The Dangers of Overconfidence in Unit Testing Validation
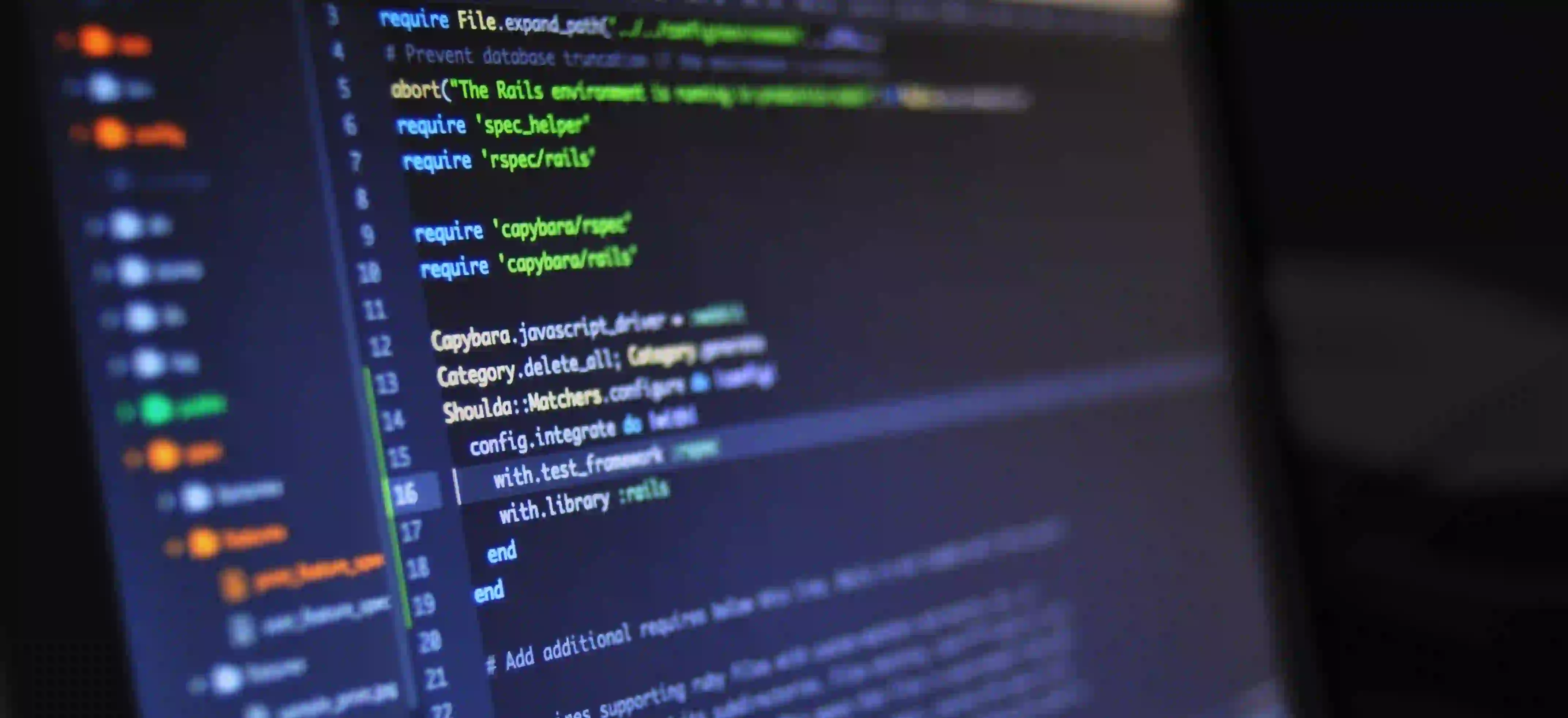
The Dangers of Overconfidence in Unit Testing Validation
In the ever-evolving landscape of software development, unit testing stands as a pillar of effective code validation. It serves as a safety net, ensuring that individual components of the application function correctly. However, there is a lurking danger that many developers overlook: overconfidence in unit testing validation. This article delves deep into the nuances of unit testing, the potential pitfalls of overconfidence, and strategies to mitigate these risks while ensuring robust application performance.
