Common Pitfalls When Using Functional Interfaces in Java 8
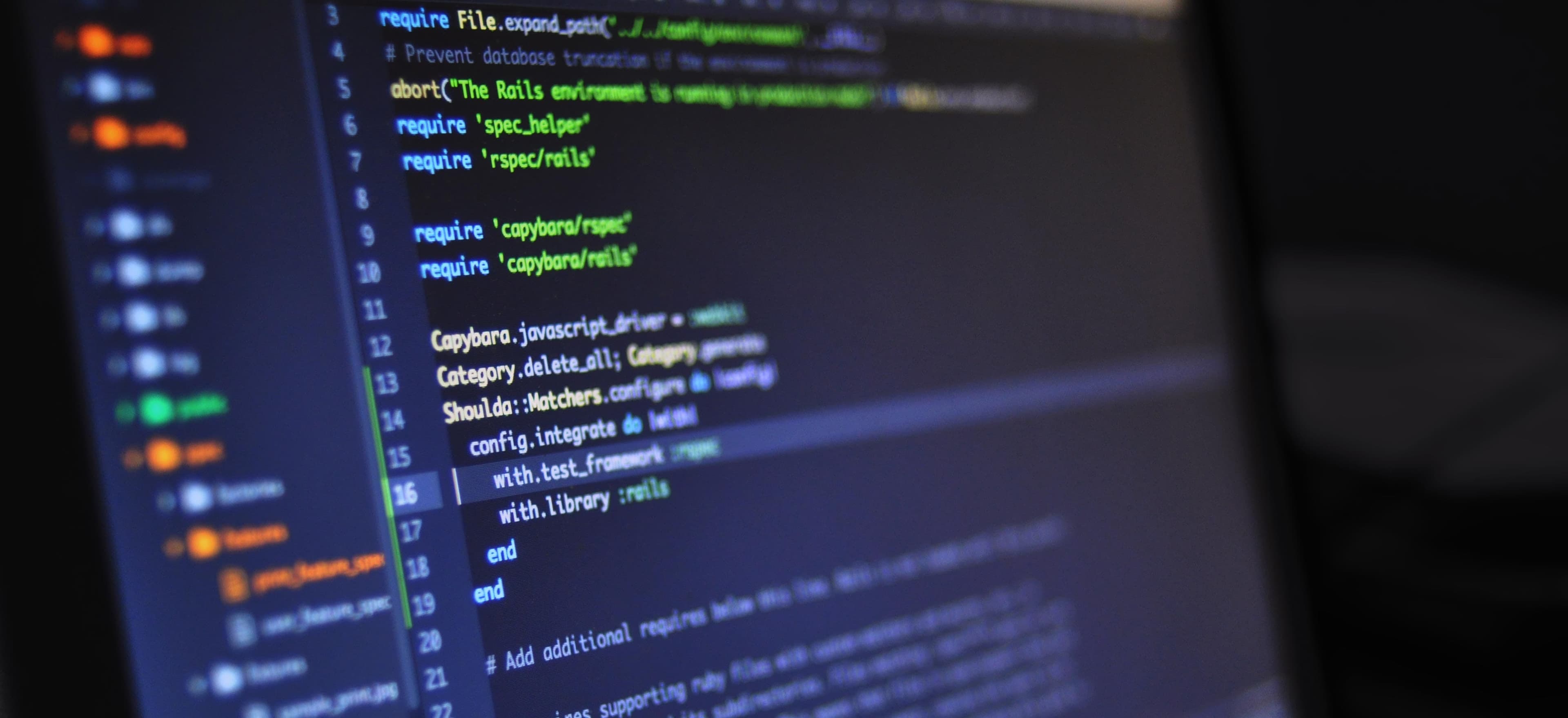
- Published on
Common Pitfalls When Using Functional Interfaces in Java 8
Java 8 introduced a new programming paradigm through its support for functional programming. One of the cornerstones of this paradigm is the functional interface. However, as with any powerful tool, there are common pitfalls developers can fall into when leveraging functional interfaces. In this post, we'll explore these pitfalls, their implications, and provide best practice tips to navigate them effectively.
What is a Functional Interface?
A functional interface is an interface that contains exactly one abstract method. They are foundational to Java 8's lambda expressions, Stream API, and method references. Functional interfaces help to define methods that can be passed as parameters, making code more concise and expressive.
Examples of built-in functional interfaces include Runnable
, Callable
, Consumer
, Supplier
, Function
, and Predicate
.
