer = new Customer();
customer.setAge(17); // Underage customer
kieSession.insert(customer);
kieSession.fireAllRules();
assertEquals(10, customer.getDiscount());
}
}
Why unit testing is essential?
Unit tests help identify logical errors early in the development lifecycle. By isolating rules in tests, you can ensure they perform as expected without additional overhead.
The Bottom Line
Drools and jBPM are potent tools that can enhance your KIE applications when used effectively. By understanding and addressing these common challenges, you can leverage their capabilities to create intelligent applications that stand the test of time.
For further learning, consider exploring the official documentation or following community forums for best practices and updates in the Drools and jBPM community.
Navigating the complexities of business rules and processes can be daunting, but with a proactive approach and a clear understanding of the challenges, you can become adept at developing robust applications using Drools, jBPM, and the entire KIE ecosystem.
Checkout our other articles