Understanding Unit Testing
Unit testing is a software testing methodology where individual components or functions of a program are tested in isolation. This practice helps developers identify issues at an early stage, enhancing maintainability and reliability. Tools like JUnit, Mockito, and TestNG are instrumental for Java developers in automating unit tests.
A Simple Example of Unit Testing in Java
Below is a simple example illustrating a unit test created using JUnit to validate a method that calculates the square of a number.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class MathUtils {
public static int square(int number) {
return number * number;
}
}
class MathUtilsTest {
@Test
public void testSquare() {
assertEquals(25, MathUtils.square(5));
assertEquals(0, MathUtils.square(0));
assertEquals(1, MathUtils.square(1));
assertEquals(100, MathUtils.square(10));
}
}
Why Unit Testing Matters
Unit tests help ensure that functions perform as expected, and they serve several purposes:
-
Catching Bugs Early: Launching a project without unit testing increases the risk of bugs. By testing smaller units, developers can identify errors quickly, saving time and resources.
-
Facilitating Refactoring: During development, code often requires modifications. Unit tests provide a benchmark to ascertain that changes do not break existing functionality.
-
Providing Documentation: Unit tests can serve as a form of documentation. They illustrate intended usage and expected outcomes, which can be beneficial for onboarding new team members.
The Temptation of Overconfidence
Despite the evident advantages, overconfidence in the unit testing process can lead to dire consequences. Developers may fall into the trap of believing their code is bulletproof simply because it passes all unit tests. This assumption can be dangerous for several reasons.
1. False Sense of Security
When developers relentlessly pursue test coverage without critically assessing the quality of the tests, they may assume the application is more reliable than it is. A complete suite of passing tests does not necessarily imply that the application behaves correctly under various scenarios.
2. Ignoring Integration Testing
Unit tests focus on smallest parts of an application in isolation. However, many bugs occur due to interactions between these parts. Ignoring integration and system testing can let errors slip through the cracks. Java developers should complement unit testing with integration tests using tools like Spring Test or Arquillian.
3. Assumptions in Test Logic
Overconfidence can lead developers to make unwarranted assumptions about their tests. For example, if a method reads data from an external source, a unit test that mocks this behavior may pass, but it will not account for real-world scenarios. An assertEquals method does not guarantee correctness if external dependencies are involved.
Strategies to Avoid Overconfidence
Emphasize Test Quality Over Quantity
While achieving high test coverage is a desirable goal, it should never come at the expense of test quality. Rather than simply writing more tests, developers should aim to write meaningful tests that capture a range of scenarios, including edge cases and failure cases.
Implement Continuous Integration (CI)
Using CI tools, such as Jenkins, Travis CI, or GitHub Actions, can automate testing processes and facilitate frequent testing of both unit and integration tests. This encourages developers to catch regressions and bugs proactively rather than relying solely on an initial test run.
Leverage Code Reviews
Regular code reviews can serve as an excellent mechanism to catch potential issues in testing strategies. Feedback from peers can provide valuable insights on how to improve both unit tests and the implications of the code.
Keep Abreast of Code Coverage Metrics
Tools like JaCoCo help monitor test coverage and identify untested paths in the application. However, developers should not blindly pursue higher metrics but instead assess whether tests meaningfully contribute to code integrity.
Utilize Contract Testing
As the complexity of applications increases, consider incorporating contract testing tools like Pact. This enables developers to establish contracts between services and ensure that each adheres to predefined interfaces. This helps bridge the gap between unit and integration testing effectively.
My Closing Thoughts on the Matter
In conclusion, while unit testing is a critical component of software development, overconfidence in its validation can lead to significant pitfalls. Understanding the limitations of unit tests and complementing them with comprehensive testing strategies will fortify the robustness of an application.
By being conscious of testing practices, leveraging modern tools, and fostering a culture of thoroughness and scrutiny, developers can minimize risks associated with overconfidence. Remember, quality over quantity reigns supreme in the realm of unit testing.
For further reading on unit testing best practices, consider exploring Martin Fowler's principles or JUnit documentation. They provide invaluable insights into fostering a culture of disciplined testing in software development.