Example:
@FunctionalInterface
public interface MyFunctionalInterface {
void execute();
}
Here's a simple lambda implementing the MyFunctionalInterface
:
MyFunctionalInterface myFunc = () -> System.out.println("Hello, Functional Interface");
myFunc.execute();
In the above example, the lambda expression is used to instantiate the MyFunctionalInterface
. But while using functional interfaces, there are several common pitfalls you should be aware of.
1. Forgetting the Single Abstract Method Rule
A functional interface must have exactly one abstract method. If you accidentally add another abstract method, you will not be able to use lambda expressions or method references with that interface.
Example of the Pitfall:
@FunctionalInterface
public interface InvalidInterface {
void firstMethod();
void secondMethod(); // This will cause a compilation error
}
Why This Matters
Compiling errors could frustrate developers new to functional programming. Always check that your interface adheres to the Single Abstract Method (SAM) principle. Ensure that you don’t mistakenly introduce a second abstract method.
2. Not Using the @FunctionalInterface Annotation
While it’s not mandatory to annotate an interface as a functional interface, using the @FunctionalInterface
annotation is a best practice.
Example:
@FunctionalInterface
public interface MyFunctional {
void doSomething();
}
By marking your interface this way, the compiler can enforce the rules of functional interfaces. If you accidentally add another abstract method, it will alert you.
Why This Matters
Using this annotation can aid in code readability and help avoid potential issues during the development phase. It makes your intentions clear to other developers, fostering better code collaboration and maintenance.
3. Overcomplicating Lambda Expressions
One of the major benefits of using functional interfaces is the ability to write clean and concise code using lambda expressions. However, it's easy to overcomplicate these expressions, leading to code that's difficult to read or maintain.
Poor Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> {
if (name.startsWith("A")) {
System.out.println(name.toUpperCase());
} else {
System.out.println(name);
}
});
Better Approach:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name.startsWith("A") ? name.toUpperCase() : name));
Why This Matters
Clean code is key to maintainability, and excessive logic in lambdas defeats the purpose. Aim for keeping your lambda expressions as simple as possible. If a lambda becomes complex, consider extracting it into a separate method.
4. Ignoring Exceptional Handling
Lambdas can obscure exception handling. If your functional interface's method throws a checked exception, handling that exception becomes tricky. Java's functional interfaces don't declare that they throw checked exceptions.
Example of the Pitfall:
@FunctionalInterface
public interface ExceptionThrowingInterface {
void performAction() throws IOException;
}
You cannot directly use this functional interface with lambda:
ExceptionThrowingInterface action = () -> { throw new IOException(); }; // Compilation error
Why This Matters
If you know that exceptions might be thrown, you should define a new unchecked exception or wrap checked exceptions in a runtime exception to avoid compilation issues.
Solution:
public interface SafeAction {
void perform() throws RuntimeException;
}
SafeAction safeAction = () -> {
try {
throw new IOException();
} catch (IOException e) {
throw new RuntimeException(e);
}
};
5. Not Understanding Scope and this
When using this
in a lambda, it refers to the instance of the enclosing class, not anything related to the lambda itself. This behavior can often lead to confusion.
Example:
class MyClass {
private String name = "Outer";
public void method() {
Runnable r = () -> System.out.println(this.name); // This refers to MyClass' name
r.run();
}
}
Why This Matters
Make sure you distinguish between the outer class's context and the lambda's context. This understanding helps prevent malfunctioning code due to misplaced or misunderstood references.
6. Performance Considerations
Lambdas do have a performance cost, particularly when used in tight loops. Each lambda reference can lead to creation of a new object, which isn't always optimal.
Example Pitfall:
List<String> list = Arrays.asList("One", "Two", "Three");
for (String s : list) {
Runnable r = () -> System.out.println(s);
r.run(); // Creates a new Runnable for each iteration
}
Better Approach:
for (String s : list) {
// Directly reference s without recreating
System.out.println(s);
}
Why This Matters
Understanding how your code translates into performance can be crucial, especially in applications where efficiency is paramount. Be mindful of how you structure your lambdas, particularly in performance-sensitive situations.
Key Takeaways
While functional interfaces in Java 8 offer powerful new features, it's essential to navigate them with caution. Recognizing and avoiding these common pitfalls will help you write more effective, maintainable, and robust Java applications. As you immerse yourself in functional programming, keep these best practices close at hand to guide you on your journey.
For more on Java 8 features, consider checking out the Java 8 Tutorial or dive deeper into functional programming concepts.
By being vigilant and proactive, you can harness the full potential of functional interfaces and elevate your Java programming skills to new heights. Happy coding!